Vector Iterator in C++
-
Use
begin()
andend()
Methods to Iterate Over a Vector in C++ -
Use
advance()
Method to Iterate Over a Vector in C++ -
Use
next()
andprev()
Methods to Iterate Over a Vector in C++ -
Use
inserter()
Method to Iterate Over a Vector in C++
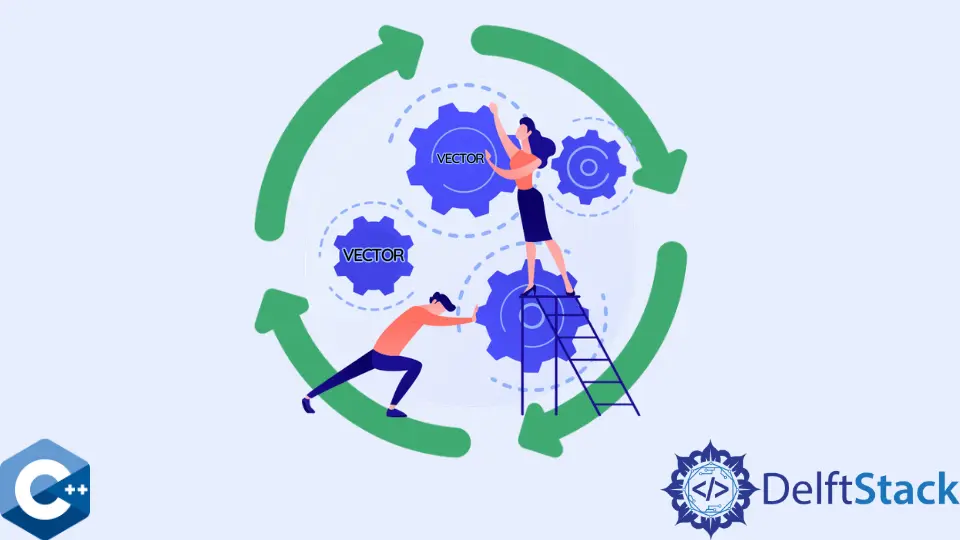
This tutorial demonstrates how to iterate over a vector in C++.
C++ provides many methods to iterate over vectors. These methods are called iterators, which point to the memory addresses of STL containers; this tutorial demonstrates different methods to iterate over the vectors in C++.
Use begin()
and end()
Methods to Iterate Over a Vector in C++
The begin()
method returns the beginning point of a container and the end()
returns the endpoint of the container. Both of these methods can be used after the iterator show or use the value of a vector.
See the example:
#include <iostream>
#include <iterator>
#include <vector>
using namespace std;
int main() {
vector<char> DemoVector = {'d', 'e', 'l', 'f', 't', 's', 't', 'a', 'c', 'k'};
// Declare the iterator to a vector
vector<char>::iterator ptr;
// use begin() and end() to dispaly the members of the vector
cout << "The vector values are : ";
for (ptr = DemoVector.begin(); ptr < DemoVector.end(); ptr++)
cout << *ptr << " ";
return 0;
}
The code above will display the vector values using the begin()
and end()
iterator methods. See the output:
The vector Values are : d e l f t s t a c k
Use advance()
Method to Iterate Over a Vector in C++
The advance()
method is used to increment the iterator position until a particular number is given in the argument. Let’s see how it works.
#include <iostream>
#include <iterator>
#include <vector>
using namespace std;
int main() {
vector<char> DemoVector = {'d', 'e', 'l', 'f', 't', 's', 't', 'a', 'c', 'k'};
// Declare the iterator to a vector
vector<char>::iterator ptr = DemoVector.begin();
// Use advance() to increment iterator position to 6th element
advance(ptr, 5);
cout << "The element after incrementing the iterator position is : ";
cout << *ptr << " ";
return 0;
}
The code above will use the advance
method to increment the position of the iterator to the sixth element s
. See the output:
The element after incrementing the iterator position is : s
Use next()
and prev()
Methods to Iterate Over a Vector in C++
The next()
method is used to return a new iterator, and that iterator will point after advancing the positions mentioned in its arguments. Similarly, the prev()
method is used to return a new iterator, and that iterator will point after decrementing the positions mentioned in its arguments.
Let’s try to iterate over a vector using the next
and prev
methods.
#include <iostream>
#include <iterator>
#include <vector>
using namespace std;
int main() {
vector<char> DemoVector = {'d', 'e', 'l', 'f', 't', 's', 't', 'a', 'c', 'k'};
// Declaring iterators to a vector
vector<char>::iterator ptr = DemoVector.begin();
vector<char>::iterator ftr = DemoVector.end();
// Use next() method to return new iterator points to the 5th element
auto DemoIterator1 = next(ptr, 4);
// Use prev() method to return new iterator points to 4th element
auto DemoIterator2 = prev(ftr, 4);
// Display next iterator position
cout << "Element of new iterator using next() is : ";
cout << *DemoIterator1 << " ";
cout << endl;
// Display prev iterator position
cout << "Element of new iterator using prev() is : ";
cout << *DemoIterator2 << " ";
cout << endl;
return 0;
}
The code above iterates over the vector using the next()
and prev()
methods. See the output:
Element of new iterator using next() is : t
Element of new iterator using prev() is : t
Use inserter()
Method to Iterate Over a Vector in C++
This method inserts elements into the vector while iterating over it. This method takes two parameters, the first is the container, and the other is the position where the element will be inserted.
Now let’s try an example to insert elements to a vector while iterating.
#include <iostream>
#include <iterator>
#include <vector>
using namespace std;
int main() {
vector<char> DemoVector1 = {'d', 'e', 'l', 'f', 't', 's', 't', 'a', 'c', 'k'};
vector<char> DemoVector2 = {'.', 'c', 'o', 'm'};
// Declare iterator to a vector
vector<char>::iterator ptr = DemoVector1.begin();
// Use advance to set the position
advance(ptr, 10);
// copy 1 vector elements into other using inserter()
copy(DemoVector2.begin(), DemoVector2.end(), inserter(DemoVector1, ptr));
// Display the new vector elements
cout << "The new vector after insertion of elements is : ";
for (char &a : DemoVector1) cout << a << " ";
return 0;
}
The code above will insert the element of one vector into another at a particular position while iterating. See the output:
The new vector after insertion of elements is : d e l f t s t a c k . c o m
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook