How to Use setprecision in C++
- Understanding setprecision
-
Use
setprecision()
andstd::fixed()
to Set Custom Precision for Floats -
Use
setprecision()
andstd::fixed()
to Align Floats to a Decimal Point - Conclusion
- FAQ
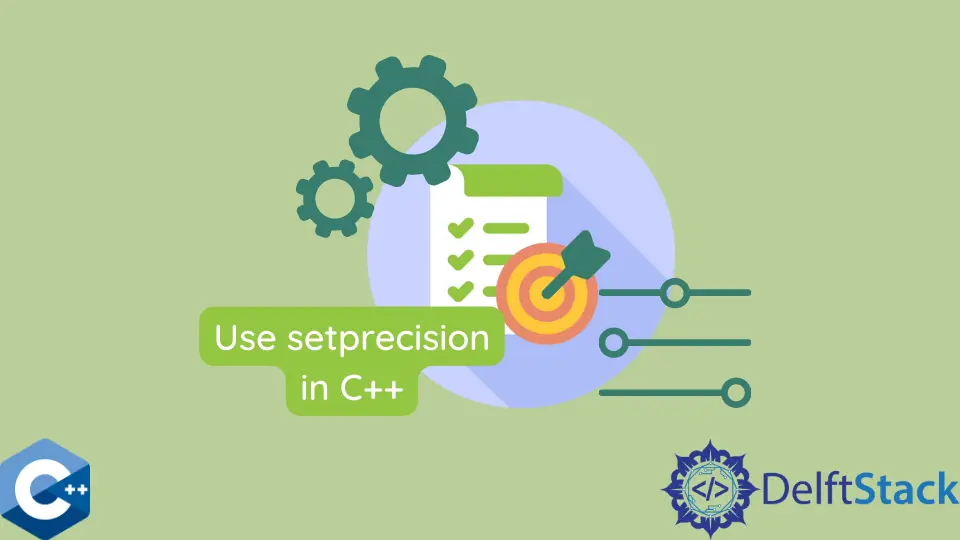
When it comes to formatting output in C++, one of the most useful tools at your disposal is the setprecision
function. This function allows you to control the number of digits displayed for floating-point numbers, which is essential for ensuring that your output is both readable and precise. Whether you’re dealing with scientific calculations or financial data, the ability to format numbers correctly can make a significant difference.
In this article, we’ll explore how to use the setprecision
function in C++, providing clear examples to help you grasp its functionality. By the end, you’ll have a solid understanding of how to apply this feature effectively in your own projects.
Understanding setprecision
The setprecision
function is part of the <iomanip>
header in C++. It manipulates the output format of floating-point numbers, allowing you to specify how many digits to display after the decimal point. By default, C++ displays floating-point numbers with a precision that may not meet your specific needs. This is where setprecision
comes into play.
To use setprecision
, you need to include the <iomanip>
header and use the std::cout
stream. You can control the precision of the output by passing an integer value to setprecision
. This integer represents the total number of digits displayed, including both the digits before and after the decimal point.
Here’s a simple example to illustrate how setprecision
works:
#include <iostream>
#include <iomanip>
int main() {
double num = 3.14159265359;
std::cout << std::fixed << std::setprecision(2) << num << std::endl;
std::cout << std::fixed << std::setprecision(5) << num << std::endl;
return 0;
}
Output:
3.14
3.14159
In this example, we first set the precision to 2, which results in the output of 3.14
. In the second case, we set the precision to 5, which gives us 3.14159
. The use of std::fixed
ensures that the output is in fixed-point notation, displaying the specified number of digits after the decimal point.
Use setprecision()
and std::fixed()
to Set Custom Precision for Floats
Alternatively, we can use setprecision()
and fixed()
stream manipulators in conjunction to print floating-point values with the same number of digits after the decimal point. The fixed()
method sets the fractional part of the number to a fixed length, which by default is 6 digits. In the following code sample, we output to cout
stream and call both manipulators before the number is inserted into the output.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013, 92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << fixed << setprecision(3) << i << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.231 | 2.234 | 0.324 | 0.012 | 26.949 | 11013.000 | 92.001 | 0.000 |
Use setprecision()
and std::fixed()
to Align Floats to a Decimal Point
Finally, we can combine the setw
, right
, setfill
, fixed
and setprecision
manipulators to output floating-point numbers aligned to the decimal point. setw
method specifies the output stream width with the number of characters passed as an argument. setfill
sets a character by which the unused space will be filled and right
method tells cout
the side to which filling operation applies.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013, 92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << std::setw(10) << std::right << std::setfill(' ') << fixed
<< setprecision(4) << i << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.2310
2.2343
0.3240
0.0120
26.9491
11013.0000
92.0011
0.0000
Conclusion
In summary, the setprecision
function in C++ is a powerful tool for controlling the output format of floating-point numbers. By understanding how to use it effectively, you can enhance the readability and precision of your program’s output. Whether you’re working with fixed-point or scientific notation, setprecision
gives you the flexibility to present your data in a way that meets your specific needs. As you continue to develop your C++ skills, mastering this function will undoubtedly prove beneficial in your coding journey.
FAQ
-
What is setprecision in C++?
setprecision is a function that controls the number of digits displayed for floating-point numbers in C++. -
Do I need to include any special headers to use setprecision?
Yes, you need to include theheader to use the setprecision function. -
Can I use setprecision with integers?
No, setprecision is specifically designed for floating-point numbers. -
What is the difference between fixed and scientific notation in C++?
Fixed notation displays numbers in standard decimal format, while scientific notation represents numbers in the form of a coefficient multiplied by a power of ten. -
How can I display a number with a specific number of decimal places?
You can use setprecision along with std::fixed to specify the number of decimal places you want to display.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook