C++ でボイド関数を使用する
胡金庫
2023年10月12日
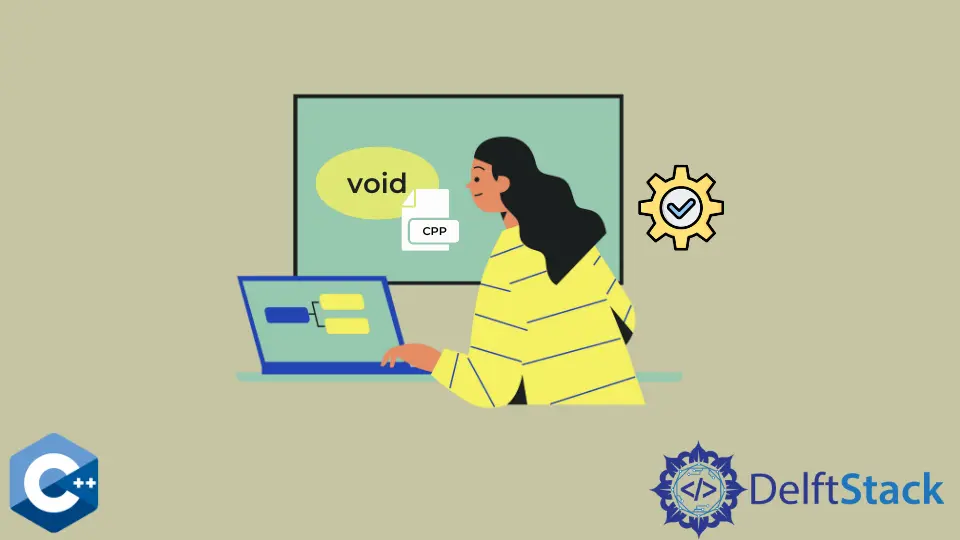
この記事では、C++ で void
関数を使用する方法に関する複数の方法を示します。
void
関数を使用して、どの文字列が長いかを見つける
戻り値のない関数には、戻りパラメーターとして指定された void
タイプがあります。void
関数では、暗黙の return
ステートメントは、関数本体の最後のステートメントの後に呼び出されます。明示的な return
ステートメントは、制御フローを呼び出し元の関数にすぐに移動する必要がある void
関数本体に配置できることに注意してください。次の例では、isLessString
関数に対応する文字列をコンソールに出力するための唯一の条件ステートメントが含まれており、その後に暗黙の return
が実行されます。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
using std::vector;
void isLessString(string &s1, string &s2) {
s1.size() < s2.size() ? cout << "string_1 is shorter than string_2" << endl
: cout << "string_2 is shorter than string_1" << endl;
}
int main() {
string str1 = "This string has arbitrary contents";
string str2 = "Another string with random contents";
isLessString(str1, str2);
return EXIT_SUCCESS;
}
出力:
string_1 is shorter than string_2
void
関数を使用して、キーがマップに存在するかどうかを確認する
この場合、void
関数を使用して、std::map
コンテナのキー検索関数を実装します。結果は、対応する文字列定数を cout
ストリームに出力することによって伝達されることに注意してください。これは、プログラムの内部でデータを渡すための推奨される方法ではありませんが、エンドユーザーに情報を示すために使用できます。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
using std::vector;
void keyExistsInMap(map<string, string>& m, const string& key) {
m.find(key) != m.end() ? cout << "Key exists in a map" << endl
: cout << "Key does not exist in a map" << endl;
}
int main() {
map<string, string> veggy_map = {{
"a",
"Ali",
},
{
"m",
"Malvo",
},
{
"p",
"Pontiac",
},
{
"s",
"Sensi",
}};
keyExistsInMap(veggy_map, "s");
keyExistsInMap(veggy_map, "x");
return EXIT_SUCCESS;
}
出力:
Key exists in a map
Key does not exist in a map
void
関数を使用して、ベクトル内の要素を並べ替える
または、vector
オブジェクトを参照として受け取り、その要素を昇順で並べ替える、std::sort
アルゴリズムのラッパー関数を実装することもできます。void
関数には通常、失敗を呼び出し元の関数に伝える手段がないため、ソリューションを設計する際には常に考慮に入れる必要があることに注意してください。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
using std::vector;
void sortVector(vector<string> &vec) {
std::sort(vec.begin(), vec.end(),
[](const auto &x, const auto &y) { return x < y; });
}
int main() {
vector<string> arr = {
"element", "implementation", "or", "the", "execution", "dependent",
"template", "character", "that", "all", "via", "class"};
sortVector(arr);
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
出力:
all; character; class; dependent; element; execution; implementation; or; template; that; the; via;
著者: 胡金庫