How to Convert Float to Int in C++
- Use Direct Assignment to Convert Float to Int
- Use C-style Cast to Convert Float to Int
-
Use
static_cast
to Convert Float to Int - Use Rounding Strategies to Convert Float to Int
- Conclusion
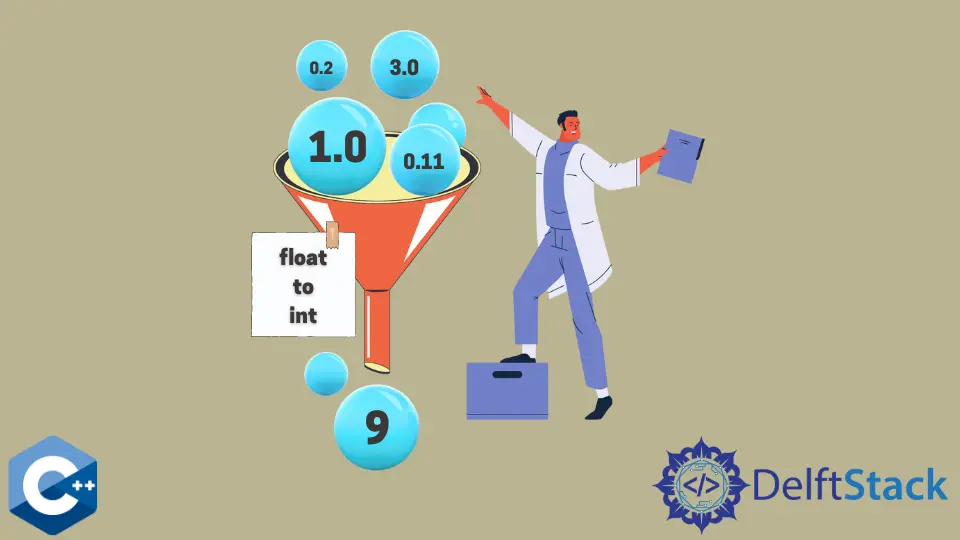
Converting a floating-point value (float
) to an integer (int
) is often required when dealing with numerical operations and data processing. In C++, there are several methods to achieve this conversion, each with its characteristics and use cases.
This article will demonstrate multiple methods of how to convert a float
to an int
in C++.
Use Direct Assignment to Convert Float to Int
Direct assignment (using the assignment operator) is a straightforward method to truncate a float
to an int
in C++. When you directly assign a float
variable to an int
variable, the C++ compiler automatically truncates the value by removing the decimal part and retaining only the whole number.
This method works efficiently for positive as well as negative float values.
Here’s a deeper discussion about direct assignment in the context of truncating floats to integers:
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<float> f_vec{12.123, 32.23, 534.333333339};
vector<int> i_vec;
i_vec.reserve(f_vec.size());
for (const auto &f : f_vec) {
i_vec.push_back(f);
}
for (const auto &i : i_vec) {
cout << i << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
12; 32; 534;
Here, we defined a vector of floats (f_vec
), initialized with three float values, and an empty vector of integers (i_vec
).
Then, the reserve
function is called on i_vec
to preallocate memory for the number of elements in f_vec
. This is a performance optimization to prevent unnecessary reallocations when elements are added.
Following this, we used a for
loop to iterate over each element in f_vec
(float values), convert them to integers, and append them to i_vec
using the push_back
function.
We then used another for
loop to iterate over each element in i_vec
(integers) and print them to the standard output separated by semicolons and spaces.
The direct assignment also works for negative float values, considering the sign of the float. For instance:
#include <iostream>
int main() {
float negativeFloatValue = -4.789;
int truncatedValueDirect = negativeFloatValue;
std::cout << "Original float value: " << negativeFloatValue << std::endl;
std::cout << "Truncated integer value: " << truncatedValueDirect << std::endl;
return 0;
}
Output:
Original float value: -4.789
Truncated integer value: -4
In this case, the negative float value -4.789
is directly assigned to an int
, resulting in truncatedValueDirect
being assigned the value -4
. The negative sign is preserved in the truncated integer value.
Use C-style Cast to Convert Float to Int
Another way to convert a float
to an int
is the use of a C-style cast. When using casting, we explicitly instruct the compiler to interpret the float value as an integer, disregarding the decimal part and potential loss of precision.
While C++ provides safer alternatives like static_cast
, reinterpret_cast
, const_cast
, and dynamic_cast
, the C-style cast using (typename)
notation is a more traditional and flexible approach.
However, it’s important to note that the C-style cast is considered unsafe and not recommended in modern C++ due to its potential for unintended behaviors and loss of type safety.
One specific concern with C-style casting is the possibility of undefined behavior when casting pointers to incomplete or unrelated types. Hence, it’s essential to exercise caution when utilizing C-style casts to ensure they are appropriate for the given context and won’t lead to unexpected issues.
Here’s an example of a float
to int
conversion using a C-style cast:
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<float> f_vec{12.123, 32.23, 534.333333339};
vector<int> i_vec;
i_vec.reserve(f_vec.size());
for (const auto &f : f_vec) {
i_vec.push_back(int(f));
}
for (const auto &i : i_vec) {
cout << i << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
12; 32; 534;
In the provided code, we start by declaring a vector of floats (f_vec
) and a vector of integers (i_vec
). The goal is to convert the floating-point numbers in f_vec
to integers and store them in i_vec
.
To achieve this, we iterate through each element of f_vec
using a range-based for
loop. Inside the loop, we perform the type conversion using a C-style cast: int(f)
.
As we can see in the output, this cast converts the floating-point number f
to its integer representation.
In practice, when possible, it’s better to use more type-safe casting mechanisms provided by C++, such as static_cast
, which provides compile-time type checking and is generally considered safer compared to C-style casting. By utilizing static_cast
, the code becomes more self-explanatory and provides a level of type safety that C-style casting lacks.
We will discuss this further in the next section.
Use static_cast
to Convert Float to Int
As per the modern C++ recommendation, we should utilize a named cast to convert a float
value to an int
.
In order to do this, the static_cast
operator is used for safe and generic type casting in C++. It is used when the type conversion is known to be safe at compile-time and does not involve any pointer conversions.
It converts the types without checking the value; hence, the programmer is responsible for ensuring correctness. Note that named casts and their behavior are quite complicated to grasp with a single overview, so here’s the full manual of static_cast
listing all the edge cases and features.
The syntax for casting a float to an int in C++ using static_cast
is as follows:
int intValue = static_cast<int>(floatValue);
Here, floatValue
is the float variable we want to convert to an int, and intValue
is the resulting integer variable.
Let’s demonstrate this through a complete example:
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<float> f_vec{12.123, 32.23, 534.333333339};
vector<int> i_vec;
i_vec.reserve(f_vec.size());
for (const auto &f : f_vec) {
i_vec.push_back(static_cast<int>(f));
}
for (const auto &i : i_vec) {
cout << i << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
12; 32; 534;
As we can see in the main
function, two vectors are declared: f_vec
, a vector of floats, and i_vec
, an empty vector of integers.
Then, reserve
is called on i_vec
to preallocate memory for i_vec
based on the size of f_vec
. This improves efficiency by reducing the number of reallocations needed as elements are added to i_vec
.
This is followed by a loop that iterates over each element in the f_vec
. For each float f
in f_vec
, it casts f
to an int
using static_cast<int>(f)
and pushes the resulting integer into i_vec
.
This demonstrates the process of converting each float in f_vec
to its integer representation.
Another loop iterates over the elements in i_vec
, printing each integer followed by a semicolon and space. After the loop, endl
is used to insert a new line in the output, providing a clean formatting for the printed integers.
Finally, the main
function returns EXIT_SUCCESS
, indicating successful program execution to the operating system.
Use Rounding Strategies to Convert Float to Int
Rounding Down (Floor)
Rounding down a floating-point number involves truncating the decimal part of the number, effectively moving towards zero. This means that any fractional part of the float is removed, leaving only the whole number part or the integer.
In C++, the floor
function from the <cmath>
library is commonly used to achieve rounding down. It returns the largest integer that is less than or equal to the given float value.
Here’s a simple example demonstrating how to round a float down to the nearest integer:
#include <cmath>
#include <iostream>
int main() {
float floatValue = 4.625;
int intValue = static_cast<int>(std::floor(floatValue));
std::cout << "Rounded down value: " << intValue << std::endl;
return 0;
}
Output:
Rounded down value: 4
In this example, std::floor(floatValue)
rounds the float value down to the nearest whole number (in this case, 4) and then converts it to an integer using static_cast<int>
.
Rounding Up (Ceil)
Rounding up a floating-point number involves finding the smallest integer greater than or equal to the given float. This means any fractional part of the float is rounded up to the nearest whole number.
In C++, the ceil
function from the <cmath>
library is commonly used to achieve rounding up. It returns the smallest integer that is greater than or equal to the given float value.
Here’s an example showing how to round a float up to the nearest integer:
#include <cmath>
#include <iostream>
int main() {
float floatValue = 4.245;
int intValue = static_cast<int>(std::ceil(floatValue));
std::cout << "Rounded up value: " << intValue << std::endl;
return 0;
}
Output:
Rounded up value: 5
Here, std::ceil(floatValue)
rounds the float value up to the nearest whole number (in this case, 5) and then converts it to an integer using static_cast<int>
.
Rounding to the Nearest Integer
Rounding a floating-point number to the nearest integer involves finding the closest whole number to the given float. If the fractional part is less than 0.5, it’s rounded down; if it’s 0.5 or greater, it’s rounded up.
In C++, the round
function from the <cmath>
library is commonly used to achieve rounding to the nearest integer. The round
function returns the nearest integer value to the given float value.
Here’s an example:
#include <cmath>
#include <iostream>
int main() {
float floatValue = 4.5;
int intValue = static_cast<int>(std::round(floatValue));
std::cout << "Rounded to the nearest integer: " << intValue << std::endl;
return 0;
}
Output:
Rounded to the nearest integer: 5
In this example, std::round(floatValue)
rounds the float value to the nearest whole number (in this case, 5) and then converts it to an integer using static_cast<int>
.
Converting a float to an integer in C++ using different rounding strategies, such as rounding down, rounding up, or rounding to the nearest integer, is straightforward using the appropriate functions provided by the <cmath>
library. Choose the appropriate rounding strategy based on the specific requirements of your application.
Conclusion
Converting a float
to an int
in C++ is a fundamental operation frequently encountered in various applications. Each method presented in this article offers a different approach, allowing you to choose based on the specific requirements of your program.
Whether you need truncation, rounding, or controlled rounding, these methods provide you with the flexibility to handle float-to-int conversions effectively. Understanding the implications of each method is essential for precise data manipulation and robust programming in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook