How to Print Numbers With Specified Decimal Points in C++
-
Using
std::fixed
andstd::setprecision
-
Using
std::cout
with Custom Formatting -
Using
printf
for Formatting - Conclusion
- FAQ
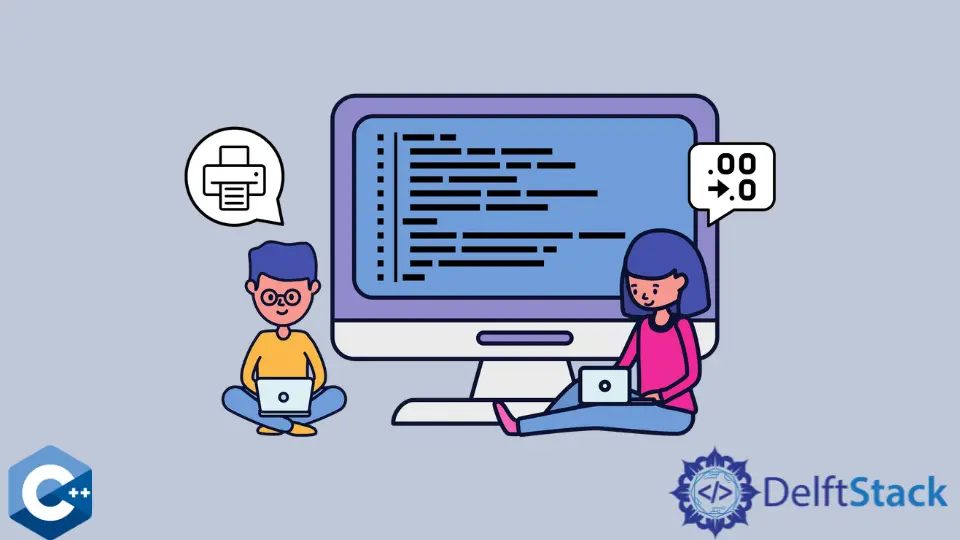
Printing numbers with a specific number of decimal points is a common requirement in programming, especially when dealing with financial data or scientific calculations. In C++, the standard library provides several ways to format output, making it easier to display numbers in a human-readable way.
This article will guide you through the various methods to print numbers with specified decimal points in C++. Whether you’re a beginner or an experienced programmer, you’ll find useful tips and examples to enhance your coding skills. By the end of this article, you’ll be able to format your numerical outputs effectively, ensuring clarity and precision in your programs.
Using std::fixed
and std::setprecision
One of the most straightforward ways to print numbers with a specific number of decimal points in C++ is by using the std::fixed
manipulator along with std::setprecision
. This method is part of the <iomanip>
header, which provides various utilities for input/output formatting.
Here’s how you can use these manipulators:
#include <iostream>
#include <iomanip>
int main() {
double number = 3.14159;
std::cout << std::fixed << std::setprecision(2) << number << std::endl;
return 0;
}
Output:
3.14
In this example, we first include the necessary headers, <iostream>
for standard input/output and <iomanip>
for manipulating the output format. The std::fixed
manipulator tells the output stream to use fixed-point notation instead of scientific notation. The std::setprecision(2)
function sets the precision to two decimal places. When we print the number
, it displays as 3.14
, rounded to two decimal points.
This method is particularly useful when you need consistent formatting across multiple outputs. You can adjust the precision by changing the argument in std::setprecision()
, allowing for flexible formatting based on your requirements.
Alternatively, we can fixate the decimal points after the comma and specify the precision value simultaneously. In the next code example, the fixed
function is called together with setprecision
, which itself takes int
value as a parameter.
Using std::cout
with Custom Formatting
Another method to print numbers with specified decimal points in C++ is through custom formatting using std::cout
. This approach provides more control over how numbers are displayed, allowing you to format them according to your needs.
Here’s an example:
#include <iostream>
int main() {
double number = 2.71828;
std::cout << "Formatted output: " << std::fixed << number * 100 << std::endl;
return 0;
}
Output:
Formatted output: 271.83
In this snippet, we multiply the number
by 100 before printing. The std::fixed
manipulator ensures that the number is displayed in fixed-point notation. However, we haven’t set any precision here, so it defaults to six decimal places. The output shows 271.83
, which is the result of the multiplication rounded to the nearest hundredth.
This method is particularly handy when you want to format numbers dynamically, depending on the calculations you perform. You can easily adjust the multiplication or apply different formatting options to achieve the desired output.
Using printf
for Formatting
If you’re familiar with C-style programming, you can also use the printf
function to print numbers with a specified number of decimal points. This method is part of the C standard library and can be particularly useful for those transitioning from C to C++.
Here’s how you can do it:
#include <cstdio>
int main() {
double number = 1.41421;
printf("Number with two decimal points: %.2f\n", number);
return 0;
}
Output:
Number with two decimal points: 1.41
In this example, the printf
function allows us to format the output directly. The format specifier %.2f
indicates that we want to print a floating-point number with two decimal places. The f
stands for floating point, and the .2
specifies the number of decimal points.
Using printf
can be more concise for those who are comfortable with C-style formatting. However, it is worth noting that C++ provides more robust and type-safe alternatives, such as the previous methods. Still, printf
remains a powerful tool for formatted output, especially in legacy code.
Conclusion
In conclusion, printing numbers with specified decimal points in C++ can be achieved through various methods. Whether you prefer the modern C++ approach with std::cout
and manipulators like std::fixed
and std::setprecision
, or the classic C-style printf
, there are options available to suit your coding style. Each method offers unique advantages, allowing you to format your numerical outputs effectively. By mastering these techniques, you can improve the clarity and precision of your programs, making them more user-friendly and professional.
FAQ
-
How do I print a number with three decimal points in C++?
You can usestd::setprecision(3)
along withstd::fixed
to print a number with three decimal points. -
Is there a way to format numbers using
printf
in C++?
Yes, you can use theprintf
function with format specifiers, such as%.3f
, to print numbers with a specified number of decimal points. -
What header files do I need for formatting output in C++?
You need to include<iostream>
for standard output and<iomanip>
for manipulators likestd::setprecision
. -
Can I change the precision dynamically in my program?
Yes, you can change the precision by callingstd::setprecision()
with different values as needed. -
Is using
printf
recommended in modern C++?
Whileprintf
is still valid, usingstd::cout
with manipulators is generally preferred for type safety and better integration with C++ features.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook