How to Generate a Random Number Between 0 and 1 in C++
-
Use the
rand()
Function to Generate a Random Number Between0
and1
in C++ -
Use the C++11
<random>
Library to Generate a Random Number Between0
and1
-
Use Only
std::uniform_real_distribution()
to Generate Random Numbers Between0
and1
in C++ -
Use the
std::rand
Function to Generate a Random Float Between0
and1
in C++ - Conclusion
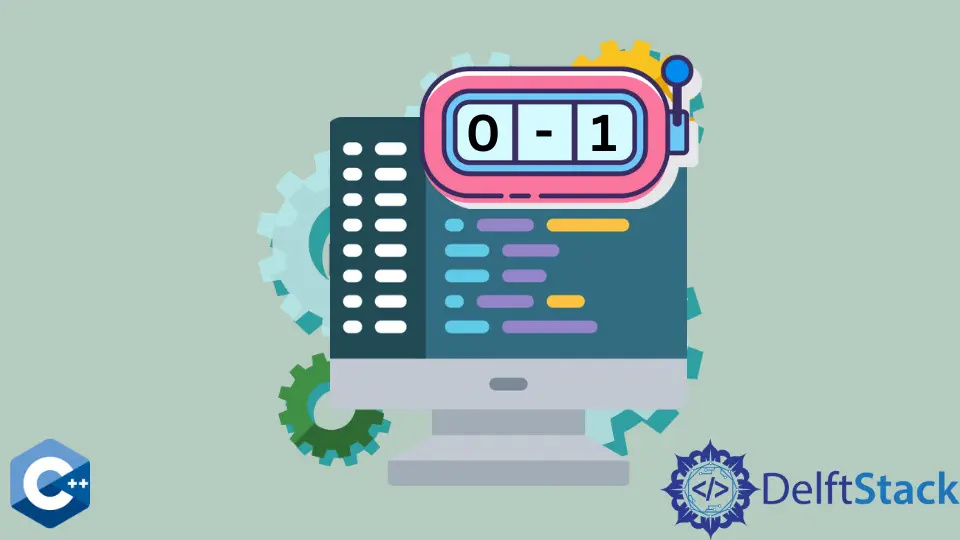
Random numbers are fundamental in learning the concept of programming, as they are often used in various applications, being useful in a wide range from simulations to gaming.
When it comes to generating random numbers between 0
and 1
in C++, developers have several methods at their disposal. Each method has its advantages and suitability for different scenarios.
In this comprehensive guide, we will explore all available methods for generating random numbers in the range [0, 1]
in C++, accompanied by detailed explanations, examples, and a conclusion containing the comparative analysis of all the available methods to help you choose the best approach for your programming needs.
Use the rand()
Function to Generate a Random Number Between 0
and 1
in C++
As the name suggests, the rand()
function is utilized to generate and display any random number with or without a given range in C++. Here, we use a term called RAND_MAX
that is made to be the upper limit of the range [0, RAND_MAX]
in the function, which can have a varied value based on certain implementations, but it is usually above the 32767
value.
The random
library needs to be imported in order to make use of this function.
The syntax for this function is as follows:
int rand(void);
The rand()
function doesn’t necessarily need any parameters, and it returns a pseudo-random number that is always in the range of 0
and RAND_MAX
.
Considering the topic for our tutorial is a random number between 0
and 1
, the following code implements the task at hand.
#include <iostream>
#include <random>
using namespace std;
int main() {
for (int x = 0; x < 5; ++x) {
cout << (float)rand() / (float)RAND_MAX << '\n';
}
}
The above code uses the rand()
function along with a for
loop to generate 5 random numbers between 0
and 1
.
Output:
As we see in the output above, the rand()
function is utilized to generate 5 different random numbers between 0
and 1
, with each of the values differing from one another, giving us a little glimpse of the efficiency of the function.
It is important to note the fact that whenever we run this particular piece of code, we will always get the same 5 random numbers. To get more variety, you can always include the srand()
function, with which we can specify the starting point of the range.
Use the C++11 <random>
Library to Generate a Random Number Between 0
and 1
The C++ version 11 provides classes/methods for random and pseudo-random number generation. These standard library facilities are recommended for handling random number generation in the modern C++ codebase.
The std::random_device
object is initialized first; it’s a non-deterministic uniform random bit generator used to seed the random number engine std::default_random_engine
, which is initialized on the next line. This way, it’s ensured that the engine won’t produce the same number of sequences.
There are more than a handful of random number engines that are implemented in the C++ <random>
library, and they differ from each other in a few factors like time/space requirements and much more (see the full list here).
In the following example below, we demonstrate random float generation using std::default_random_engine
. We can specify a certain algorithm engine as required by the application constraints.
The next step is we initialize a uniform distribution and pass min/max values as arguments. Finally, we print 5 random floating-point values in [0-1]
intervals to the console.
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
constexpr int FLOAT_MIN = 0;
constexpr int FLOAT_MAX = 1;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_real_distribution<float> distr(FLOAT_MIN, FLOAT_MAX);
for (int x = 0; x < 5; ++x) {
cout << setprecision(6) << distr(eng) << "\n";
}
return EXIT_SUCCESS;
}
Note: The
std::uniform_real_distribution< T >
yields undefined behavior if one of the following types is not passed as a template parameter:float
,double
, orlong double
.
The above code makes use of a random number engine from the C++11 <random>
library to generate a set of random numbers.
Output:
The output above displays the set of random numbers generated with the help of the engine from the C++11 <random>
library.
Use Only std::uniform_real_distribution()
to Generate Random Numbers Between 0
and 1
in C++
The uniform_real_distribution
function can help generate floating-point random numbers while adhering to a uniform distribution pattern. This uniform distribution pattern adheres to the probability density function.
The syntax:
template <class RealType = double>
class uniform_real_distribution;
Here, the template parameter RealType
refers to the type of result generated with the help of the generator. Note that the effect remains undefined if it is not a float
, long double
, or double
.
We can make use of it as a standalone function along with a random number generator.
Consider the following code:
#include <iostream>
#include <random>
using namespace std;
int main() {
random_device rd;
mt19937 fort(rd());
uniform_real_distribution<> apex(0, 1.0);
for (int i = 0; i < 5; ++i) {
cout << apex(fort) << '\n';
}
}
Here, we use the real_uniform_distribution
function along with the very efficient std:mt19937
, which is a pseudo-random number generator. We should also note that this method is only applicable in C++ 11 and above versions.
Output:
As we see in the output above, we successfully get 5 random numbers between 0
and 1
with the help of the uniform_real_distribution()
function along with the mt19937
pseudo-random number generator.
Use the std::rand
Function to Generate a Random Float Between 0
and 1
in C++
The rand
function is a part of the C standard library random number generation facilities. It’s not recommended for applications requiring high-quality randomness.
Still, it can be utilized for testing purposes or cryptographically non-sensitive number generation.
It has the following syntax:
int rand(); // the <cstdlib> should be defined in the header
The function itself contains no parameters, and the return value is a pseudo-random integral value.
The rand
function generates a pseudo-random integer between 0
and RAND_MAX
(both included). Since the RAND_MAX
value is implementation-dependent, and the guaranteed minimum value is only 32767
, generated numbers have constrained randomness.
Keep in mind that this function must be seeded with std::srand
(preferably passing the current time using std::time
). Finally, we can generate floating-point values in the interval of [0-1]
.
Let us look at an example code to get a better understanding.
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
constexpr int FLOAT_MIN = 0;
constexpr int FLOAT_MAX = 1;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < 5; i++)
cout << setprecision(6)
<< FLOAT_MIN +
(float)(rand()) / ((float)(RAND_MAX / (FLOAT_MAX - FLOAT_MIN)))
<< endl;
return EXIT_SUCCESS;
}
The above code makes use of the std::rand()
function to generate a set of 5 random numbers in the range of [0,1]
.
Output:
The output above displays a set of 5 random numbers that are between the range of 0
and 1
as specified.
Conclusion
C++ offers various methods for generating random numbers between 0
and 1
, each with its advantages.
While the traditional rand()
method can serve basic needs, C++11’s <random>
library provides more robust and flexible options. If you’re using C++17 or later, the <random>
header simplifies the process even further.
Consider factors like randomness quality, performance, and library availability when selecting the best method for generating random numbers in the [0, 1]
range. Understanding the strengths of each approach will enable you to make informed decisions that enhance the reliability and effectiveness of your C++ applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook