C++의 함수에서 여러 값 반환
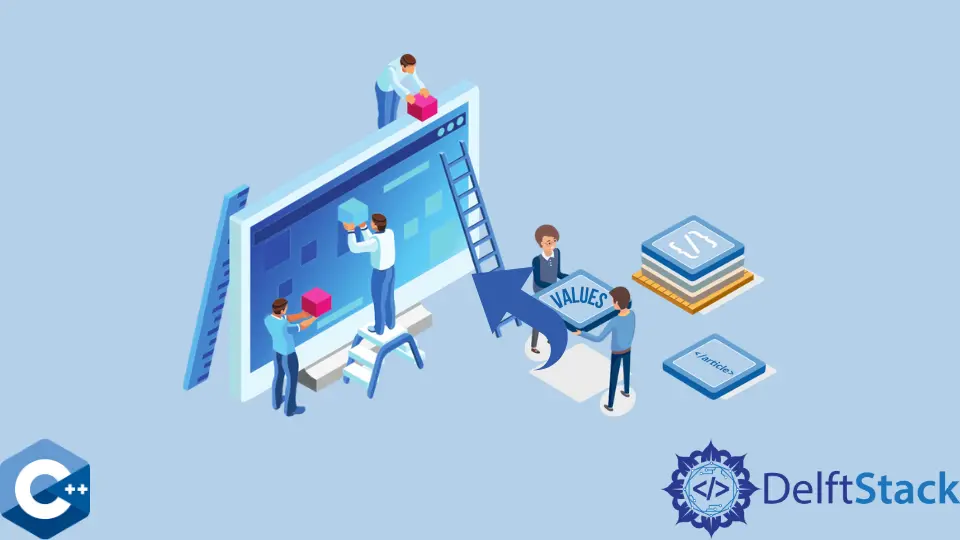
이 기사에서는 C++의 함수에서 여러 값을 반환하는 방법에 대한 여러 메서드를 보여줍니다.
struct
를 사용하여 C++의 함수에서 여러 값 반환
사용자 정의struct
변수를 사용하여 함수에서 여러 값을 반환 할 수 있습니다. 즉, 정수의벡터
에서 최대 / 최소를 검색하는 함수에서int
쌍을 반환하기 위해 두 데이터 멤버 구조를 구현하는 예를 보여줍니다. 필요에 맞게 모든 유형의struct
를 정의하고 데이터 멤버 수를 확장 할 수 있습니다. 이 경우findMaxMin
함수 범위에서 로컬struct
변수를 선언하고main
루틴에 값별로 반환합니다. struct
변수는main
함수에서 초기화 될 수 있으며 참조를 통해findMaxMin
에 전달되어 결과 값을 저장할 수 있습니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
struct IntPair {
int first, second;
} typedef IntPair;
IntPair findMaxMin(vector<int> &arr) {
IntPair ret;
int max = arr[0];
int min = arr[0];
for (const auto &item : arr) {
if (item > max) max = item;
if (item < min) min = item;
}
ret.first = max;
ret.second = min;
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
auto ret = findMaxMin(array);
cout << "Maximum element is " << ret.first << ", Minimum - " << ret.second
<< endl;
return EXIT_SUCCESS;
}
출력:
Maximum element is 20, Minimum - 1
std::pair
를 사용하여 C++의 함수에서 여러 값을 반환합니다
std::pair
는 C++ 표준 라이브러리를 제공하며 한 쌍처럼 2 개의 이기종 객체를 저장할 수 있습니다. 따라서std::pair
에 이미 광범위한 기능이 내장되어 있으므로 사용자 정의struct
를 정의하지 않고 이전 예제를 다시 구현할 수 있습니다. 한 가지 단점은 다른 다중 요소 구조를 저장하지 않으면 두 값만 반환 할 수 있다는 것입니다. 우리는max
/min
찾기 알고리즘을 STL 알고리즘 인std::max_element
및std::min_element
로 각각 교체했습니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
std::pair<int, int> findMaxMin2(vector<int> &arr) {
std::pair<int, int> ret;
auto max = std::max_element(arr.begin(), arr.end());
auto min = std::min_element(arr.begin(), arr.end());
ret.first = arr.at(std::distance(arr.begin(), max));
ret.second = arr.at(std::distance(arr.begin(), min));
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
auto ret = findMaxMin2(array);
cout << "Maximum element is " << ret.first << ", Minimum - " << ret.second
<< endl;
return EXIT_SUCCESS;
}
출력:
Maximum element is 20, Minimum - 1
배열을 사용하여 C++의 함수에서 여러 값 반환
또는 함수에서 여러 값을 저장하고 반환하기 위해 C 스타일 배열을 선언 할 수 있습니다. 이 방법은 많은 양의 값으로 작업하기위한보다 간단한 인터페이스를 제공합니다. 호출자 함수에서 배열을 선언하고 해당 주소를 수신자에게 전달하는 것이 더 효율적입니다. 이렇게하면 오버 헤드없이 하나의 포인터 만 사용하여 거대한 배열도 전달할 수 있습니다.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
int *findMaxMin2(vector<int> &arr, int ret[]) {
auto max = std::max_element(arr.begin(), arr.end());
auto min = std::min_element(arr.begin(), arr.end());
ret[0] = arr.at(std::distance(arr.begin(), max));
ret[1] = arr.at(std::distance(arr.begin(), min));
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18, 19, 20};
int ret_array[2];
auto ret = findMaxMin2(array, ret_array);
cout << "Maximum element is " << ret[0] << ", Minimum - " << ret[1] << endl;
return EXIT_SUCCESS;
}
출력:
Maximum element is 20, Minimum - 1
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook