How to Convert Float to String in C++
- Use Macro Expression to Convert a Float to a String in C++
-
Use the
std::to_string()
Method to Convert a Float to a String in C++ -
Use the
std::stringstream
Class and thestr()
Method to Convert a Float to a String in C++ -
Convert a Float to a String in C++ Using
sprintf
-
Convert a Float to a String in C++ Using Boost
lexical_cast
- Conclusion
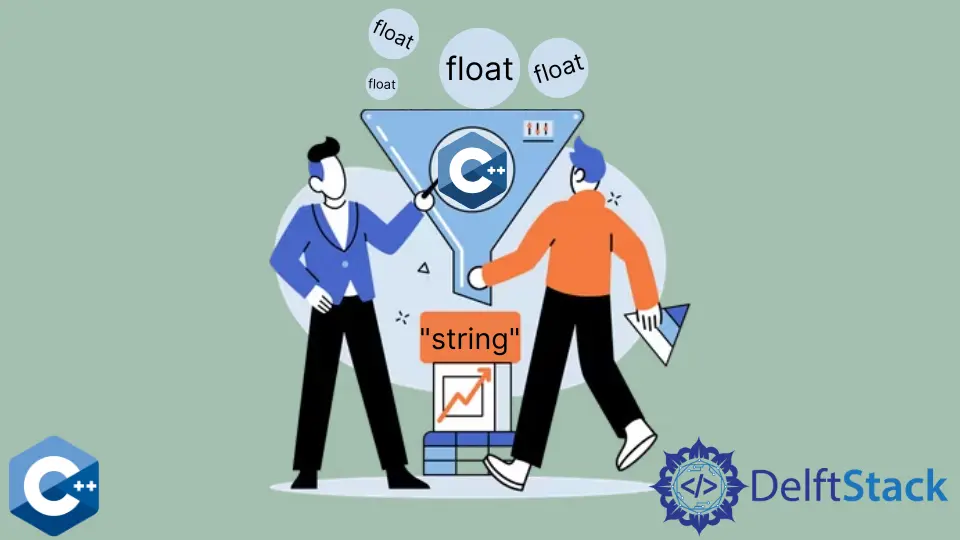
This article introduces several methods of converting a float value to a string in C++.
Use Macro Expression to Convert a Float to a String in C++
While C++ offers standard library functions and features for this purpose, using preprocessor macros can be a convenient and efficient method, especially when you are dealing with constant values that need to be converted at compile time.
Before diving into the conversion process, let’s understand the basics of preprocessor macros.
Macros are essentially placeholders for code snippets that are replaced by their defined values during the compilation process. In C++, macros are defined using the #define
directive.
To create a macro for float-to-string conversion, you can use the stringizing operator #
. This operator transforms a macro argument into a string literal.
#define STRINGIZE(x) #x
Now that we have the basic concept let’s see how we can use macros for converting a float to a string.
#define STRINGIFY(x) #x
int main() {
const char* str = STRINGIFY("Hello, World !");
// str now contains "Hello, World!"
return 0;
}
In the code above, the STRINGIFY
macro takes an argument x
and stringizes it using the #
operator, resulting in "Hello, World!"
. This mechanism is the basis for converting float literals into string representations.
Suppose you have a constant floating-point number, and you want to convert it into a string using preprocessor macros. Here’s how you can do it:
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
#define STRINGIFY_FLOAT(x) #x
int main() {
string num_str = STRINGIFY_FLOAT(3.14159);
cout << "Converted string: " << num_str << endl;
return 0;
}
Output:
Converted string: 3.14159
In this example, the STRINGIFY_FLOAT
macro takes a floating-point literal, 3.14159
, and transforms it into a string "3.14159"
using the #
operator.
This can be especially useful when you want to embed floating-point values in code or configuration files.
But what if your float value is defined as another macro expression? In this case, you’ll need to use a two-level macro approach to ensure the correct conversion. Consider this example:
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
#define STRINGIFY(x) STRINGIFY_HELPER(x)
#define STRINGIFY_HELPER(x) #x
#define MY_FLOAT 2.71828
int main() {
string num_str = STRINGIFY(MY_FLOAT);
cout << "Converted string: " << num_str << endl;
return 0;
}
Output:
Converted string: 2.71828
In this case, we’ve introduced two macros: STRINGIFY
and STRINGIFY_HELPER
. STRINGIFY
takes the float value defined as MY_FLOAT
and passes it to STRINGIFY_HELPER
, which then applies the stringizing operator.
This two-level macro approach ensures that the value is correctly converted to a string.
Using macros for float-to-string conversion, you can’t easily control the precision or formatting of the float value. Macros are primarily used for simple substitutions and stringization.
Suppose you need to control the number of decimal places or apply custom formatting. In that case, it’s recommended to use other methods like std::to_string()
or std::stringstream
, which provide more flexibility in formatting and precision control.
Also, it’s important to note that macros are not well-suited for runtime conversions or scenarios where you need dynamic control over the conversion process.
Use the std::to_string()
Method to Convert a Float to a String in C++
The to_string
function, provided by the C++ standard library and defined in the <string>
header, offers a straightforward and convenient way to convert various numeric types into a std::string
value. This function takes a numeric value as a parameter and returns the equivalent string representation.
To convert a float to a string using std::to_string()
, you can follow these simple steps:
-
Include the necessary header files:
#include <iostream> #include <string>
-
Declare a float variable that you want to convert to a string.
-
Use the
std::to_string()
function to convert the float to a string.
Let’s explore the basics of using the to_string
function for numeric-to-string conversion:
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
float n1 = 123.456;
double n2 = 0.456;
double n3 = 1e-40;
// Convert numeric values to strings
string num_str1 = std::to_string(n1);
string num_str2 = std::to_string(n2);
string num_str3 = std::to_string(n3);
// Display the converted strings
cout << "num_str1: " << num_str1 << endl;
cout << "num_str2: " << num_str2 << endl;
cout << "num_str3: " << num_str3 << endl;
return 0;
}
Output:
num_str1: 123.456001
num_str2: 0.456000
num_str3: 0.000000
In the code above, we have three numeric values, n1
, n2
, and n3
, representing a float
, a double
, and a very small double
, respectively. We use the std::to_string
function to convert these numeric values into string representations, and then we display the results.
One important consideration when using std::to_string
is how it handles precision and significant digits. As shown in the example, std::to_string
may return results with more digits than expected, especially for floating-point values.
For instance, the num_str1
string has more decimal places than the original n1
value. This is because std::to_string
does not truncate or round the floating-point value by default. It retains the full precision of the original number.
If you need more control over the precision of the converted string, you can combine std::to_string
with the <iomanip>
header to set the desired precision using the std::setprecision
manipulator. Here’s an example of how to do that:
#include <iomanip>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
double n = 123.456789;
int precision = 2;
// Convert the number to a string with limited precision
std::ostringstream ss;
ss << std::fixed << std::setprecision(precision) << n;
string num_str = ss.str();
cout << "num_str: " << num_str << endl;
return 0;
}
Output:
num_str: 123.46
In this code, we use std::ostringstream
to format the number with a specified precision of two decimal places. This allows for more control over the number of digits in the resulting string.
The std::to_string
function is a valuable tool for converting numeric values into string representations, and it’s particularly useful when you need a quick and simple conversion for logging, displaying values, or generating strings with numerical data.
However, it’s essential to be aware of the precision behavior and consider using additional formatting mechanisms, as demonstrated with std::setprecision
, when precise control over the number of digits is required. In cases where advanced formatting or custom rounding rules are necessary, other approaches like std::stringstream
can provide greater flexibility.
Use the std::stringstream
Class and the str()
Method to Convert a Float to a String in C++
std::stringstream
is a powerful class that is part of the Standard C++ Library. It combines the functionality of both input and output streams, allowing you to read from and write to string objects as if they were files.
This makes it exceptionally useful for various tasks, including the conversion of numeric values to strings.
To convert a float to a string using std::stringstream
, you’ll follow a few simple steps:
-
Include the necessary header files:
#include <iostream> #include <sstream> #include <string>
-
Create an instance of
std::stringstream
. -
Use the
<<
operator to insert the float value into thestd::stringstream
. -
Retrieve the string representation of the float using the
str()
method of thestd::stringstream
.
Let’s delve into the basics of using std::stringstream
to convert a floating-point value into a string:
#include <iostream>
#include <sstream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
float n1 = 123.456;
std::stringstream sstream;
sstream << n1; // Insert the floating-point value into the stringstream
string num_str =
sstream.str(); // Extract the string representation from the stringstream
// Display the converted string
cout << "Converted string: " << num_str << endl;
return 0;
}
Output:
Converted string: 123.456
In this example, we create a std::stringstream
object named sstream
and use the insertion (<<
) operator to place the n1
floating-point value into the stream. Next, we retrieve the resulting string representation using the str()
method and store it in the num_str
variable.
It’s crucial to understand the behavior of temporary objects when working with std::stringstream
. In the provided code, you may notice a commented-out line that attempts to retrieve the C-style string pointer from the temporary string returned by sstream.str()
.
However, this operation results in a dangling pointer because the temporary string goes out of scope, leaving ptr
pointing to invalid memory.
Here’s the commented line for reference:
auto *ptr = sstream.str().c_str(); // RESULTS in dangling pointer
To avoid such issues, always make sure to store the result of sstream.str()
in a named string, as demonstrated in the main example.
One of the advantages of using std::stringstream
is the ability to control the precision and formatting of the converted string. You can manipulate the stream’s properties using manipulators like std::fixed
, std::scientific
, and std::setprecision
to format the output as needed.
For instance, you can display the float with a specific number of decimal places like this:
ss << std::fixed << std::setprecision(2) << floatValue;
This code will format the float with two decimal places in the resulting string.
Convert a Float to a String in C++ Using sprintf
Another way to convert a floating-point number to a string is by using the sprintf
function. sprintf
is part of the C Standard Library and is available in C++ as well. It provides a means to format and convert data into strings with a high degree of control over the formatting.
sprintf
takes a character array (C-string) and a format string as arguments. It then formats and converts the data according to the format string and stores the result as a null-terminated string in the character array.
This makes it a valuable tool for customizing the appearance of numeric values when converting them to strings.
Let’s take a look at how to use sprintf
to convert a float to a string:
#include <cstdio> // Include the C Standard Library for sprintf
#include <iostream>
int main() {
float floatValue = 3.14159;
char buffer[50]; // Create a character array to store the result
// Use sprintf to format and convert the float to a string
sprintf(buffer, "%.2f", floatValue); // "%.2f" specifies the format
// The buffer now contains the string representation of the float
std::cout << "Converted string: " << buffer << std::endl;
return 0;
}
Output:
Converted string: 3.14
In the example above, we start by including the <cstdio>
header, which provides the sprintf
function. We then create a character array called buffer
to store the resulting string.
The sprintf
function is used to format the floatValue
with the format string "%.2f"
, which specifies that the float should be displayed with two decimal places.
The formatted string is stored in the buffer
, and we can subsequently use it as needed.
The format string in sprintf
allows you to specify the precision, field width, and other formatting details for the output string. In the example above, "%.2f"
was used to display the float with two decimal places.
You can adjust the format string to meet your specific requirements.
It’s important to note that sprintf
does not perform any bounds checking on the destination buffer. If the formatted string is longer than the buffer can hold, it can lead to buffer overflows, which can be a security risk.
In order to mitigate this risk, it’s recommended to use the safer snprintf
function in modern C and C++ programming. snprintf
allows you to specify the size of the destination buffer and ensures that no more characters are written than the buffer can hold.
sprintf
is a suitable choice when you need precise control over the formatting of floating-point values when converting them to strings. It’s especially useful when dealing with numeric data that requires custom formatting, such as currency amounts, measurements, or scientific notation.
However, suppose safety is a concern, or you need more flexibility in handling different data types. In that case, C++ provides other options, such as the std::stringstream
for string conversion or the std::to_string
function for simple conversions.
These alternatives can be more suitable for general-purpose string conversions and dynamic scenarios.
Convert a Float to a String in C++ Using Boost lexical_cast
Boost lexical_cast
is a part of the Boost C++ Libraries, a collection of high-quality libraries that enhance and extend C++. The lexical_cast
function template allows you to perform type-safe conversions between a wide range of data types and their string representations.
It’s a handy utility for simplifying conversions and ensuring robust error handling in C++.
To convert a float to a string using Boost lexical_cast
, you need to include the necessary headers and make use of the boost::lexical_cast
function template. Here’s a step-by-step example:
-
Include the Boost
lexical_cast
header:#include <boost/lexical_cast.hpp>
-
Use
boost::lexical_cast
to convert a float to a string:float floatValue = 3.14159; std::string strValue = boost::lexical_cast<std::string>(floatValue);
Here’s the complete code example:
#include <boost/lexical_cast.hpp>
#include <iostream>
#include <string>
int main() {
float floatValue = 3.14159;
try {
std::string strValue = boost::lexical_cast<std::string>(floatValue);
std::cout << "Converted string: " << strValue << std::endl;
} catch (const boost::bad_lexical_cast& e) {
std::cerr << "Conversion failed: " << e.what() << std::endl;
}
return 0;
}
Output:
Converted string: 3.14159
In this example, we start by including the <boost/lexical_cast.hpp>
header, which provides access to the boost::lexical_cast
function. We then declare a float floatValue
and use boost::lexical_cast
to convert it into a string, storing the result in strValue
.
This conversion is wrapped in a try-catch
block to handle exceptions. The boost::bad_lexical_cast
exception is caught if the conversion fails.
One of the advantages of using Boost lexical_cast
is its built-in exception handling. If the conversion encounters an error, it throws a boost::bad_lexical_cast
exception.
This allows you to handle conversion failures gracefully by catching the exception and taking appropriate action, as demonstrated in the code above.
Boost lexical_cast
is a convenient choice when you need to convert a float to a string while maintaining type safety and robust error handling. It’s especially valuable when working with multiple data types and ensuring that conversions are performed safely and reliably.
However, if you need more complex formatting options or want to perform other types of conversions, you may consider alternatives such as std::to_string
or std::stringstream
.
Conclusion
In this article, we’ve examined various techniques for converting float values to strings in C++.
Preprocessor macros offer a straightforward way to perform simple conversions, particularly for constant values. However, they lack flexibility in precision control and are best suited for compile-time conversions.
The std::to_string()
function provides a convenient and safe method for converting float values to strings. It is suitable for most use cases, with the ability to control precision using manipulators like std::setprecision
.
For more advanced formatting and precision control, the std::stringstream
class is a powerful tool that combines ease of use with flexibility. It allows for customized formatting and precision settings, making it a valuable choice for various string conversion scenarios.
Additionally, Boost lexical_cast
offers type-safe conversions with built-in exception handling, ensuring reliable and error-tolerant conversions. It’s particularly useful when working with a variety of data types and requiring robust error management.
Ultimately, the choice of method depends on the specific requirements of your project. Whether you need a simple, efficient conversion or more advanced control over precision and error handling, C++ provides a range of options to suit your needs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook