Differences Between sizeof Operator and strlen Function for Strings in C++
-
the
sizeof
Operator Characteristics and Usage Scenarios -
Use the
std::string::size
Member Function to Retrieve the Number of Characters in Strings
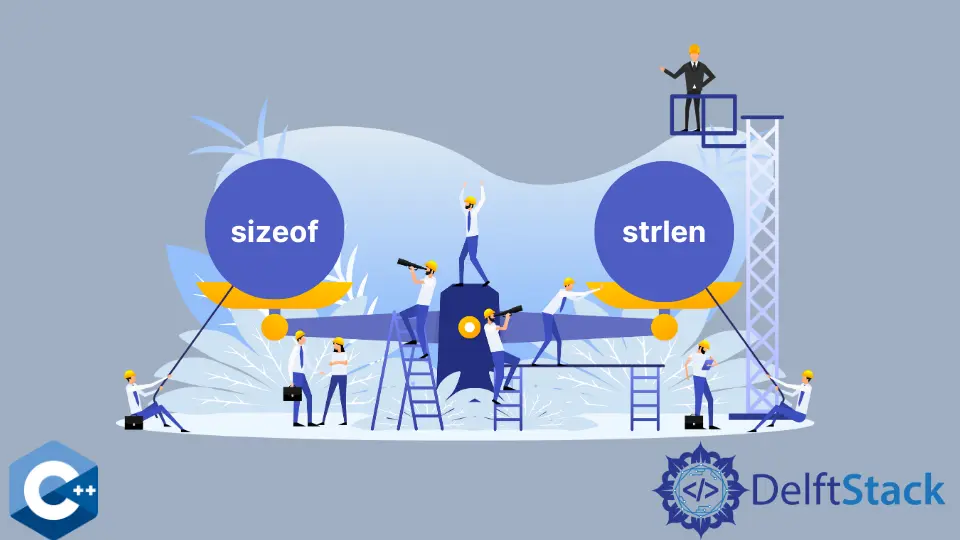
This article will demonstrate multiple differences when using the sizeof
operator as opposed to the strlen
function with strings in C++.
the sizeof
Operator Characteristics and Usage Scenarios
The sizeof
operator is a unary operator that retrieves the storage size for the given expression or data type. This operator evaluates the object size in byte units, and sizeof(char)
is guaranteed to be 1
.
There is a misguided notion that the 1 byte always equals 8 bits, and consequently, we can calculate the object’s size in bits. Actually, a byte is not guaranteed to be 8 bits by the language itself. It depends mainly on an underlying hardware platform. Still, most contemporary general-purpose hardware utilizes 8-bit bytes.
Mind that sizeof
operator can’t be applied to the expression that includes a function or an incomplete type or bitfields. In the following example code, we apply the sizeof
operator to two arrays of different types, and the results will likely be the same on your platform as well. Since the char
is guaranteed to have 1 byte size, 20 character array will take up 20 bytes.
On the other hand, the long long
data type is implementation-defined, and in this case, it happens to be 8 bytes. Hence the total array size of 160 bytes. Notice that we can also find the number of elements in the array by dividing the sizeof
whole array by the sizeof
one element, as shown on the last lines of the next example.
#include <iostream>
using std::cout;
using std::endl;
int main() {
char arr[20];
long long arr2[20];
cout << "sizeof ( array of 20 long longs ) : " << sizeof arr << endl;
cout << "sizeof ( array of 20 long longs ) : " << sizeof arr2 << endl;
cout << "length of array of 20 chars) : "
<< ((sizeof arr) / (sizeof arr[0])) << endl;
cout << "length of array of 20 long longs ): "
<< ((sizeof arr2) / (sizeof arr2[0])) << endl;
return EXIT_SUCCESS;
}
Output:
sizeof ( array of 20 long longs ) : 20
sizeof ( array of 20 long longs ) : 160
length of array of 20 chars) : 20
length of array of 20 long longs ): 20
Using the sizeof
operator to find the length of the string is wrong. Let’s consider two representation types of strings in C++, a character string and a std::string
class. The former one is mostly accessed using the char
pointer, and applying the sizeof
on it will retrieve the storage size of the pointer itself rather than the whole string.
If we try to retrieve the size of the std::string
object using the sizeof
operator, we get the object’s storage size, which will not be the number of characters in the string as demonstrated in the below code snippet.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "sizeof char* : " << sizeof text1 << endl;
cout << "sizeof string: " << sizeof text2 << endl;
cout << "sizeof string.data: " << sizeof text2.data() << endl;
return EXIT_SUCCESS;
}
Output:
sizeof char* : 8
sizeof string: 32
sizeof string.data: 8
Then we have got the strlen
function, which is the remnant of the C string library. It computes the length of the given character string, and the function returns the number of bytes as integral value. strlen
can be applied to char
pointer where a valid string is stored or to the value returned by std::string::c_str
, but it should not be the choice of the C++ programmer.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "strlen char* : " << strlen(text1) << endl;
cout << "strlen string: " << strlen(text2.c_str()) << endl;
return EXIT_SUCCESS;
}
Output:
strlen char* : 24
strlen string: 24
Use the std::string::size
Member Function to Retrieve the Number of Characters in Strings
On the contrary, the C++ programmer should utilize the size
member function of the std::string
class, as it offers a more safe method for handling strings. Notice that we can even use the size
member function if we have a character string. We need to construct a new string
object and directly invoke the size
function on the returned value. The following code example shows the primary usage for both scenarios.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "length char* : " << string(text1).size() << endl;
cout << "length string: " << text2.size() << endl;
return EXIT_SUCCESS;
}
Output:
length char* : 24
length string: 24
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++