How to Shift Elements in Array in C++
-
Shift Array Elements in C++ Using
std::rotate
-
Shift Array Elements in C++ Using a Custom Wrapper for
std::rotate
-
Shift Array Elements in C++ Using
std::rotate_copy
- Shift Array Elements in C++ Using a Temporary Array
- Conclusion
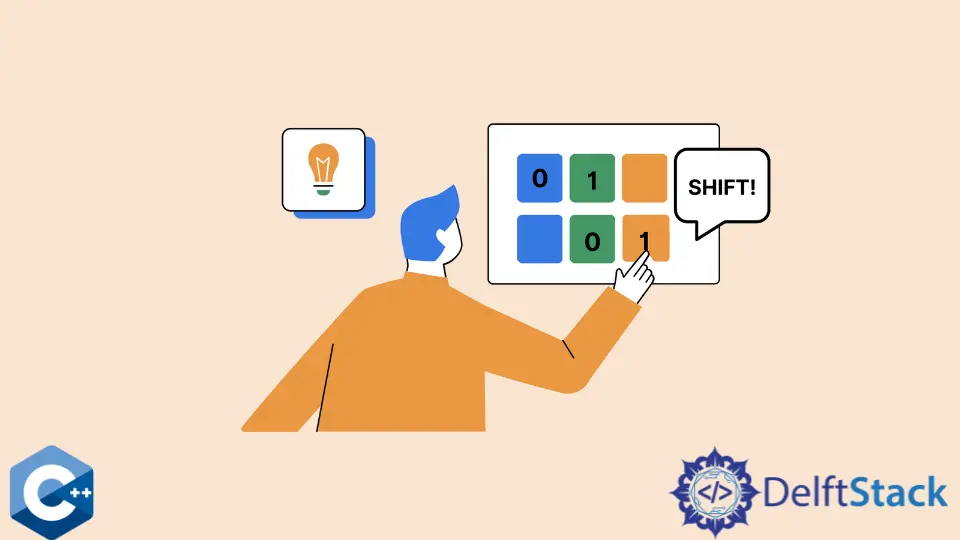
Array manipulation, including shifting elements, is a common task in programming. Shifting elements within an array involve rearranging its contents to the left or right by a certain number of positions.
In this article, we’ll explore various methods to shift array elements in C++, providing insights into their syntax, functionality, and use cases.
Shift Array Elements in C++ Using std::rotate
When it comes to shifting array elements, the std::rotate
function provides a concise and efficient solution. This standard library function is a part of the <algorithm>
header and can be a powerful tool for rearranging elements within a range.
The std::rotate
function takes three iterators as arguments and rotates the elements in the specified range. The three iterators define the range: the beginning of the range, the element to become the new first element after the rotation, and the end of the range.
The function syntax is as follows:
template <class ForwardIt>
void rotate(ForwardIt first, ForwardIt middle, ForwardIt last);
first
: Iterator pointing to the beginning of the range.middle
: Iterator pointing to the element that should be the new first element of the rotated range.last
: Iterator pointing to the end of the range.
Code Example 1: Shifting Array Elements to the Left
To shift array elements in C++ using std::rotate
, you can use iterators to define the range of elements to be shifted. Let’s consider a simple example of shifting elements to the left:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
std::cout << "Original Array: ";
for (const auto &item : vec) {
std::cout << item << " ";
}
std::cout << std::endl;
// Use std::rotate to shift elements to the left
std::rotate(vec.begin(), vec.begin() + 3, vec.end());
// Display the shifted array
std::cout << "Shifted Array: ";
for (const auto &item : vec) {
std::cout << item << " ";
}
std::cout << std::endl;
return 0;
}
In this example, we begin by creating a vector named vec
containing the integers from 1 to 10. The original array is displayed using a loop that iterates over each element in the vector.
Next, the std::rotate
function is employed to shift the array elements to the left. The second argument of std::rotate
(vec.begin() + 3
) specifies that the element at index 3 should become the new first element after the rotation.
Following the rotation, the shifted array is displayed again using a loop. The output showcases the result of shifting the elements to the left.
Code Output:
Code Example 2: Shifting Array Elements to the Right
Now, let’s shift array elements to the right using std::rotate
:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
std::cout << "Original Array: ";
for (const auto &item : vec) {
std::cout << item << " ";
}
std::cout << std::endl;
// Use std::rotate to shift elements to the right
std::rotate(vec.rbegin(), vec.rbegin() + 3, vec.rend());
// Display the shifted array
std::cout << "Shifted Array: ";
for (const auto &item : vec) {
std::cout << item << " ";
}
std::cout << std::endl;
return 0;
}
Similarly, this example begins with the creation of a vector, vec
, containing the integers from 1 to 10. The original array is displayed, and then std::rotate
is used to shift the elements to the right.
In this case, reverse iterators (vec.rbegin()
and vec.rend()
) are employed to ensure the correct rotation direction.
After the rotation, the shifted array is displayed again. The output illustrates the result of shifting the elements to the right.
Code Output:
std::rotate
is a versatile algorithm for shifting array elements in C++. It allows for both left and right rotations, making it a valuable tool for array manipulation in your C++ programs.
Shift Array Elements in C++ Using a Custom Wrapper for std::rotate
While std::rotate
is a powerful tool for shifting array elements in C++, creating a custom wrapper function around it can enhance flexibility and encapsulate the shifting logic. This approach allows you to tailor the shifting operation according to specific requirements or create a more intuitive interface.
The wrapper function requires only two arguments - the array object to be rotated and an integer representing the number of positions to shift. The sign of the integer determines the direction of rotation, with positive values indicating rightward rotation and negative values indicating leftward rotation.
Let’s design a custom wrapper function named rotateArrayElements
:
template <typename T>
int rotateArrayElements(T &v, int dir) {
if (dir > 0) {
rotate(v.rbegin(), v.rbegin() + dir, v.rend());
return 0;
} else if (dir < 0) {
rotate(v.begin(), v.begin() + abs(dir), v.end());
return 0;
} else {
return 1;
}
}
In this template function:
T
: Template type representing the array type (e.g.,std::vector
,std::array
).v
: Reference to the array object to be rotated.dir
: Integer representing the number of positions to shift. Positive values indicate rightward rotation; negative values indicate leftward rotation.
Code Example: Shifting Array Elements With the Custom Wrapper
Let’s demonstrate the usage of this custom wrapper function with both a std::array
and a std::vector
:
#include <algorithm>
#include <array>
#include <iostream>
#include <vector>
using namespace std;
template <typename T>
void printElements(T &v) {
cout << "[ ";
for (const auto &item : v) {
cout << item << ", ";
}
cout << "\b\b ]" << endl;
}
template <typename T>
int rotateArrayElements(T &v, int dir) {
if (dir > 0) {
rotate(v.rbegin(), v.rbegin() + dir, v.rend());
return 0;
} else if (dir < 0) {
rotate(v.begin(), v.begin() + abs(dir), v.end());
return 0;
} else {
return 1;
}
}
int main() {
array<int, 10> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Shifting std::array elements to the right
rotateArrayElements(arr, 3);
cout << "Shifted std::array: ";
printElements(arr);
// Shifting std::vector elements to the left
rotateArrayElements(vec, -3);
cout << "Shifted std::vector: ";
printElements(vec);
return 0;
}
In this example, we first define a utility function, printElements
, to display the contents of an array. The custom wrapper function, rotateArrayElements
, is then employed to shift the elements of both a std::array
and a std::vector
.
The wrapper function checks the sign of the dir
parameter to determine the rotation direction. If dir
is positive, it performs rightward rotation using reverse iterators; if negative, it performs leftward rotation using regular iterators.
The absolute value of dir
is used to calculate the rotation magnitude. The printElements
function is called to display the shifted arrays.
Code Output:
The custom wrapper function for std::rotate
provides a convenient and readable way to shift array elements in C++.
Shift Array Elements in C++ Using std::rotate_copy
In addition to std::rotate
, C++ offers another option for shifting array elements: the std::rotate_copy
function. This function, like std::rotate
, is part of the <algorithm>
header and provides a mechanism for creating a new sequence with the elements rotated in a specified range.
However, unlike std::rotate
, the std::rotate_copy
function performs a rotation on a specified range and creates a new sequence with the rotated elements while leaving the original sequence unchanged. This function can be beneficial when you want to keep the original array intact while obtaining a rotated version of it.
The syntax of std::rotate_copy
is as follows:
template <class ForwardIt, class OutputIt>
OutputIt rotate_copy(ForwardIt first, ForwardIt middle, ForwardIt last,
OutputIt result);
first
: Iterator pointing to the beginning of the range.middle
: Iterator pointing to the element that should be the new first element of the rotated range.last
: Iterator pointing to the end of the range.result
: Iterator pointing to the beginning of the destination range where the rotated elements will be copied.
Code Example: Shifting Array Elements With std::rotate_copy
Let’s demonstrate how to use std::rotate_copy
to shift array elements:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
template <typename T>
void printElements(T &v) {
cout << "[ ";
for (const auto &item : v) {
cout << item << ", ";
}
cout << "\b\b ]" << endl;
}
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
cout << "Original Array: ";
printElements(vec1);
// Create a vector for the rotated elements using std::rotate_copy
vector<int> vec2(vec1.size());
rotate_copy(vec1.begin(), vec1.begin() + 3, vec1.end(), vec2.begin());
// Display the rotated array
cout << "Rotated Array: ";
printElements(vec2);
return 0;
}
In this example, we begin by defining a utility function, printElements
, to display the contents of an array. The original array, vec1
, is displayed using this function.
Next, we create another vector, vec2
, with the same size as the original array to store the rotated elements.
The std::rotate_copy
function is then used to copy the rotated elements from vec1
to vec2
. The second argument (vec1.begin() + 3
) specifies that the element at index 3 should become the new first element of the rotated range.
Finally, the rotated array, vec2
, is displayed using the printElements
function.
Code Output:
std::rotate_copy
provides an alternative method for shifting array elements while preserving the original array. This function is particularly useful when you need to create a new sequence with the rotated elements rather than modifying the existing array.
Shift Array Elements in C++ Using a Temporary Array
Shifting array elements can also be achieved using a temporary array as an intermediary step. This approach involves copying elements to a new location based on the desired shift, creating a temporary array to store the intermediate state, and then copying the modified array back to the original one.
Although it may not be as concise as using certain standard library functions, this method provides a straightforward and understandable solution.
Code Example: Shifting Array Elements With a Temporary Array
Let’s dive into the code example demonstrating how to shift array elements using a temporary array:
#include <iostream>
#include <vector>
using namespace std;
template <typename T>
void printElements(T &v) {
cout << "[ ";
for (const auto &item : v) {
cout << item << ", ";
}
cout << "\b\b ]" << endl;
}
void shiftArray(int arr[], int size, int shift) {
int temp[size];
for (int i = 0; i < size; ++i) {
temp[(i + shift) % size] = arr[i];
}
for (int i = 0; i < size; ++i) {
arr[i] = temp[i];
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int size = sizeof(arr) / sizeof(arr[0]);
// Display the original array
cout << "Original Array: ";
printElements(arr);
// Shift array elements using a temporary array
shiftArray(arr, size, 3);
// Display the shifted array
cout << "Shifted Array: ";
printElements(arr);
return 0;
}
In this example, a utility function named printElements
is defined to display the contents of an array. The main function starts by creating an integer array, arr
, representing the original array. The original array is displayed using the printElements
function.
The core of the shifting operation is performed by the shiftArray
function. It takes three parameters: the array to be shifted (arr
), the size of the array (size
), and the number of positions to shift (shift
).
Inside the function, a temporary array temp
is created with the same size as the original array. This array will be used to temporarily store the shifted elements.
int temp[size];
A loop iterates over each element of the original array, and the elements are copied to the temporary array with the desired shift. The expression (i + shift) % size
ensures that the elements wrap around when reaching the end of the array, creating a circular shift.
for (int i = 0; i < size; ++i) {
temp[(i + shift) % size] = arr[i];
}
Another loop is used to copy the elements from the temporary array back to the original array.
for (int i = 0; i < size; ++i) {
arr[i] = temp[i];
}
After the shifting operation, the modified array is displayed using the printElements
function.
Code Output:
The temporary array method provides a straightforward approach to shift array elements in C++. Although it involves additional memory for the temporary array, it can be a practical solution in situations where preserving the original array is essential.
Conclusion
Shifting array elements is a fundamental operation in array manipulation, and in C++, various methods are available to achieve this task. Choosing the right method depends on factors such as efficiency, memory considerations, and the specific requirements of the task at hand.
The std::rotate
algorithm offers elegance and efficiency, while a custom wrapper function provides a simplified interface. std::rotate_copy
adds the capability to create a new array with rotated elements. Meanwhile, the use of a temporary array provides a straightforward solution, albeit with additional memory usage.
Select the most suitable approach for your applications to ensure efficient and effective array manipulation. Whether in algorithm design, data processing, or other programming tasks, the ability to shift array elements is a valuable skill that enhances the versatility of C++ programmers.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook