How to Return Multiple Values From Function in C++
-
Use
struct
to Return Multiple Values From a Function in C++ -
Use
std::pair
to Return Multiple Values From a Function in C++ - Use Array to Return Multiple Values From a Function in C++
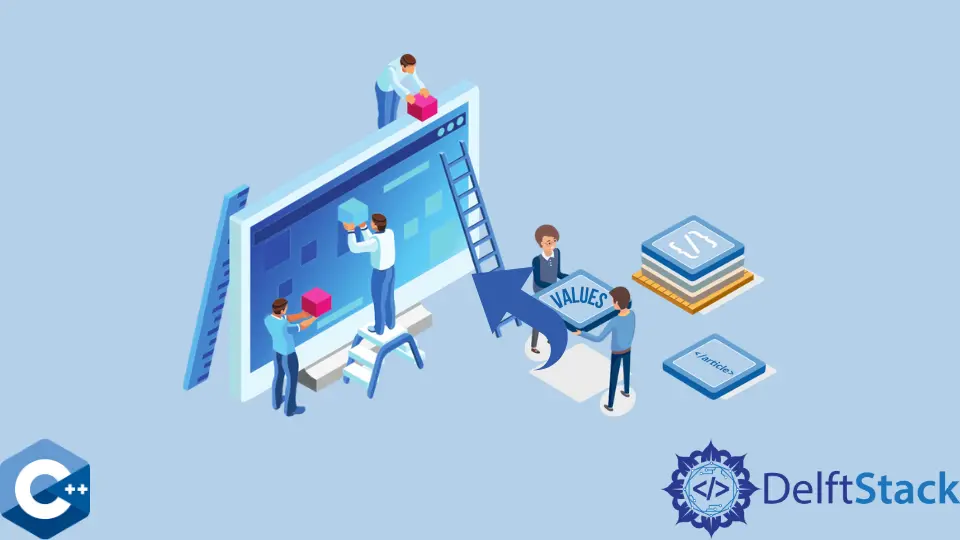
This article will demonstrate multiple methods about how to return multiple values from a function in C++.
Use struct
to Return Multiple Values From a Function in C++
Custom-defined struct
variables can be used utilized to return multiple values from functions. Namely, we demonstrate an example that implements a two-data member structure to return an int
pair from the function that searches for the maximum/minimum in the vector
of integers. Notice that one can define any type of struct
to fit their needs and expand the data member count. In this case, we declare the local struct
variable in the findMaxMin
function scope and return it by value to the main
routine. The struct
variable can also be initialized in the main
function and passed to the findMaxMin
by reference to store the result values there.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
struct IntPair {
int first, second;
} typedef IntPair;
IntPair findMaxMin(vector<int> &arr) {
IntPair ret;
int max = arr[0];
int min = arr[0];
for (const auto &item : arr) {
if (item > max) max = item;
if (item < min) min = item;
}
ret.first = max;
ret.second = min;
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
auto ret = findMaxMin(array);
cout << "Maximum element is " << ret.first << ", Minimum - " << ret.second
<< endl;
return EXIT_SUCCESS;
}
Output:
Maximum element is 20, Minimum - 1
Use std::pair
to Return Multiple Values From a Function in C++
std::pair
is provided the C++ standard library, and it can store two heterogeneous objects like a pair. Thus, we can reimplement the previous example without defining our custom struct
as the std::pair
has already extensive functionality builtin. One downside is that it can only return two values if we don’t store some other multi-element structures in them. Note that, we swapped max
/min
finding algorithms with STL ones - std::max_element
and std::min_element
respectively.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
std::pair<int, int> findMaxMin2(vector<int> &arr) {
std::pair<int, int> ret;
auto max = std::max_element(arr.begin(), arr.end());
auto min = std::min_element(arr.begin(), arr.end());
ret.first = arr.at(std::distance(arr.begin(), max));
ret.second = arr.at(std::distance(arr.begin(), min));
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
auto ret = findMaxMin2(array);
cout << "Maximum element is " << ret.first << ", Minimum - " << ret.second
<< endl;
return EXIT_SUCCESS;
}
Output:
Maximum element is 20, Minimum - 1
Use Array to Return Multiple Values From a Function in C++
Alternatively, we can declare a C-style array to store and return multiple values from the function. This method provides a more straightforward interface for working with a larger amount of values. It’s more efficient to declare an array in the caller function and pass its address to the callee. This way, even huge arrays can be passed using just one pointer without any growing overhead.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
int *findMaxMin2(vector<int> &arr, int ret[]) {
auto max = std::max_element(arr.begin(), arr.end());
auto min = std::min_element(arr.begin(), arr.end());
ret[0] = arr.at(std::distance(arr.begin(), max));
ret[1] = arr.at(std::distance(arr.begin(), min));
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
int ret_array[2];
auto ret = findMaxMin2(array, ret_array);
cout << "Maximum element is " << ret[0] << ", Minimum - " << ret[1] << endl;
return EXIT_SUCCESS;
}
Output:
Maximum element is 20, Minimum - 1
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook