Vector Container in C++ STL
-
Use
std::vector
to Construct a Dynamic Arrays in C++ -
Use
std::vector
to Storestruct
Objects in C++ -
Use
std::vector::swap
Function to Swap Vector Elements in C++
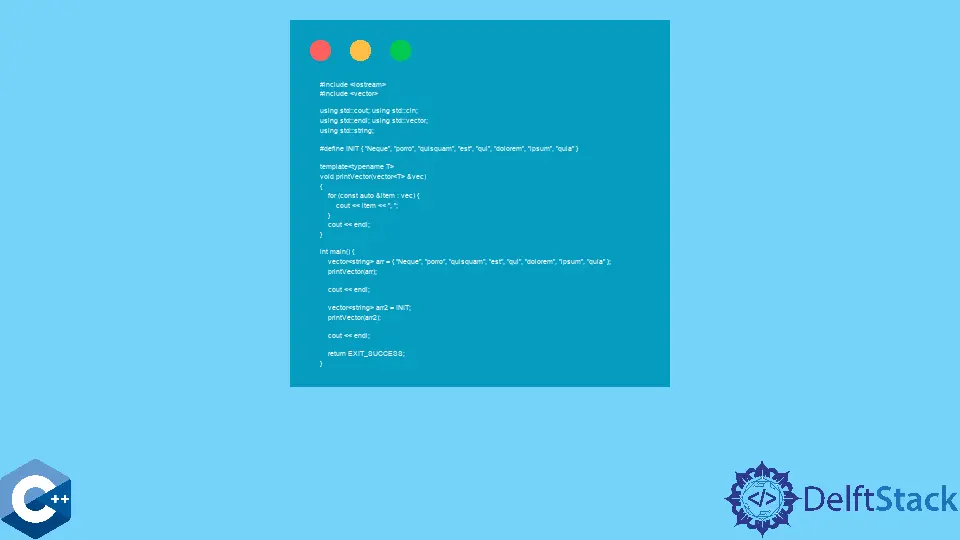
This article will introduce multiple methods about how to use a vector
container in C++.
Use std::vector
to Construct a Dynamic Arrays in C++
std::vector
is part of the containers library, and it implements a dynamically resizable array that stores elements in a contiguous storage. vector
elements are usually added at the end with push_back
or emplace_back
built-in functions. vector
container can be easily constructed with the initializer list as shown in the following example code - arr
object. Also, one can store static initializer list values in the macro expression and concisely express the initialization operation when needed.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
#define INIT \
{ "Neque", "porro", "quisquam", "est", "qui", "dolorem", "ipsum", "quia" }
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<string> arr = {"Neque", "porro", "quisquam", "est",
"qui", "dolorem", "ipsum", "quia"};
printVector(arr);
cout << endl;
vector<string> arr2 = INIT;
printVector(arr2);
cout << endl;
return EXIT_SUCCESS;
}
Use std::vector
to Store struct
Objects in C++
std::vector
can be utilized to store custom-defined structures and initialize them with the braced list notation similar to the previous example. Note that, when adding a new struct
element using the push_back
function, the value should be passed as a braced list, and if there are multiple nested structures within a struct
, the corresponding notation should be used.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct cpu {
string wine;
string country;
int year;
} typedef cpu;
int main() {
vector<cpu> arr3 = {{"Chardonnay", "France", 2018}, {"Merlot", "USA", 2010}};
for (const auto &item : arr3) {
cout << item.wine << " - " << item.country << " - " << item.year << endl;
}
arr3.push_back({"Cabernet", "France", 2015});
for (const auto &item : arr3) {
cout << item.wine << " - " << item.country << " - " << item.year << endl;
}
return EXIT_SUCCESS;
}
Use std::vector::swap
Function to Swap Vector Elements in C++
std::vector
provides multiple built-in functions to operate on its elements, one of which is swap
. It can be called from the vector
object and take another vector
as an argument to swap their contents. Another useful function is size
, which retrieves the current element count in the given vector
object. Note though, there is a similar function called capacity
that returns the number of elements stored in the currently allocated buffer. The latter is sometimes allocated larger in size to avoid unessential calls to the operating system services. The following example code demonstrates the scenario where the two functions return different values.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr4 = {1, 12, 45, 134, 424};
vector<int> arr5 = {41, 32, 15, 14, 99};
printVector(arr4);
arr4.swap(arr5);
printVector(arr4);
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
for (int i = 0; i < 10000; ++i) {
arr4.push_back(i * 5);
}
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
arr4.shrink_to_fit();
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
return EXIT_SUCCESS;
}
Output:
1, 12, 45, 134, 424,
41, 32, 15, 14, 99,
arr4 capacity: 5 size: 5
arr4 capacity: 10240 size: 10005
arr4 capacity: 10005 size: 10005
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook