如何在 C++ 中删除字符串两边字符
Jinku Hu
2023年10月12日
C++
C++ String
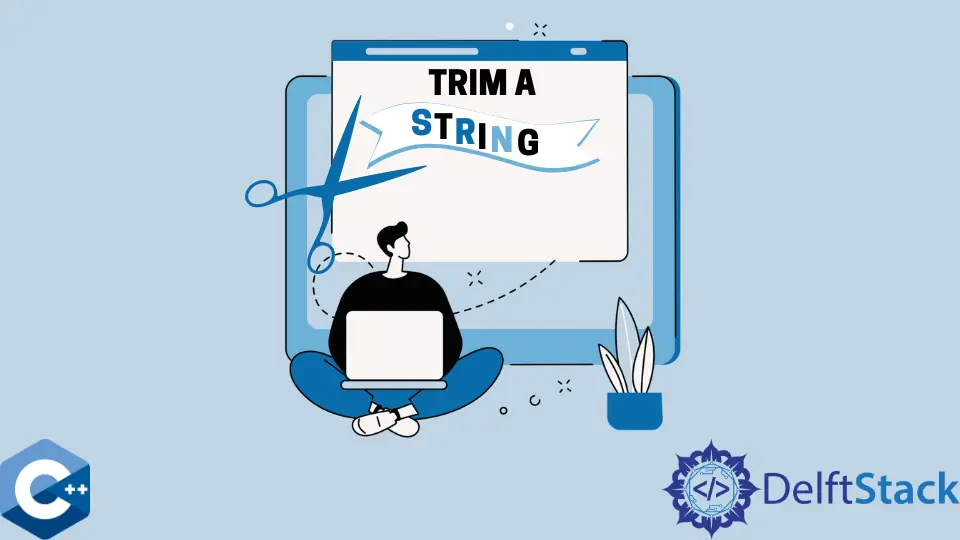
本文将介绍如何在 C++ 中删除字符串两边的字符。
使用 erase()
、find_first_not_of()
和 find_last_not_of()
方法实现删除字符串两边字符
由于标准的 C++ 库中不包含用于字符串修剪的函数,因此你需要自己实现这些函数,或者使用外部库,如 Boost(参见字符串算法)。
在下面的例子中,我们将演示如何使用 2 个内置的 std::string
方法来构造自定义函数。首先,我们实现了 leftTrim
函数,它从字符串的左侧删除作为参数传递的字符。我们任意指定要删除的字符 .
、,
、/
和空格。
leftTrim
函数调用 find_first_not_of
方法,以查找给定参数中第一个不等于 char
的字符,并返回一个位置给找到的字符。然后,erase
方法删除从开始直到找到的位置的字符范围。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string& leftTrim(string& str, string& chars) {
str.erase(0, str.find_first_not_of(chars));
return str;
}
int main() {
string chars_to_trim = ".,/ ";
string text = ",., C++ Standard";
cout << text << endl;
leftTrim(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
输出:
,., C++ Standard
C++ Standard
或者,我们可以使用 trimLeft
函数从字符串的右侧删除给定的字符。在这种情况下,我们利用 find_last_not_of
方法,搜索最后一个等于没有作为参数传递的字符。相应地,调用 erase
方法,参数为 found position + 1
。
注意,这两个函数都是对字符串进行原地操作,而不是返回删除字符后的副本。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string& rightTrim(string& str, string& chars) {
str.erase(str.find_last_not_of(chars) + 1);
return str;
}
int main() {
string chars_to_trim = ".,/ ";
string text = "C++ Standard /././";
cout << text << endl;
rightTrim(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
输出:
C++ Standard /././
C++ Standard
最后,我们可以结合前面的函数实现 trimString
函数,删除两边的字符。该函数的参数与左/右版本相同。trimString
调用 leftTrim
,将 rigthTrim
函数的结果作为参数传给它。你可以交换这些函数调用的位置而不改变程序的正确性。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string& leftTrim(string& str, string& chars) {
str.erase(0, str.find_first_not_of(chars));
return str;
}
string& rightTrim(string& str, string& chars) {
str.erase(str.find_last_not_of(chars) + 1);
return str;
}
string& trimString(string& str, string& chars) {
return leftTrim(rightTrim(str, chars), chars);
}
int main() {
string chars_to_trim = ".,/ ";
string text = ",,, .. C++ Standard ...";
cout << text << endl;
trimString(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
,,, .. C++ Standard ...
C++ Standard
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu