Multidimensional Vectors in C++
- Understanding Multidimensional Vectors
- Initializing and Accessing Elements
- Resizing and Modifying Vectors
- Conclusion
- FAQ
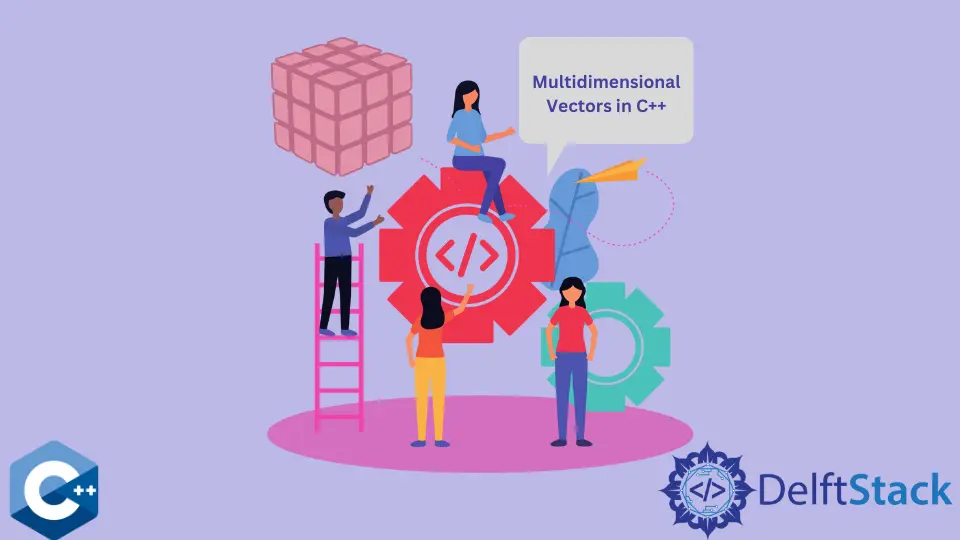
When it comes to programming in C++, one of the most useful data structures is the multidimensional vector. These vectors allow you to store data in a more organized way, making it easier to manage complex datasets. Whether you’re working on a game, a simulation, or any application that requires matrix-like structures, understanding how to declare and use multidimensional vectors is crucial.
In this tutorial, we will explore how to create and manipulate multidimensional vectors in C++, providing clear examples and explanations along the way. By the end, you’ll have a solid grasp of this powerful feature, ready to implement it in your projects.
Understanding Multidimensional Vectors
In C++, a multidimensional vector is essentially a vector of vectors. This allows you to create a matrix or a grid-like structure to hold your data. For instance, a two-dimensional vector can be thought of as a table with rows and columns. The syntax for declaring a multidimensional vector is straightforward but can be a bit confusing at first.
Here’s how you can declare a two-dimensional vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix(3, std::vector<int>(4, 0));
return 0;
}
In this example, we declare a two-dimensional vector named matrix
with 3 rows and 4 columns, initializing all elements to zero. The outer vector holds three inner vectors, each containing four integers.
The outer vector represents the rows, while each inner vector represents the columns. This structure is very helpful when you need to represent data in a tabular format, such as grids, game boards, or even mathematical matrices.
Initializing and Accessing Elements
Once you have declared your multidimensional vector, the next step is to initialize it with actual data and access its elements. You can do this using nested loops, which allow you to traverse through each element of the vector.
Here’s an example of initializing and accessing elements in a two-dimensional vector:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix(3, std::vector<int>(4, 0));
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 4; ++j) {
matrix[i][j] = i * j;
}
}
for (const auto& row : matrix) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
In this code snippet, we first initialize the matrix
with the product of its indices. Then, we use a nested loop to print out each element. The outer loop iterates through the rows, while the inner loop goes through the columns.
Output:
0 0 0 0
0 1 2 3
0 2 4 6
This output shows the initialized values of the matrix, where each element is the product of its row and column indices. This method of initialization is very flexible and can be adapted to fit various data needs.
Resizing and Modifying Vectors
One of the advantages of using vectors in C++ is their dynamic nature. You can resize a multidimensional vector at any point, allowing you to adapt to changing data requirements. Resizing can be particularly useful if you’re unsure of the size of your data at compile time.
Here’s how to resize and modify a multidimensional vector:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix;
matrix.resize(2, std::vector<int>(2, 1));
matrix.resize(3);
for (int i = 0; i < matrix.size(); ++i) {
matrix[i].resize(3, i + 1);
}
for (const auto& row : matrix) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
In this example, we start with an empty matrix
and resize it to have 2 rows and 2 columns, initializing all values to 1. Then, we resize it again to have 3 rows, adjusting the column size based on the row index.
Output:
1 1 1
2 2 2
3 3 3
The output shows how the vector has been resized and modified. This flexibility makes multidimensional vectors a powerful tool in C++, enabling you to handle data that may change during the program’s execution.
Conclusion
Multidimensional vectors in C++ are an essential feature for programmers looking to manage complex datasets efficiently. They allow for the storage of data in a structured manner, making it easier to perform operations on matrices or grids. Throughout this article, we’ve explored how to declare, initialize, access, and modify multidimensional vectors with clear examples. Whether you’re developing a game, a simulation, or any application that requires organized data, mastering multidimensional vectors will enhance your programming skills and efficiency.
FAQ
-
What is a multidimensional vector in C++?
A multidimensional vector in C++ is a vector of vectors, allowing you to create structures like matrices or grids. -
How do I declare a two-dimensional vector?
You can declare it using the syntaxstd::vector<std::vector<int>> matrix(rows, std::vector<int>(columns, initial_value));
. -
Can I resize a multidimensional vector?
Yes, you can resize a multidimensional vector using theresize()
method, which allows for dynamic changes in size. -
How do I access elements in a multidimensional vector?
You can access elements using the syntaxmatrix[row_index][column_index];
. -
What are the benefits of using multidimensional vectors?
They provide flexibility and dynamic sizing, making them suitable for various applications that require organized data storage.