How to Trim a String in C++
-
Using the
std::string::erase
Method -
Using the
std::string::find_first_not_of
andstd::string::find_last_not_of
Methods - Using Regular Expressions for Trimming
- Conclusion
- FAQ
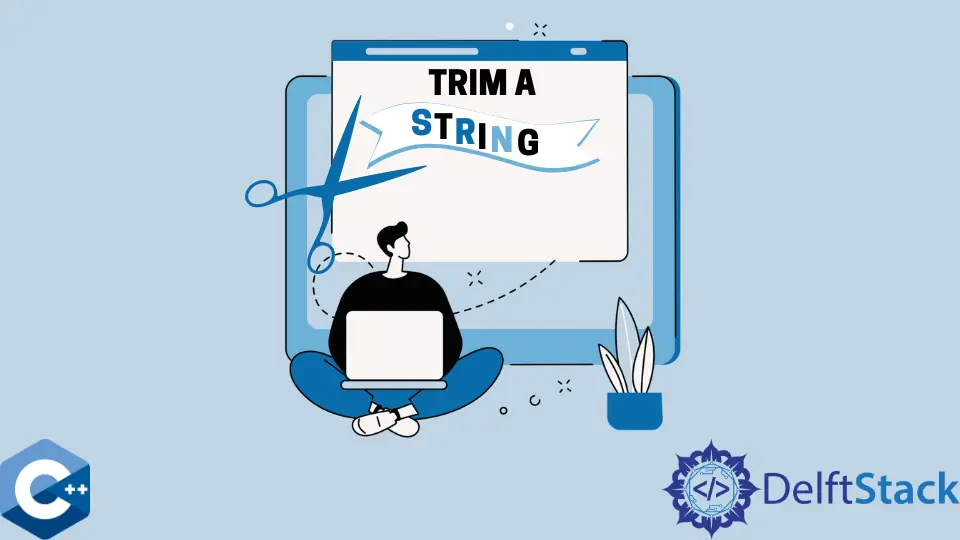
Trimming a string is a common task in programming, especially when dealing with user input or data processing. In C++, trimming refers to removing unwanted whitespace from the beginning and end of a string. This can ensure that your string data is clean and ready for further manipulation or analysis. While C++ does not have a built-in function specifically for trimming strings, you can easily achieve this using standard library functions.
In this article, we will explore several methods to trim a string in C++, providing clear examples and explanations to help you understand each approach. Whether you are a beginner or an experienced programmer, you will find useful insights here.
Using the std::string::erase
Method
One of the simplest ways to trim a string in C++ is by using the std::string::erase
method in conjunction with std::find_if
. This method allows you to remove characters from the beginning and end of a string based on a specific condition. Here’s how you can implement it:
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
std::string trim(const std::string& str) {
auto start = str.begin();
while (start != str.end() && std::isspace(*start)) {
++start;
}
auto end = str.end();
do {
--end;
} while (end != start && std::isspace(*end));
return std::string(start, end + 1);
}
int main() {
std::string input = " Hello, World! ";
std::string trimmed = trim(input);
std::cout << "'" << trimmed << "'" << std::endl;
return 0;
}
Output:
'Hello, World!'
In this example, we define a function named trim
that takes a constant reference to a string. The function uses iterators to find the first non-whitespace character and the last non-whitespace character. The std::isspace
function checks for whitespace characters. Finally, we create a new string that contains only the trimmed content and return it. This method is efficient and straightforward, making it a great choice for trimming strings in C++.
Using the std::string::find_first_not_of
and std::string::find_last_not_of
Methods
Another effective way to trim a string is by utilizing the std::string::find_first_not_of
and std::string::find_last_not_of
methods. These functions allow you to locate the positions of the first and last non-whitespace characters in the string. Here’s an example of how to use these methods:
#include <iostream>
#include <string>
std::string trim(const std::string& str) {
size_t start = str.find_first_not_of(" \t\n");
size_t end = str.find_last_not_of(" \t\n");
if (start == std::string::npos) {
return ""; // String is all whitespace
}
return str.substr(start, end - start + 1);
}
int main() {
std::string input = " C++ Programming! ";
std::string trimmed = trim(input);
std::cout << "'" << trimmed << "'" << std::endl;
return 0;
}
Output:
'C++ Programming!'
In this code, we define the trim
function that first finds the index of the first and last non-whitespace characters in the input string. If the entire string consists of whitespace, we return an empty string. Otherwise, we use std::string::substr
to extract the trimmed portion of the string. This method is particularly useful when dealing with strings that may contain various types of whitespace characters, such as spaces, tabs, or newlines.
Using Regular Expressions for Trimming
For those who prefer a more versatile approach, using regular expressions can also be an effective way to trim strings. The C++ standard library provides support for regular expressions through the <regex>
header. Here’s how you can trim a string using regex:
#include <iostream>
#include <string>
#include <regex>
std::string trim(const std::string& str) {
std::regex ws_re("\\s+"); // regex for whitespace
return std::regex_replace(str, ws_re, " ");
}
int main() {
std::string input = " Hello, World! ";
std::string trimmed = trim(input);
std::cout << "'" << trimmed << "'" << std::endl;
return 0;
}
Output:
' Hello, World! '
In this example, we define a regex pattern that matches one or more whitespace characters. The std::regex_replace
function replaces these matches with a single space. This approach is particularly powerful because it allows for more complex trimming scenarios, such as removing multiple spaces between words. However, keep in mind that regex can be overkill for simple trimming tasks and may introduce some performance overhead.
Conclusion
Trimming strings in C++ is an essential skill for any programmer. Whether you choose to use the std::string::erase
method, the find_first_not_of
and find_last_not_of
methods, or regular expressions, each approach has its advantages. By understanding these techniques, you can ensure that your string data is clean and ready for further processing. As you become more comfortable with these methods, you’ll find that trimming strings becomes a seamless part of your coding routine.
FAQ
-
what is string trimming in C++?
String trimming in C++ refers to the process of removing whitespace from the beginning and end of a string. -
how do I trim a string in C++?
You can trim a string in C++ using methods likestd::string::erase
,find_first_not_of
, or regular expressions. -
are there built-in functions for trimming strings in C++?
No, C++ does not have built-in functions specifically for trimming strings, but you can easily implement this functionality.
-
can I trim specific characters in C++?
Yes, you can modify the trimming functions to remove specific characters by adjusting the conditions used to find the start and end of the string. -
is there a performance difference between trimming methods?
Yes, some methods may be more efficient than others depending on the size of the string and the complexity of the trimming logic.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++