C++에서 문자열을 16 진수로 변환
-
std::cout
및std::hex
를 사용하여 C++에서 문자열을 16 진수 값으로 변환 -
std::stringstream
및std::hex
를 사용하여 C++에서 문자열을 16 진수 값으로 변환
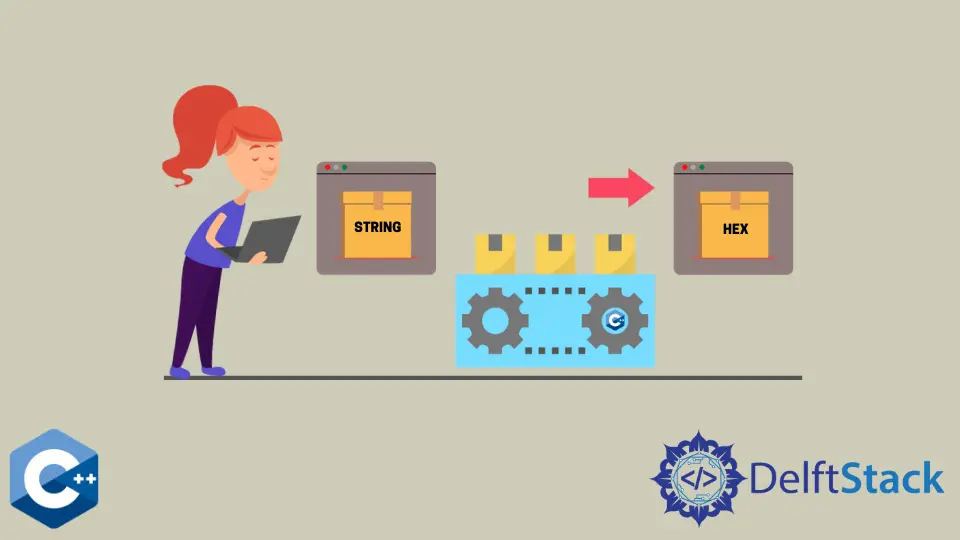
이 기사에서는 C++에서 문자열을 16 진수로 변환하는 방법에 대한 여러 방법을 보여줍니다.
std::cout
및std::hex
를 사용하여 C++에서 문자열을 16 진수 값으로 변환
16 진수 표기법은 프로그램 파일, 인코딩 된 형식 또는 텍스트 만 나타내는 이진 파일을 읽기위한 일반적인 형식입니다. 따라서 16 진수 데이터로 파일 내용을 생성하고 필요에 따라 출력해야합니다.
이 예에서는 저장된string
객체를 16 진수 문자로 콘솔에 출력합니다. C++는 스트림 데이터의 숫자 기반을 수정할 수있는std::hex
I/O
조작기를 제공합니다. string
객체는 단일 문자로 분해 한 다음std::hex
를 사용하여 각각의 16 진수 표현으로 개별적으로 수정해야합니다. 범위 기반 루프를 구현하여문자열문자를 반복하고 수정 된 데이터를cout
스트림으로 리디렉션합니다.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
using std::cout;
using std::endl;
using std::hex;
using std::string;
using std::stringstream;
int main() {
string s1 = "This will be converted to hexadecimal";
string s2;
cout << "string: " << s1 << endl;
cout << "hexval: ";
for (const auto &item : s1) {
cout << hex << int(item);
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
string: This will be converted to hexadecimal
hexval: 546869732077696c6c20626520636f6e76657274656420746f2068657861646563696d616c
std::stringstream
및std::hex
를 사용하여 C++에서 문자열을 16 진수 값으로 변환
이전 방법은 16 진수 데이터를 객체에 저장하는 기능이 없습니다. 이 문제에 대한 해결책은 반복을 사용하여string
문자의 16 진수 값을 삽입하는stringstream
객체를 만드는 것입니다. 데이터가stringstream
에 있으면 수정 된 문자 데이터를 저장하기위한 새로운string
객체를 생성 할 수 있습니다.
데이터는stringstream
객체에서 직접 출력 할 수 있지만 다음 예제 코드에서는 더 간단한 형식 인cout << string
이 사용됩니다. 다른 최종 사용 사례는 표준 라이브러리의 파일 시스템 유틸리티를 사용하여 16 진수 데이터를 파일에 직접 쓰는 것입니다.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
using std::cout;
using std::endl;
using std::hex;
using std::string;
using std::stringstream;
int main() {
string s1 = "This will be converted to hexadecimal";
string s2;
stringstream ss;
cout << "string: " << s1 << endl;
for (const auto &item : s1) {
ss << hex << int(item);
}
s2 = ss.str();
cout << "hexval: " << s2 << endl;
return EXIT_SUCCESS;
}
출력:
string: This will be converted to hexadecimal
hexval: 546869732077696c6c20626520636f6e76657274656420746f2068657861646563696d616c
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook