How to Resize Array in C++
-
Resize an Array in C++ Using the
resize
Method -
Resize an Array in C++ Using the
erase
Method - Resize an Array in C++ With a Custom-Defined Function
- Resize an Array in C++ Using Dynamic Memory Allocation
- Conclusion
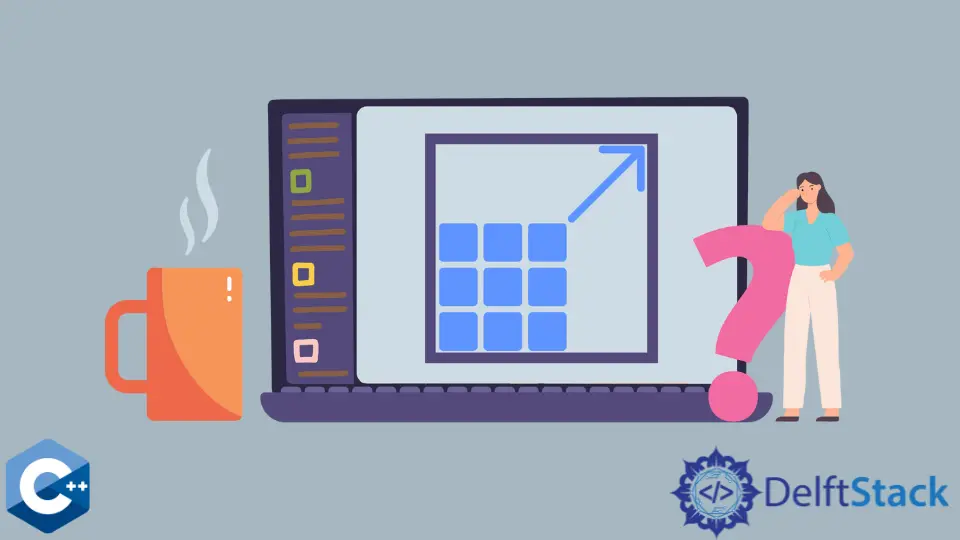
Resizing arrays is a common task in C++ programming, often necessitated by changing data requirements during runtime. Properly managing array sizes is crucial for efficient memory usage and program functionality.
In this article, we will explore various methods to resize arrays in C++, including built-in functions like resize
and erase
for vectors, custom-defined functions tailored to specific needs, and dynamic memory allocation for manual control.
Resize an Array in C++ Using the resize
Method
One of the ways to resize a dynamic array in C++ is by using the resize
method.
The resize
method is a built-in function of the std::vector
class in C++. It allows us to modify the number of elements contained within the vector, either by expanding or shrinking it.
The method has two main syntaxes:
- Resize without Initialization:
#include <vector>
std::vector<T> myVector;
myVector.resize(newSize);
- Resize with Initialization:
#include <vector>
std::vector<T> myVector;
myVector.resize(newSize, initValue);
Here, T
represents the type of elements stored in the vector, myVector
is the vector you want to resize, newSize
is the new size you want the vector to have, and initValue
is an optional argument that initializes the new elements with a specific value.
If you only want to change the size without initializing the new elements, use the first syntax. If you want to initialize the new elements with a specific value, use the second syntax.
The resize
method operates in two scenarios:
- If
newSize
is less than the current size, it truncates the vector by removing elements from the end. - If
newSize
is greater than the current size, it inserts additional elements, initialized with either the default value (if no optional value is provided) or the specified optional value.
Now, let’s delve into a complete working example to illustrate the usage of the resize
method.
Code Example: Resizing a Vector With resize
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> myVector = {1, 2, 3, 4, 5};
cout << "Original Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
int newSize = 8;
myVector.resize(newSize, 0); // Optional value set to 0
cout << "Resized Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
return 0;
}
In this example, we start by including necessary headers and declaring a vector of integers named myVector
.
We initialize it with values for demonstration purposes. The original vector is displayed using a simple loop.
Next, we introduce the resize
method to adjust the size of the vector. We set newSize
to 8, indicating that we want to resize the vector to contain 8 elements.
Additionally, the second argument of resize
is set to 0, specifying that any newly added elements should be initialized with the value 0.
Finally, we display the resized vector to observe the changes made by the resize
method.
Output:
In the output, we can see that the original vector containing elements 1 through 5 has been resized to include 3 additional elements, initialized with the value 0. The resize
method efficiently handles the array resizing operation, offering a flexible and straightforward approach in C++.
Resize an Array in C++ Using the erase
Method
Resizing an array can also be accomplished using the erase
method.
The erase
method is a member function of the std::vector
class in C++. It is commonly used to remove elements from a vector, but it can also be employed to resize the vector dynamically by erasing a specific range of elements.
Its syntax is as follows:
#include <iterator>
#include <vector>
// Syntax for erasing a single element
iterator erase(iterator position);
// Syntax for erasing a range of elements
iterator erase(iterator first, iterator last);
Here, iterator
is a placeholder representing the iterator type associated with the vector. The erase
method takes one or two iterators as arguments:
position
: Iterator pointing to the element to be removed.first
andlast
: Iterators delimiting the range of elements to be removed.
The erase
method operates in two primary scenarios:
- If provided with a position, it removes the single element at that position.
- If given a range defined by start and end iterators, it removes all elements within that range.
Now, let’s illustrate the usage of the erase
method through a complete working example.
Code Example: Resizing a Vector Using the erase
Method
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> myVector = {1, 2, 3, 4, 5};
cout << "Original Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
myVector.erase(myVector.begin() + 2, myVector.end());
cout << "Resized Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
return 0;
}
In the above example, we begin by including necessary headers and declaring a vector of integers named myVector
, initialized with values for demonstration. The original vector is displayed using a simple loop.
Next, we introduce the erase
method to resize the vector. The method is applied to remove elements within the specified range, defined by iterators pointing to the start and end positions.
In this case, we remove elements starting from the third position (index 2) to the end of the vector.
Finally, we display the resized vector to observe the changes made by the erase
method.
Output:
Here, we can see that the original vector containing elements 1 through 5 has been resized using the erase
method. The elements starting from the third position have been removed, resulting in a vector with only the first two elements.
The erase
method allows for dynamic resizing by removing a specific range of elements and adapting the size of the array to the current requirements. Unlike manual memory management, the erase
method efficiently handles the removal of elements, maintaining the integrity of the container.
When using the erase
method, it’s important to ensure that the indices provided are within the valid range of the vector to avoid undefined behavior.
Resize an Array in C++ With a Custom-Defined Function
While standard containers like std::vector
simplify dynamic array management, creating a custom-defined function can offer more control and cater to specific requirements. This method involves creating a function that iterates through the vector and removes a specified number of elements from the end using the pop_back
built-in function.
The custom-defined function for resizing a vector below involves a loop and the use of the pop_back
function. The syntax for such a function is outlined below:
template <typename T>
void resizeVector(std::vector<T>& vec, int elementsToRemove) {
for (int i = 0; i < elementsToRemove; ++i) {
vec.pop_back();
}
}
vec
: A reference to the vector that needs resizing.elementsToRemove
: The number of elements to be removed from the end of the vector.
The resizeVector
function takes two parameters: a reference to a vector (vec
) and an integer representing the number of elements to be removed from the end (elementsToRemove
). Inside the function, a for
loop iterates elementsToRemove
times, and in each iteration, the pop_back
function is called, removing the last element from the vector.
Now, let’s explore a complete working example to illustrate the usage of this custom-defined function.
Code Example: Resizing a Vector With a Custom-Defined Function
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void resizeVector(vector<T>& vec, int elementsToRemove) {
for (int i = 0; i < elementsToRemove; ++i) {
vec.pop_back();
}
}
int main() {
vector<int> myVector = {1, 2, 3, 4, 5};
cout << "Original Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
resizeVector(myVector, 2);
cout << "Resized Vector: ";
for (const auto& element : myVector) {
cout << element << " ";
}
cout << endl;
return 0;
}
Here, the main
function begins by initializing a vector named myVector
with the values {1, 2, 3, 4, 5}
. It then prints the original vector using a for
loop and displays each element separated by a space.
Next, the custom-defined function resizeVector
is called, passing myVector
and the value 2
as arguments. This operation removes the last two elements from the vector, effectively resizing it.
Finally, the resized vector is displayed using another for
loop, and the program returns 0
to indicate successful execution.
Output:
The output shows that the original vector, containing elements 1 through 5, has been resized using the custom-defined function. Two elements have been removed from the end of the vector, resulting in a resized vector with only the first three elements.
This custom-defined function offers a personalized and effective way to resize vectors based on specific requirements.
Resize an Array in C++ Using Dynamic Memory Allocation
In C++, resizing an array can also be achieved through dynamic memory allocation using the new
and delete
operators. This method provides manual control over memory allocation, allowing us to resize an array by creating a new array with a different size and copying elements from the old array to the new one.
Dynamic memory allocation involves requesting memory from the heap during runtime. In C++, the new
operator is used for dynamic memory allocation, while the delete
operator is used for deallocating the memory when it is no longer needed.
// Syntax for dynamic memory allocation using new
int* dynamicArray = new int[size];
// Syntax for deallocating dynamic memory using delete
delete[] dynamicArray;
The process involves the following steps:
-
Create a new array with the desired size using the
new
operator. -
Copy elements from the old array to the new array.
-
Release the memory occupied by the old array using the
delete
operator. -
Update the pointer to point to the new array.
Now, let’s delve into a complete working example to illustrate the usage of dynamic memory allocation for resizing an array.
Code Example: Resizing an Array With Dynamic Memory Allocation
#include <iostream>
int main() {
int size = 5;
int* originalArray = new int[size]{1, 2, 3, 4, 5};
std::cout << "Original Array: ";
for (int i = 0; i < size; ++i) {
std::cout << originalArray[i] << " ";
}
std::cout << std::endl;
// Resizing the array to a new size
int newSize = 3;
int* newArray = new int[newSize];
// Copying elements from the original array to the new array
for (int i = 0; i < newSize; ++i) {
newArray[i] = originalArray[i];
}
// Releasing memory of the original array
delete[] originalArray;
// Updating the pointer to point to the new array
originalArray = newArray;
// Displaying the resized array
std::cout << "Resized Array: ";
for (int i = 0; i < newSize; ++i) {
std::cout << originalArray[i] << " ";
}
std::cout << std::endl;
// Release memory of the resized array
delete[] originalArray;
return 0;
}
In this C++ code, we begin by creating the original array using dynamic memory allocation (new int[size]
) and initializing it with values {1, 2, 3, 4, 5}
. We then display the original array using a loop.
Next, we want to resize the array to a new size (newSize = 3
). We allocate memory for the new array using new int[newSize]
and copy elements from the original array to the new one.
After that, we release the memory occupied by the original array using delete[] originalArray
and update the pointer to point to the new array (originalArray = newArray
).
Finally, we display the resized array and release the memory of the resized array using delete[] originalArray
.
Output:
The output demonstrates the process of resizing an array using dynamic memory allocation. The original array, initially containing elements 1 through 5, is resized to include only the first three elements.
This method offers manual control over memory management and is suitable for scenarios where dynamic resizing is required.
Conclusion
Resizing an array in C++ can be achieved through various methods, each catering to specific requirements. We explored techniques such as using the resize
method with vectors, the erase
method for selective removal, custom-defined functions for personalized resizing, and dynamic memory allocation for manual control over array size.
Whether leveraging built-in functions or employing dynamic memory management, the choice of method depends on the specific needs of the program. Understanding these diverse approaches equips you with the flexibility to adapt and efficiently manage array sizes in C++, ensuring optimal performance and resource utilization.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook