How to Convert String to Hex in C++
-
Use
std::cout
andstd::hex
to Convert String to Hexadecimal Value in C++ -
Use
std::stringstream
andstd::hex
to Convert String to Hexadecimal Value in C++
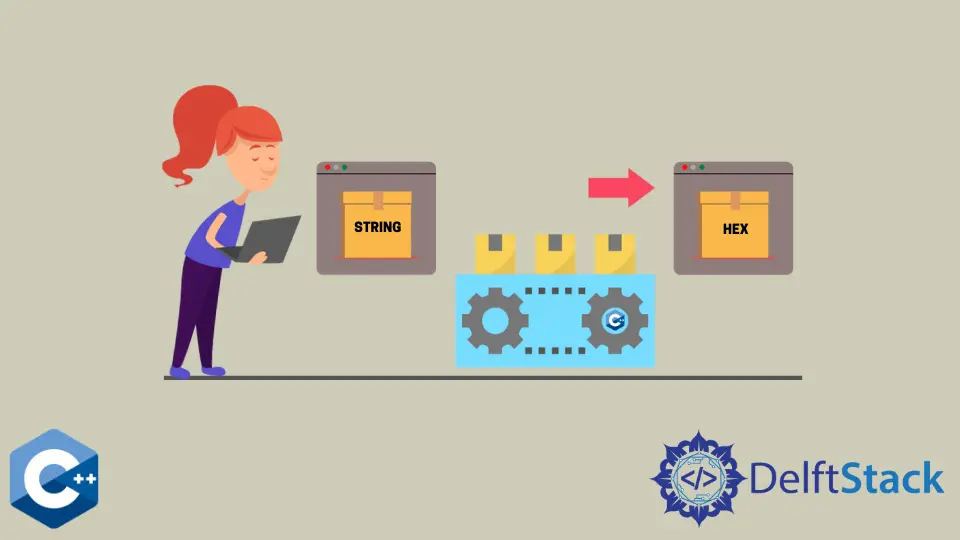
This article will demonstrate multiple methods about how to convert string to hex in C++.
Use std::cout
and std::hex
to Convert String to Hexadecimal Value in C++
Hexadecimal notation is a common format for reading binary files representing program files, encoded format, or just text. Thus, one would need to generate file contents with hexadecimal data and output it as needed.
In this example, we output the stored string
object as hexadecimal characters to the console. Note that C++ provides a std::hex
I/O manipulator that can modify the stream data’s number base. A string
object should be decomposed as single characters and then individually modified with std::hex
to their respective hexadecimal representation. We implement the range-based loop to iterate over string
characters and redirect modified data to the cout
stream.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
using std::cout;
using std::endl;
using std::hex;
using std::string;
using std::stringstream;
int main() {
string s1 = "This will be converted to hexadecimal";
string s2;
cout << "string: " << s1 << endl;
cout << "hexval: ";
for (const auto &item : s1) {
cout << hex << int(item);
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
string: This will be converted to hexadecimal
hexval: 546869732077696c6c20626520636f6e76657274656420746f2068657861646563696d616c
Use std::stringstream
and std::hex
to Convert String to Hexadecimal Value in C++
The previous method lacks the feature of storing the hexadecimal data in the object. The solution to this issue is to create a stringstream
object, where we insert the hexadecimal values of string
characters using the iteration. Once the data is in stringstream
, it can construct a new string
object for storing modified character data.
Notice that data can be outputted directly from the stringstream
object, but in the following example code, a more simple form - cout << string
is employed. An alternative end-use case could be to write the hexadecimal data directly into the file, using filesystem utilities of the standard library.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
using std::cout;
using std::endl;
using std::hex;
using std::string;
using std::stringstream;
int main() {
string s1 = "This will be converted to hexadecimal";
string s2;
stringstream ss;
cout << "string: " << s1 << endl;
for (const auto &item : s1) {
ss << hex << int(item);
}
s2 = ss.str();
cout << "hexval: " << s2 << endl;
return EXIT_SUCCESS;
}
Output:
string: This will be converted to hexadecimal
hexval: 546869732077696c6c20626520636f6e76657274656420746f2068657861646563696d616c
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++