How to Create Vector of Vectors in C++
- Use Default Constructor to Create a Vector of Vectors in C++
-
Use the
rand
Function to Fill Vector of Vectors With Arbitrary Values in C++ - Use the Range Based Loop to Modify Each Element of Vector of Vectors in C++
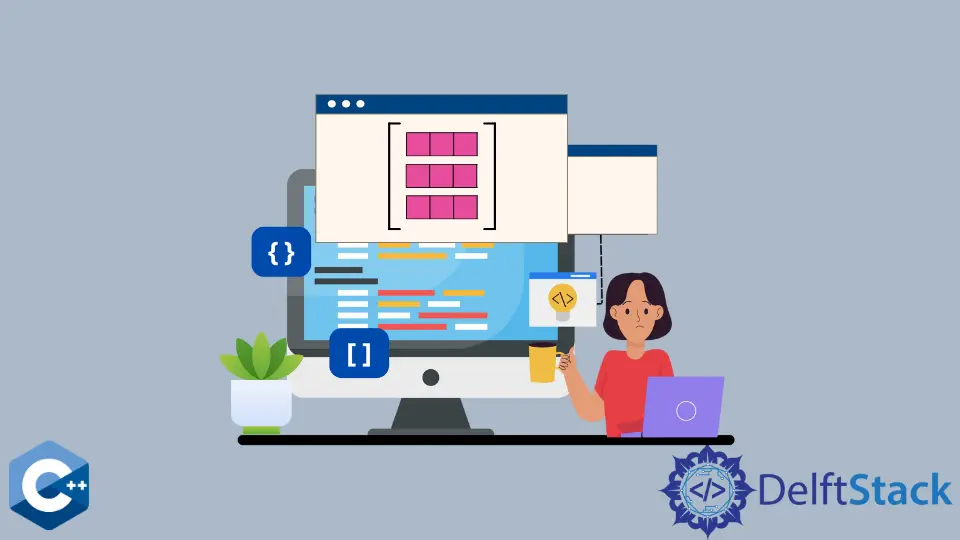
This article will explain how to create a vector of vectors in C++.
Use Default Constructor to Create a Vector of Vectors in C++
Since creating a vector of vectors means constructing a two-dimensional matrix, we will define LENGTH
and WIDTH
constants to specify as constructor parameters. Notation needed to declare an integer vector of vectors is vector<vector<int> >
(space after the first <
is for readability purposes only).
In the following example, we essentially declare a 4x6 dimensional matrix, elements of which can be accessed using [x][y]
notation and initialized using literal values. Note that we can also access the 2d vector’s elements by calling the at
method twice with given positions.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int LENGTH = 4;
constexpr int WIDTH = 6;
int main() {
vector<vector<int> > vector_2d(LENGTH, vector<int>(WIDTH, 0));
vector_2d[2][2] = 12;
cout << vector_2d[2][2] << endl;
vector_2d.at(3).at(3) = 99;
cout << vector_2d[3][3] << endl;
return EXIT_SUCCESS;
}
Output:
12
99
Use the rand
Function to Fill Vector of Vectors With Arbitrary Values in C++
A vector of vectors is often used in multiple linear algebra or graphics workflows. Thus, it’s common to have a two-dimensional vector initialized with random values. Initializing relatively larger 2D vectors using the initializer list can be cumbersome, so one should utilize loop iteration and the rand
function to generate arbitrary values.
Since this case does not entail any cryptographically sensitive operation, the rand
function seeded with the current time argument will generate sufficiently random values. We are generating a random number in the interval of [0, 100)
and at the same time outputting each element to the console.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int LENGTH = 4;
constexpr int WIDTH = 6;
int main() {
vector<vector<int> > vector_2d(LENGTH, vector<int>(WIDTH, 0));
std::srand(std::time(nullptr));
for (auto &item : vector_2d) {
for (auto &i : item) {
i = rand() % 100;
cout << setw(2) << i << "; ";
}
cout << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
83; 86; 77; 15; 93; 35;
86; 92; 49; 21; 62; 27;
90; 59; 63; 26; 40; 26;
72; 36; 11; 68; 67; 29;
Use the Range Based Loop to Modify Each Element of Vector of Vectors in C++
Generally, declaring two-dimensional matrices using std::vector
as shown in the previous examples can be quite inefficient and compute-heavy for latency-critical applications. Time-sensitive applications usually declare matrices using old school C-style [][]
notation. On the plus side, the std::vector
matrix can be iterated with a range-based loop, as demonstrated in the following example.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int LENGTH = 4;
constexpr int WIDTH = 6;
int main() {
vector<vector<int> > vector_2d(LENGTH, vector<int>(WIDTH, 0));
for (auto &item : vector_2d) {
for (auto &i : item) {
i = rand() % 100;
cout << setw(2) << i << "; ";
}
cout << endl;
}
cout << endl;
// Multiply Each Element By 3
for (auto &item : vector_2d) {
for (auto &i : item) {
i *= 3;
}
}
for (auto &item : vector_2d) {
for (auto &i : item) {
cout << setw(2) << i << "; ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Output:
83; 86; 77; 15; 93; 35;
86; 92; 49; 21; 62; 27;
90; 59; 63; 26; 40; 26;
72; 36; 11; 68; 67; 29;
249; 258; 231; 45; 279; 105;
258; 276; 147; 63; 186; 81;
270; 177; 189; 78; 120; 78;
216; 108; 33; 204; 201; 87
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook