How to Resize 2D Vector C++
- Understanding 2D Vectors in C++
-
Resizing a 2D Vector with
resize()
- Dynamically Resizing a 2D Vector
- Adding and Removing Rows and Columns
- Conclusion
- FAQ
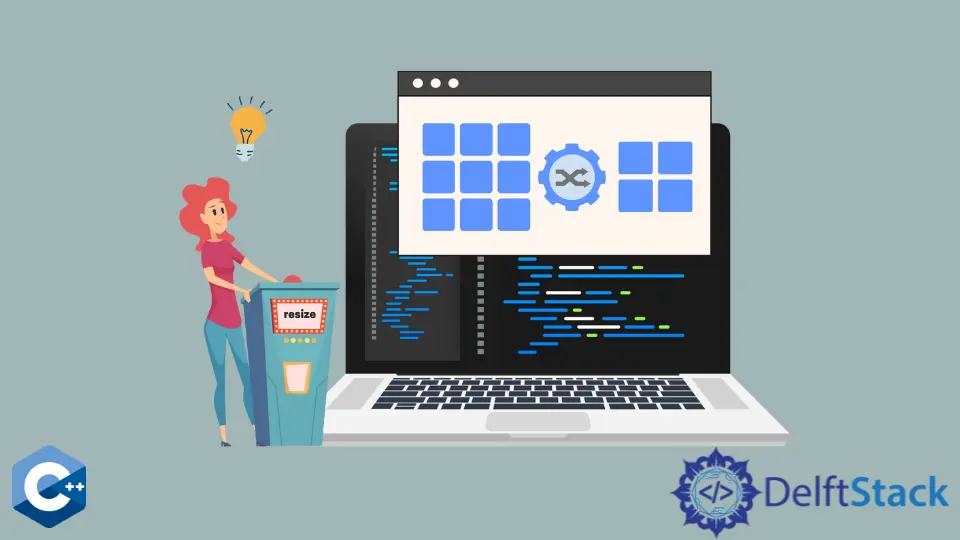
Resizing a 2D vector in C++ can seem daunting at first, especially for those new to the language or the Standard Template Library (STL). However, it’s a straightforward process once you get the hang of it. The primary function used for this task is resize()
, which allows you to change the size of your 2D vector dynamically.
In this article, we will explore how to effectively resize a 2D vector in C++, providing you with practical examples and clear explanations. Whether you’re working on a complex project or just learning the ropes, understanding how to manipulate 2D vectors is essential for your programming toolkit. Let’s dive in and unravel the intricacies of resizing 2D vectors in C++!
Understanding 2D Vectors in C++
Before we jump into resizing, let’s clarify what a 2D vector is. In C++, a 2D vector is essentially a vector of vectors. It allows you to create a grid-like structure, where each element can be accessed using two indices. For example, you can think of it as a matrix where elements are stored in rows and columns.
To declare a 2D vector, you typically do something like this:
#include <vector>
std::vector<std::vector<int>> vec;
This creates an empty 2D vector of integers. However, the real power of vectors comes when you start resizing them. The resize()
function is your go-to tool for this purpose. It allows you to adjust the dimensions of your 2D vector as needed.
Resizing a 2D Vector with resize()
The resize()
function is a member function of the vector class in C++. It can be used to change the size of the vector, and when it comes to a 2D vector, you can specify both dimensions. Here’s how you can do it:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> vec;
vec.resize(3, std::vector<int>(4, 0));
for (const auto& row : vec) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Output:
0 0 0 0
0 0 0 0
0 0 0 0
In this example, we first declare a 2D vector named vec
. We then use resize(3, std::vector<int>(4, 0))
to change its size. This means we want 3 rows, each containing 4 columns initialized to 0. The nested vector initialization is crucial here, as it ensures that each row has the correct number of elements.
When we print the vector, you can see that it successfully creates a 3x4 grid filled with zeros. This method is efficient and straightforward, making it a popular choice among C++ developers.
Dynamically Resizing a 2D Vector
Sometimes, you may not know the dimensions of your 2D vector upfront. In such cases, you can dynamically resize the vector based on user input or other conditions. Here’s a simple example:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> vec;
int rows, cols;
std::cout << "Enter number of rows: ";
std::cin >> rows;
std::cout << "Enter number of columns: ";
std::cin >> cols;
vec.resize(rows, std::vector<int>(cols));
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
vec[i][j] = i * j; // Example initialization
}
}
for (const auto& row : vec) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Output:
0 0 0
0 1 2
0 2 4
In this code, we first prompt the user to enter the number of rows and columns. After receiving the input, we call resize(rows, std::vector<int>(cols))
to create a 2D vector of the specified dimensions. We then initialize each element using a simple multiplication operation.
This approach allows you to create a flexible 2D vector whose size can be adjusted at runtime, a feature that is particularly useful in many applications.
Adding and Removing Rows and Columns
Resizing isn’t just about increasing the size of a vector; it’s also about managing its dimensions effectively. You might want to add or remove rows and columns as you process data. Here’s how you can do that:
Adding a Row
To add a new row to a 2D vector, you can use the push_back()
method:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> vec = {{1, 2}, {3, 4}};
vec.push_back({5, 6});
for (const auto& row : vec) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Output:
1 2
3 4
5 6
In this snippet, we start with a 2D vector containing two rows. By calling push_back({5, 6})
, we add a new row at the end of the vector. The output confirms that our vector now contains three rows.
Removing a Row
To remove a row, you can use the pop_back()
method:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> vec = {{1, 2}, {3, 4}, {5, 6}};
vec.pop_back();
for (const auto& row : vec) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Output:
1 2
3 4
In this example, we remove the last row by calling pop_back()
. After executing this code, the vector contains only the first two rows, demonstrating how easy it is to manage the size of a 2D vector.
Conclusion
Resizing a 2D vector in C++ is an essential skill that can greatly enhance your programming capabilities. With the resize()
function, you can create dynamic arrays that can adapt to your needs, whether you’re initializing a grid, adding new rows, or removing existing ones. By mastering these techniques, you’ll be better equipped to handle complex data structures in your projects. Remember, practice makes perfect, so don’t hesitate to experiment with different sizes and operations on your 2D vectors!
FAQ
-
What is a 2D vector in C++?
A 2D vector is a vector of vectors that allows you to create a grid-like structure for storing data in rows and columns. -
How do I initialize a 2D vector in C++?
You can initialize a 2D vector using the syntaxstd::vector<std::vector<int>> vec(rows, std::vector<int>(cols, initial_value));
. -
Can I resize a 2D vector dynamically?
Yes, you can use theresize()
function to change the dimensions of a 2D vector at runtime. -
How do I add or remove rows from a 2D vector?
You can usepush_back()
to add a new row andpop_back()
to remove the last row in a 2D vector. -
What happens to the elements when I resize a 2D vector?
When you resize a 2D vector, the new elements are initialized with the value you specify, while existing elements are preserved if the new size is larger.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook