如何在 C++ 中打印指定小数点的数字
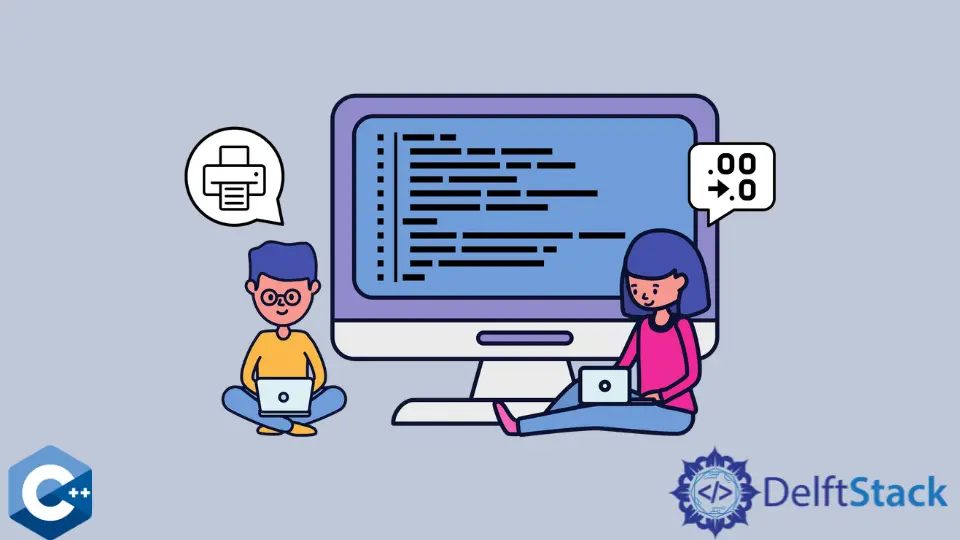
本文将介绍如何在 C++ 中打印指定小数点的数字。
使用 std::fixed
和 std::setprecision
方法指定精度
本方法使用 <iomanip>
头文件中定义的标准库中的所谓 I/O 操作器(查看完整列表)。这些函数可以修改流数据,主要用于 I/O 格式化操作。在第一个例子中,我们只使用 fixed
方法,它修改了默认的格式,使浮点数用定点符号写入。注意,结果总是有一个预定义的小数点后 6 位精度。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << fixed << d << endl;
}
return EXIT_SUCCESS;
}
输出:
123.231000
2.234300
0.324000
10.222424
6.349109
110.123294
92.001112
0.000000
另外,我们也可以将小数点固定在逗号之后,同时指定精度值。在下一个例子中,fixed
函数与 setprecision
函数一起调用,后者本身使用 int
值作为参数。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << fixed << setprecision(3) << d << endl;
}
return EXIT_SUCCESS;
}
输出:
123.231
2.234
0.324
10.222
6.349
110.123
92.001
0.000
前面的代码示例解决了最初的问题,但输出的结果似乎很混杂,而且几乎没有可读的格式。我们可以使用 std::setw
和 std::setfill
函数来显示给定向量中的浮点数,并将其对齐到同一个逗号位置。为了达到这个目的,我们应该调用 setw
函数来告诉 cout
每个输出的最大长度(本例中我们选择 8)。接下来,调用 setfill
函数,指定一个 char
来填充填充位。最后,我们添加之前使用的方法(fixed
和 setprecision
),并输出到控制台。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setfill;
using std::setprecision;
using std::setw;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << setw(8) << setfill(' ') << fixed << setprecision(3) << d << endl;
}
return EXIT_SUCCESS;
}
输出:
123.231
2.234
0.324
10.222
6.349
110.123
92.001
0.000
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu