C++ STL의 벡터 컨테이너
-
std::vector
를 사용하여 C++에서 동적 배열 생성 -
std::vector
를 사용하여struct
객체를 C++로 저장 -
std::vector::swap
함수를 사용하여 C++에서 벡터 요소 교체
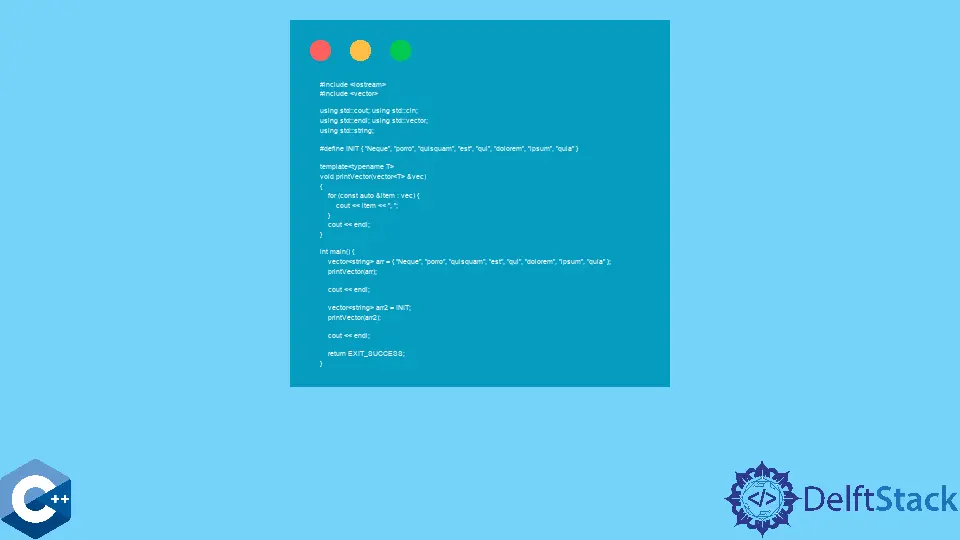
이 기사에서는 C++에서vector
컨테이너를 사용하는 방법에 대한 여러 방법을 소개합니다.
std::vector
를 사용하여 C++에서 동적 배열 생성
std::vector
는 컨테이너 라이브러리의 일부이며 연속 저장소에 요소를 저장하는 동적 크기 조정이 가능한 배열을 구현합니다. vector
요소는 일반적으로push_back
또는emplace_back
내장 함수와 함께 끝에 추가됩니다. vector
컨테이너는 다음 예제 코드 인arr
객체와 같이 이니셜 라이저 목록으로 쉽게 구성 할 수 있습니다. 또한 매크로 표현식에 정적 이니셜 라이저 목록 값을 저장하고 필요할 때 초기화 작업을 간결하게 표현할 수 있습니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
#define INIT \
{ "Neque", "porro", "quisquam", "est", "qui", "dolorem", "ipsum", "quia" }
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<string> arr = {"Neque", "porro", "quisquam", "est",
"qui", "dolorem", "ipsum", "quia"};
printVector(arr);
cout << endl;
vector<string> arr2 = INIT;
printVector(arr2);
cout << endl;
return EXIT_SUCCESS;
}
std::vector
를 사용하여struct
객체를 C++로 저장
std::vector
는 사용자 정의 구조를 저장하고 이전 예제와 유사한 중괄호 목록 표기법으로 초기화하는 데 사용할 수 있습니다. push_back
함수를 사용하여 새struct
요소를 추가 할 때 값은 중괄호 목록으로 전달되어야하며struct
내에 여러 중첩 구조가있는 경우 해당 표기법을 사용해야합니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct cpu {
string wine;
string country;
int year;
} typedef cpu;
int main() {
vector<cpu> arr3 = {{"Chardonnay", "France", 2018}, {"Merlot", "USA", 2010}};
for (const auto &item : arr3) {
cout << item.wine << " - " << item.country << " - " << item.year << endl;
}
arr3.push_back({"Cabernet", "France", 2015});
for (const auto &item : arr3) {
cout << item.wine << " - " << item.country << " - " << item.year << endl;
}
return EXIT_SUCCESS;
}
std::vector::swap
함수를 사용하여 C++에서 벡터 요소 교체
std::vector
는 요소에서 작동하는 여러 내장 함수를 제공하며, 그중 하나는 ‘스왑’입니다. vector
객체에서 호출 할 수 있으며 다른vector
를 인수로 사용하여 내용을 바꿀 수 있습니다. 또 다른 유용한 함수는 주어진vector
객체에서 현재 요소 수를 검색하는size
입니다. 하지만 현재 할당 된 버퍼에 저장된 요소의 수를 반환하는 capacity
라는 유사한 함수가 있습니다. 후자는 때때로 운영 체제 서비스에 대한 불필요한 호출을 피하기 위해 더 큰 크기로 할당됩니다. 다음 예제 코드는 두 함수가 서로 다른 값을 반환하는 시나리오를 보여줍니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr4 = {1, 12, 45, 134, 424};
vector<int> arr5 = {41, 32, 15, 14, 99};
printVector(arr4);
arr4.swap(arr5);
printVector(arr4);
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
for (int i = 0; i < 10000; ++i) {
arr4.push_back(i * 5);
}
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
arr4.shrink_to_fit();
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
return EXIT_SUCCESS;
}
출력:
1, 12, 45, 134, 424,
41, 32, 15, 14, 99,
arr4 capacity: 5 size: 5
arr4 capacity: 10240 size: 10005
arr4 capacity: 10005 size: 10005
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook