How to Loop Over an Array in C++
-
Using a
for
Loop -
Using a
while
Loop -
Using a Range-Based
for
Loop -
Use
std::for_each
Algorithm to Iterate Over an Array - Conclusion
- FAQ
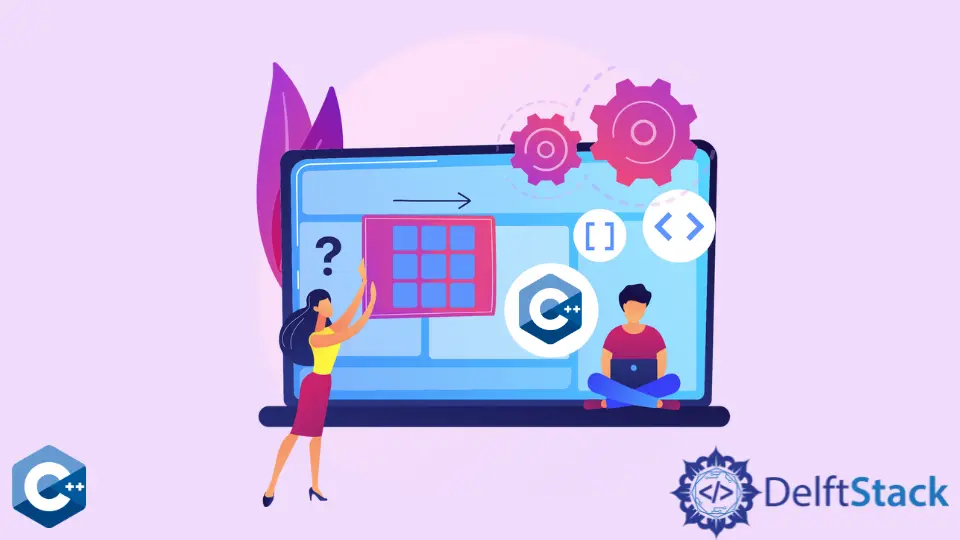
When it comes to programming in C++, one of the fundamental tasks you will often encounter is iterating over an array. Whether you’re processing data, performing calculations, or simply displaying values, knowing how to effectively loop through an array is crucial.
In this article, we will explore various methods to iterate over arrays in C++, providing clear examples and explanations for each approach. By the end of this guide, you will be equipped with the knowledge to choose the right looping technique for your needs, enhancing your coding skills and efficiency.
Using a for
Loop
One of the most common ways to loop through an array in C++ is by using a traditional for loop. This method gives you complete control over the iteration process, allowing you to specify the starting point, the end condition, and the increment for each iteration.
Here’s how you can implement a for loop to iterate over an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
int size = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output:
10 20 30 40 50
In this example, we first declare an integer array named arr
and initialize it with five values. We then calculate the size of the array by dividing the total size of the array by the size of its first element. The for loop runs from 0
to size - 1
, accessing each element of the array using the index i
. The values are printed in a single line, separated by spaces. This method is straightforward and efficient, making it a popular choice for looping through arrays.
Using a while
Loop
Another way to iterate over an array is by using a while
loop. This approach can be particularly useful when you want to loop until a certain condition is met, rather than relying on a counter.
Here’s an example of how to use a while
loop to iterate over an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {5, 15, 25, 35, 45};
int size = sizeof(arr) / sizeof(arr[0]);
int i = 0;
while (i < size) {
cout << arr[i] << " ";
i++;
}
return 0;
}
Output:
5 15 25 35 45
In this code snippet, we initialize i
to 0
before entering the while
loop. The loop continues as long as i
is less than the size of the array. Inside the loop, we print the current element and then increment i
by 1
. This method provides flexibility, allowing for more complex conditions to be implemented if necessary. while
loops can be particularly useful when the number of iterations is not predetermined.
Using a Range-Based for
Loop
C++11 introduced a more concise way to loop through arrays using the range-based for loop. This method simplifies the syntax, making your code cleaner and easier to read.
Here’s how to use a range-based for loop:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
for (int value : arr) {
cout << value << " ";
}
return 0;
}
Output:
1 2 3 4 5
In this example, we declare an integer array arr
and use a range-based for loop to iterate through its elements. Instead of managing the index manually, we simply declare a variable value
that takes on each element of the array in turn. This method is not only more readable but also less error-prone, as it reduces the chances of off-by-one errors and makes the code cleaner. Range-based for loops are particularly advantageous when working with larger arrays or when writing code that prioritizes readability.
Use std::for_each
Algorithm to Iterate Over an Array
for_each
is a powerful STL algorithm to operate on range elements and apply custom defined functions. It takes range starting and last iterator objects as the first two parameters, and the function object as the third one. In this case, we declare a function object as lambda
expression, which directly outputs the calculated results to the console. Lastly, we can pass the custom_func
variable as an argument to for_each
method to operate on array elements.
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::for_each;
using std::string;
int main() {
array<int, 10> int_arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto custom_func = [](auto &i) { cout << i * (i + i) << "; "; };
;
for_each(int_arr.begin(), int_arr.end(), custom_func);
cout << endl;
return EXIT_SUCCESS;
}
Output:
2; 8; 18; 32; 50; 72; 98; 128; 162; 200;
This code demonstrates the use of the std::for_each
algorithm from the C++ Standard Template Library (STL) to process elements in an array. It creates an std::array
of integers and defines a lambda function custom_func
that multiplies each element by twice its value and prints the result.
The for_each
function is then used to apply this custom function to every element in the array. This approach showcases the power and flexibility of STL algorithms combined with modern C++ features like lambda expressions. It provides a concise and efficient way to perform operations on container elements without explicit loop constructs, enhancing code readability and potentially allowing for better optimization by the compiler.
Conclusion
Looping over arrays in C++ is a fundamental skill that every programmer should master. Whether you choose a traditional for loop, a while
loop, or a range-based for loop, each method has its own advantages depending on the context of your code. By understanding these different techniques, you can write more efficient and readable code, making your programming journey smoother and more enjoyable. Practice these methods and see how they can fit into your projects, enhancing your coding repertoire.
FAQ
-
What is the difference between a for loop and a
while
loop in C++?
A for loop is typically used when the number of iterations is known, while awhile
loop is better for scenarios where the number of iterations is not predetermined. -
Can I use a range-based for loop with other data structures?
Yes, range-based for loops can be used with any container that supports iteration, such as vectors and lists. -
Are there any performance differences between these looping methods?
Generally, the performance differences are negligible for small arrays. However, for very large datasets, the choice of loop may affect readability and maintainability more than performance. -
Is it safe to access array elements using an index?
You should always ensure that the index is within the bounds of the array to avoid undefined behavior or runtime errors. -
Can I modify the elements of an array
while
looping through it?
Yes, you can modify elements in the array directly within the loop, but be cautious of how it may affect the loop’s logic.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook