C++의 문자열에 대한 sizeof 연산자와 strlen 함수의 차이점
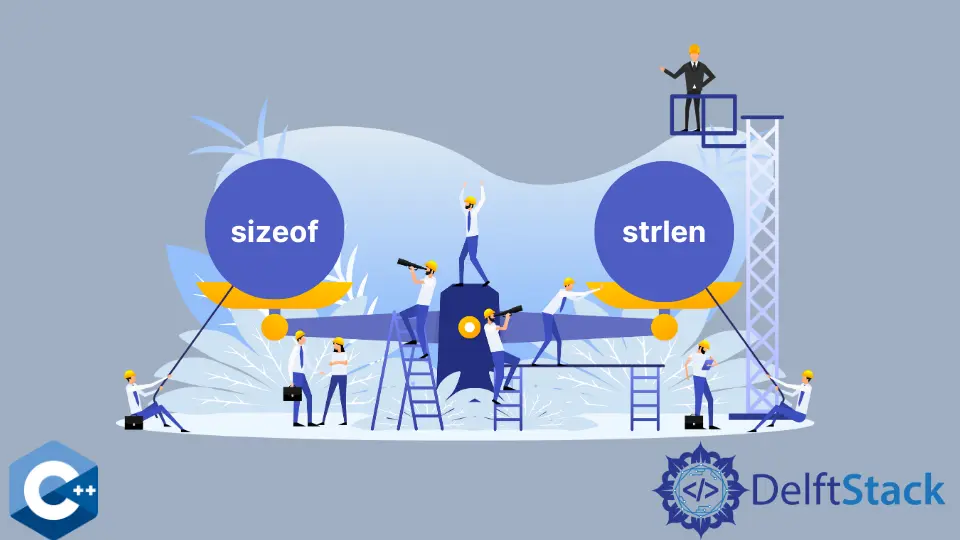
이 기사에서는 C++에서 문자열과 함께 strlen
함수와 반대로 sizeof
연산자를 사용할 때의 여러 차이점을 보여줍니다.
sizeof
연산자 특성 및 사용 시나리오
sizeof
연산자는 주어진 표현식 또는 데이터 유형에 대한 스토리지 크기를 검색하는 단항 연산자입니다. 이 연산자는 객체 크기를 바이트 단위로 평가하며 sizeof(char)
는 1
이 보장됩니다.
1바이트는 항상 8비트와 같으며 결과적으로 객체의 크기를 비트 단위로 계산할 수 있다는 잘못된 개념이 있습니다. 실제로 바이트는 언어 자체에 의해 8비트가 보장되지 않습니다. 주로 기본 하드웨어 플랫폼에 따라 다릅니다. 그러나 대부분의 최신 범용 하드웨어는 8비트 바이트를 사용합니다.
sizeof
연산자는 함수 또는 불완전한 유형 또는 비트 필드를 포함하는 표현식에 적용할 수 없습니다. 다음 예제 코드에서 sizeof
연산자를 다른 유형의 두 배열에 적용하고 결과는 플랫폼에서도 동일할 것입니다. char
는 1바이트 크기가 보장되므로 20자 배열은 20바이트를 차지합니다.
반면에 long long
데이터 유형은 구현에 따라 정의되며 이 경우 8바이트가 됩니다. 따라서 총 배열 크기는 160바이트입니다. 다음 예의 마지막 줄에 표시된 것처럼 전체 배열 sizeof
를 한 요소 sizeof
로 나누어 배열의 요소 수를 찾을 수도 있습니다.
#include <iostream>
using std::cout;
using std::endl;
int main() {
char arr[20];
long long arr2[20];
cout << "sizeof ( array of 20 long longs ) : " << sizeof arr << endl;
cout << "sizeof ( array of 20 long longs ) : " << sizeof arr2 << endl;
cout << "length of array of 20 chars) : "
<< ((sizeof arr) / (sizeof arr[0])) << endl;
cout << "length of array of 20 long longs ): "
<< ((sizeof arr2) / (sizeof arr2[0])) << endl;
return EXIT_SUCCESS;
}
출력:
sizeof ( array of 20 long longs ) : 20
sizeof ( array of 20 long longs ) : 160
length of array of 20 chars) : 20
length of array of 20 long longs ): 20
sizeof
연산자를 사용하여 문자열 길이를 찾는 것은 잘못되었습니다. C++에서 문자열의 두 가지 표현 유형인 문자열과 std::string
클래스를 고려해 보겠습니다. 전자는 대부분 char
포인터를 사용하여 액세스되며 sizeof
를 적용하면 전체 문자열이 아닌 포인터 자체의 저장 크기를 검색합니다.
sizeof
연산자를 사용하여 std::string
객체의 크기를 검색하려고 하면 아래 코드 스니펫에 표시된 것처럼 문자열의 문자 수가 아닌 객체의 저장 크기를 얻습니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "sizeof char* : " << sizeof text1 << endl;
cout << "sizeof string: " << sizeof text2 << endl;
cout << "sizeof string.data: " << sizeof text2.data() << endl;
return EXIT_SUCCESS;
}
출력:
sizeof char* : 8
sizeof string: 32
sizeof string.data: 8
그런 다음 C 문자열 라이브러리의 나머지 부분인 strlen
함수를 얻었습니다. 주어진 문자열의 길이를 계산하고 함수는 바이트 수를 정수 값으로 반환합니다. strlen
은 유효한 문자열이 저장된 char
포인터나 std::string::c_str
이 반환하는 값에 적용될 수 있지만 C++ 프로그래머가 선택해서는 안 됩니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "strlen char* : " << strlen(text1) << endl;
cout << "strlen string: " << strlen(text2.c_str()) << endl;
return EXIT_SUCCESS;
}
출력:
strlen char* : 24
strlen string: 24
std::string::size
멤버 함수를 사용하여 문자열의 문자 수 검색
반대로 C++ 프로그래머는 std::string
클래스의 size
멤버 함수를 활용해야 합니다. 이는 문자열을 처리하는 더 안전한 방법을 제공하기 때문입니다. 문자열이 있는 경우 size
멤버 함수를 사용할 수도 있습니다. 새로운 string
객체를 생성하고 반환된 값에 대해 size
함수를 직접 호출해야 합니다. 다음 코드 예제에서는 두 시나리오의 기본 사용법을 보여줍니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
const char *text1 = "arbitrary string value 1";
string text2 = "arbitrary string value 2";
cout << "length char* : " << string(text1).size() << endl;
cout << "length string: " << text2.size() << endl;
return EXIT_SUCCESS;
}
출력:
length char* : 24
length string: 24
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook