How to Deallocate a std::vector Object in C++
- Understanding std::vector Memory Management
- Method 1: Automatic Deallocation
- Method 2: Clearing the Vector
-
Method 3: Using
shrink_to_fit()
- Method 4: Manual Deallocation with Dynamic Allocation
- Conclusion
- FAQ
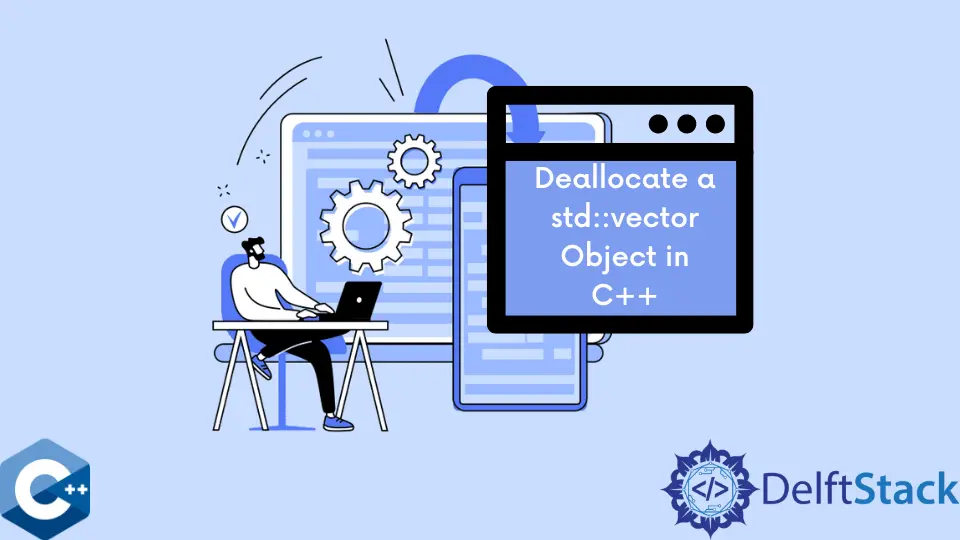
In C++, managing memory is a crucial aspect of programming. One of the most commonly used data structures is the std::vector
, which provides dynamic array capabilities. However, with great power comes great responsibility, especially when it comes to memory management. Knowing how to deallocate a std::vector
object properly is essential to prevent memory leaks and ensure efficient resource usage.
In this article, we will explore various methods to deallocate a std::vector
object in C++. We will discuss both self-managed methods and dynamic approaches that can help you handle memory more effectively. By the end of this guide, you will have a solid understanding of how to manage std::vector
objects in your C++ applications.
Understanding std::vector Memory Management
Before diving into deallocation methods, it’s important to understand how std::vector
manages memory. A std::vector
allocates memory dynamically and can grow or shrink as needed. When a vector goes out of scope, its destructor is called, automatically deallocating the memory it uses. However, there are scenarios where you might want to manually control this process, especially when dealing with large datasets or performance-critical applications.
Method 1: Automatic Deallocation
The simplest way to deallocate a std::vector
is to let C++ handle it automatically. When a std::vector
goes out of scope, its destructor is invoked, which releases the memory it occupies. This method is straightforward and requires no additional code.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Vector is automatically deallocated when it goes out of scope
return 0;
}
When you define a std::vector
within a function, as shown above, it is automatically cleaned up when the function ends. This means you don’t have to worry about explicitly deallocating the memory, making your code cleaner and less error-prone. However, keep in mind that if you use pointers or dynamic memory allocation within the vector, you will need to manage that memory separately.
Method 2: Clearing the Vector
Another method to deallocate elements from a std::vector
is to use the clear()
function. This method removes all elements from the vector, effectively deallocating the memory used by these elements, but the vector itself remains allocated.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.clear();
std::cout << "Vector cleared, size: " << vec.size() << std::endl;
return 0;
}
Output:
Vector cleared, size: 0
The clear()
method removes all elements from the vector but does not free the memory allocated for the vector itself. This can be useful if you plan to reuse the vector later, as it allows you to maintain the vector’s capacity while freeing up the space occupied by its elements. It’s a good practice to use this method when you need to reset the vector without destroying it.
Method 3: Using shrink_to_fit()
If you want to reduce the capacity of a std::vector
to match its size after clearing it, you can use the shrink_to_fit()
method. This method can help in optimizing memory usage by deallocating any excess capacity.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.clear();
vec.shrink_to_fit();
std::cout << "Vector size after shrinking: " << vec.size() << std::endl;
return 0;
}
Output:
Vector size after shrinking: 0
Using shrink_to_fit()
after clearing the vector is an excellent way to ensure that you are not holding onto unnecessary memory. It reduces the capacity of the vector to fit its size, which can lead to better memory efficiency. However, it’s worth noting that this operation may involve additional overhead, so use it judiciously based on your application’s needs.
Method 4: Manual Deallocation with Dynamic Allocation
In certain scenarios, you might need to allocate a std::vector
dynamically using new
. In such cases, you must manually deallocate the vector to prevent memory leaks. Here’s how you can do that:
#include <iostream>
#include <vector>
int main() {
std::vector<int>* vec = new std::vector<int>({1, 2, 3, 4, 5});
delete vec; // Manual deallocation
return 0;
}
When you allocate a std::vector
dynamically, you must remember to delete it using the delete
operator. Failing to do so will result in a memory leak, as the memory allocated for the vector will not be reclaimed. This method provides you with more control over memory management but requires careful handling to avoid leaks and dangling pointers.
Conclusion
Deallocating a std::vector
object in C++ is a fundamental aspect of memory management that every developer should understand. Whether you choose to rely on automatic deallocation, clear the vector, shrink its capacity, or manually manage memory through dynamic allocation, each method has its own advantages. By mastering these techniques, you can write more efficient and robust C++ applications. Remember, effective memory management not only improves performance but also enhances the overall reliability of your software.
FAQ
-
What happens if I don’t deallocate a std::vector?
Not deallocating a std::vector can lead to memory leaks, which may cause your application to consume more memory than necessary. -
Is it safe to use clear() on a std::vector?
Yes, using clear() on a std::vector is safe and will remove all elements, but the vector itself will remain allocated. -
When should I use shrink_to_fit()?
You should use shrink_to_fit() when you want to reduce the capacity of a vector to match its size after clearing it, optimizing memory usage. -
Can I allocate a std::vector dynamically?
Yes, you can allocate a std::vector dynamically using the new operator, but you must also remember to delete it to prevent memory leaks. -
Does std::vector automatically manage memory?
Yes, std::vector automatically manages memory and deallocates it when it goes out of scope, making it easier for developers to handle memory.