How to Create an Array of Strings in C++
-
Use the
std::vector
Container to Create an Array of Strings in C++ -
Use the
std::array
Container to Create an Array of Strings in C++ -
Use the
string arr[]
Notation to Create an Array of Strings in C++ -
Use the
char arr[][]
Notation to Create an Array of Strings in C++
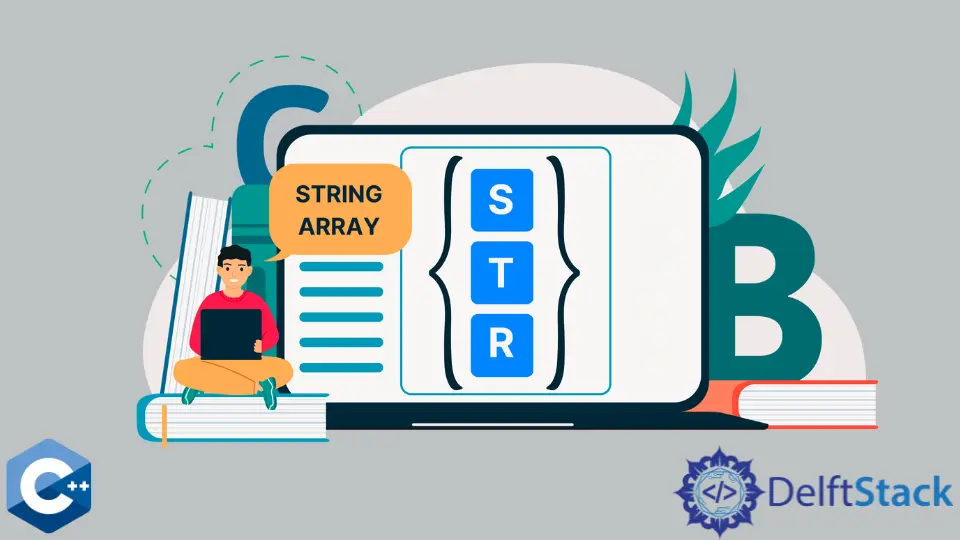
This tutorial will explain several methods of how to create an array of strings in C++.
Use the std::vector
Container to Create an Array of Strings in C++
The std::vector
container of the STL provides a dynamic array for generic data objects that can be utilized to create an array of strings. The elements in std::vector
are stored contiguously; thus, they can be accessed efficiently without overheads.
On the other hand, std::vector
usually occupies more storage than different types of static arrays because the former requires to allocate extra space for memory management efficiency. Additionally, std::vector
provides useful member functions for element manipulation like in-place element construction with the emplace_back
function, as demonstrated in the following example.
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
void printVector(std::vector<T> v) {
for (const auto &item : v) {
cout << item << "; ";
}
cout << endl;
}
int main() {
vector<string> str_arr1 = {"Sunny Cove", "Willow Cove", "Golden Cove",
"Ocean Cove"};
printVector(str_arr1);
str_arr1.emplace_back("Palm Cove");
printVector(str_arr1);
return EXIT_SUCCESS;
}
Output:
Sunny Cove; Willow Cove; Golden Cove; Ocean Cove;
Sunny Cove; Willow Cove; Golden Cove; Ocean Cove; Palm Cove;
Use the std::array
Container to Create an Array of Strings in C++
Alternatively, one can use the std::array
container to declare a static array. These arrays are similar to the C-style arrays in terms of memory footprint efficiency and provide the common member functions for accessibility. The std::array
container uses aggregate initialization. It can also be iterated using the common iterator functions and manipulated using the std::copy
algorithm, as shown in the following code sample.
#include <array>
#include <iostream>
#include <iterator>
#include <string>
using std::array;
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
int main() {
array<string, 4> str_arr2 = {"Sunny Cove", "Willow Cove", "Golden Cove",
"Ocean Cove"};
array str_arr3 = {"Sunny Cove", "Willow Cove", "Golden Cove", "Ocean Cove"};
std::copy(str_arr3.begin(), str_arr3.end(),
std::ostream_iterator<string>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Output:
Sunny Cove; Willow Cove; Golden Cove; Ocean Cove;
Use the string arr[]
Notation to Create an Array of Strings in C++
Another useful method to create an array of strings is the C-style array of string
objects; this would declare a fixed-element string array that can be randomly accessed with index notation and iterated with a range-based for
loop. Remember that you would need to implement custom functions for element manipulation and accessibility as included in the std::array
class.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str_arr4[4] = {"Sunny Cove", "Willow Cove", "Golden Cove",
"Ocean Cove"};
for (auto &i : str_arr4) {
cout << i << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
Sunny Cove; Willow Cove; Golden Cove; Ocean Cove;
Use the char arr[][]
Notation to Create an Array of Strings in C++
You can also allocate a string array that has a fixed length, with each string having the maximum number of characters predefined. Note that this method essentially declares a two-dimensional char
array that will store each string in a single row of the matrix. If the initializer list includes the string with more characters than the second dimension of the array, the last part of the string will be cropped to fit in the line.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
char str_arr5[4][20] = {"Sunny Cove", "Willow Cove", "Golden Cove",
"Ocean Cove"};
for (auto &i : str_arr5) {
cout << i << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
Sunny Cove; Willow Cove; Golden Cove; Ocean Cove;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook