Use Void Functions in C++
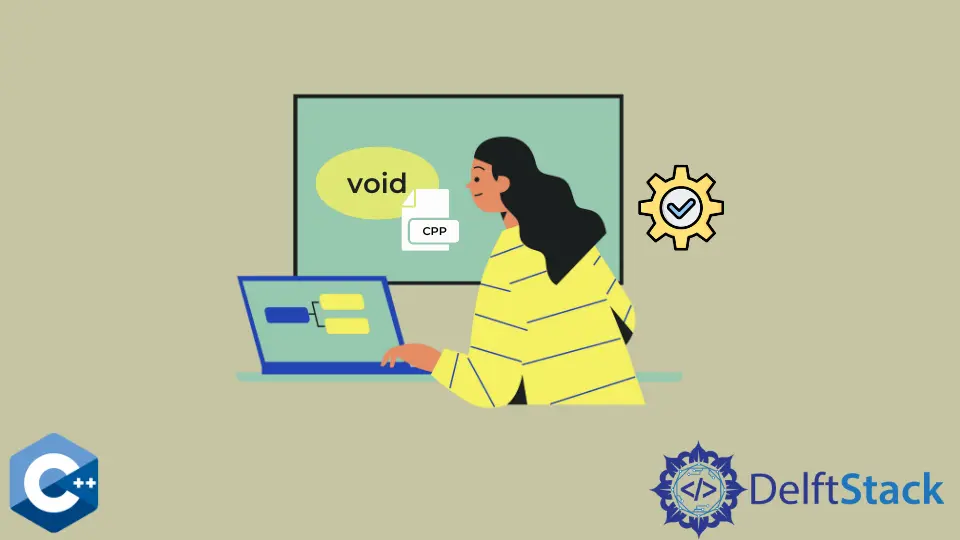
This article will demonstrate multiple methods about how to use void
functions in C++.
The article delves into the significance of void
functions in C++, covering their importance and usage in three distinct methods—no parameters, with value parameters, and reference parameters—illustrated through practical examples, including comparing string lengths, checking map keys, and sorting vector elements, all consolidated within a single C++ file.
Importance of void
Functions in C++
A void
function in C++ is a function that does not return a value. The void
keyword in the function signature indicates that the function does not produce any output.
Instead, its purpose is to execute a sequence of statements, perform tasks, or modify program state without the need to provide a result.
Key Points | Description |
---|---|
Task Execution | void functions are used to perform actions or tasks without the need to produce a return value. This is useful for procedures that contribute to a program’s functionality. |
Modular Design | void functions enhance code modularity by encapsulating specific tasks or operations. This approach improves code readability, maintainability, and understanding. |
Code Reusability | Isolating functionality within void functions promotes code reusability. Functions can be called multiple times, improving efficiency and reducing redundancy. |
Readability and Maintainability | void functions contribute to cleaner and more readable code. Organizing tasks into separate functions makes the codebase easier to navigate and maintain. Useful in larger projects. |
In C++, functions are fundamental building blocks that enable code organization and reuse. void
functions play a crucial role in situations where the execution of a task is the primary goal, and there’s no need to return a value.
void
Functions in C++
We’ll explore the versatility of void
functions by diving into three distinct methods of implementation: the no parameters
method, the with value parameters
method, and the with reference parameters
method. Understanding these variations enhances your ability to design modular and reusable code.
No Parameters Method
Using void
functions with no parameters in C++ is crucial for tasks that don’t require external input. This method simplifies code, emphasizing clarity and efficiency.
Without parameters, it excels in readability, making it ideal for concise operations where simplicity and directness are paramount.
#include <iostream>
// Void function with no parameters
void greetUser() {
std::cout << "Welcome! You are now inside the void." << std::endl;
}
int main() {
// Function call
greetUser();
// Other code can be added here...
return 0;
}
In this example, we define a void
function named greetUser
that takes no parameters.
The function simply prints a welcome message to the console. In the main
function, we call greetUser
, showcasing the simplicity of a void
function without parameters.
The output, when executed, will be:
The void
function with no parameters successfully executes the task. This concise method enhances code simplicity and readability for actions that require no external input.
Value Parameters Method
void
functions with value parameters in C++ provide flexibility by accepting input for specific tasks. This method allows dynamic customization, making code adaptable to various scenarios.
It enhances versatility, enabling functions to process different data sets without modifying their structure, contributing to more reusable and modular code.
#include <iostream>
// Void function with value parameters
void multiplyAndDisplay(int a, int b) {
int result = a * b;
std::cout << "Result of multiplication: " << result << std::endl;
}
int main() {
// Function call with arguments
multiplyAndDisplay(5, 7);
// Other code can be added here...
return 0;
}
In this example, we define a void
function named multiplyAndDisplay
that takes two integer parameters. Inside the function, we perform multiplication and display the result.
The main
function calls multiplyAndDisplay
with the arguments 5 and 7. This illustrates how void
functions can accept values, perform operations, and produce side effects without returning a value.
The output, when executed, will be:
The void
function with value parameters effectively multiplies provided values. This approach showcases the flexibility of customizing functions to process specific data sets, enhancing code adaptability and reusability.
With Reference Parameters Method
void
functions with reference parameters in C++ efficiently modify external variables, offering a memory-saving alternative. This method avoids unnecessary data duplication, making it resource-friendly and suitable for functions requiring direct manipulation of existing values.
It enhances performance and is particularly beneficial for large datasets or resource-intensive operations.
#include <iostream>
// Void function with reference parameters
void swapValues(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
// Before swapping
std::cout << "Before swapping - x: " << x << ", y: " << y << std::endl;
// Function call with reference parameters
swapValues(x, y);
// After swapping
std::cout << "After swapping - x: " << x << ", y: " << y << std::endl;
return 0;
}
In this example, we define a void
function named swapValues
that takes two integer reference parameters. This function swaps the values of the provided references.
In the main
function, we declare two integers, x
and y
, and display their values before and after calling swapValues
. This demonstrates how void
functions with reference parameters can modify the values of variables outside their scope.
The output, when executed, will be:
The void
function with reference parameters efficiently swaps variable values. This method optimally modifies existing data, making it ideal for resource-intensive operations and enhancing overall program performance.
void
functions, with their ability to execute actions without returning values, are indispensable tools in C++ programming. By understanding and utilizing the no parameters
, with value parameters
, and with reference parameters
methods, you gain a deeper insight into the flexibility and power that void
functions offer.
Incorporating these techniques into your code allows for modular, readable, and efficient programming practices.
Power of void
Functions in C++
In the realm of C++ programming, functions serve as indispensable tools, allowing developers to modularize and streamline their code. Among these, void
functions play a vital role by executing tasks without the need to return a value.
We’ll explore the versatility of void
functions through practical examples. We’ll cover finding which string is longer, checking if a key exists in a map, and sorting elements in a vector, all within a single C++ file.
Code Example:
#include <algorithm>
#include <iostream>
#include <map>
#include <string>
#include <vector>
// Function to compare string lengths
void compareStrings(const std::string& str1, const std::string& str2);
// Function to check if key exists in a map
void checkKeyInMap(const std::map<int, std::string>& myMap, int key);
// Function to sort elements in a vector
void sortVector(std::vector<int>& myVector);
int main() {
// Example 1: Comparing strings
std::string firstString = "Hello";
std::string secondString = "Greetings";
compareStrings(firstString, secondString);
// Example 2: Checking key in map
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
checkKeyInMap(myMap, 2);
// Example 3: Sorting vector
std::vector<int> myVector = {5, 2, 8, 1, 6};
sortVector(myVector);
return 0;
}
Comparing Strings
The compareStrings
void
function takes two strings as input parameters, str1
and str2
. Using the length()
method, it compares the lengths of the strings.
If str1
is longer, it prints String 1 is longer
. If str2
is longer, it prints String 2 is longer
.
Otherwise, if both strings have equal length, it prints Both strings are of equal length
.
Checking Key in Map
In the checkKeyInMap
void
function, it checks if a specified key exists in a given map (myMap
). It utilizes the find
method to search for the key.
If found, it prints Key [key] exists in the map with value: [value]
. If not found, it prints Key [key] does not exist in the map
.
Sorting Vector
The sortVector
void
function sorts the elements in a vector (myVector
) in ascending order using the std::sort
algorithm. After sorting, it prints the sorted vector elements.
This function is effective for arranging vector elements in numerical order.
By incorporating these void
functions into a single C++ file, we’ve demonstrated their flexibility and efficiency in performing diverse tasks.
From comparing strings to checking keys in a map and sorting vectors, void
functions prove invaluable for enhancing code modularity and readability. The output of each example showcases the seamless execution of these operations, illustrating the power of void
functions in C++.
Conclusion
The article highlights the significance of void
functions in C++, showcasing their versatility in three distinct methods: no parameters, value parameters, and reference parameters. Through practical examples, the article demonstrates how void
functions streamline code by efficiently handling diverse tasks, such as comparing string lengths, checking map keys, and sorting vectors.
This unified approach emphasizes clarity, modularity, and readability, illustrating the essential role of void
functions in enhancing C++ programming efficiency.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook