How to Initialize a Vector in C++
- What is a Vector in C++?
- Method 1: Initializing a Vector with a Specific Size
- Method 2: Initializing a Vector with Specific Values
- Method 3: Initializing a Vector from Another Vector
- Method 4: Initializing a Vector with a Fill Value
- Conclusion
- FAQ
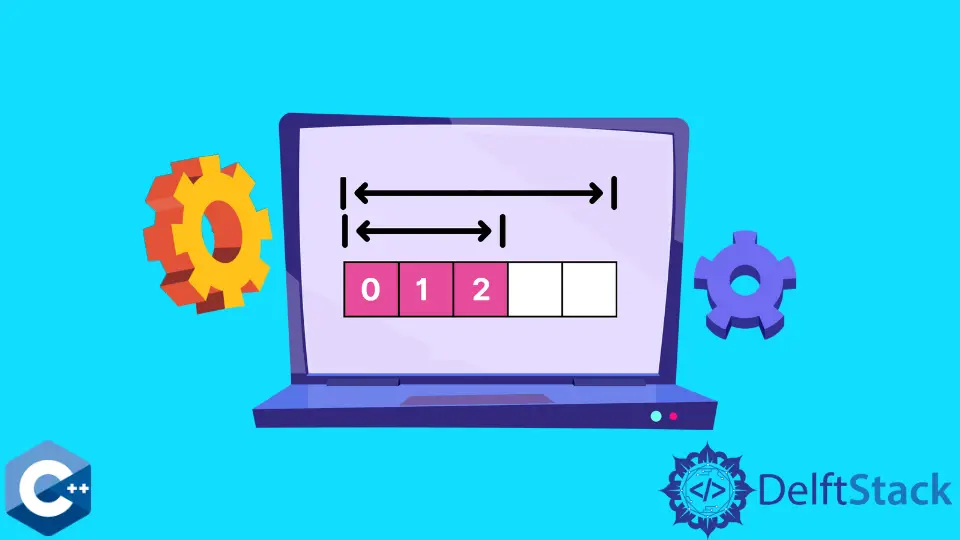
When it comes to programming in C++, one of the most versatile and widely used data structures is the vector. Vectors provide a dynamic array that can resize itself automatically when elements are added or removed. However, initializing a vector correctly is crucial for effective memory management and performance.
In this article, we will explore various methods to initialize a vector in C++. Whether you’re a beginner or an experienced developer, understanding these techniques will enhance your coding skills and efficiency. Let’s dive into the world of vectors and discover how to initialize them in C++.
What is a Vector in C++?
Before we delve into the initialization methods, it’s essential to understand what a vector is. A vector in C++ is part of the Standard Template Library (STL) and is defined in the <vector>
header. Unlike traditional arrays, vectors can grow and shrink in size dynamically, making them ideal for situations where the number of elements isn’t known in advance. They are implemented as dynamic arrays, allowing for random access to elements and automatic memory management.
Method 1: Initializing a Vector with a Specific Size
One of the simplest ways to initialize a vector in C++ is by specifying its size during declaration. This method creates a vector with a predefined number of elements, all initialized to a default value (typically zero for numeric types).
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec(5); // Initializes a vector of size 5
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
Output:
0 0 0 0 0
In this example, we declare a vector named vec
with a size of 5. Since we did not specify any initial values, all elements are initialized to zero. This method is particularly useful when you know the number of elements you will need in advance. Using this approach allows for efficient memory allocation right from the start.
Method 2: Initializing a Vector with Specific Values
Another common method for initializing a vector is to provide specific values at the time of declaration. This method allows you to create a vector that is pre-filled with data, making it easier to work with predefined lists.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5}; // Initializes a vector with specific values
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
Output:
1 2 3 4 5
In this example, we initialize the vector vec
with specific integer values. This method is particularly useful when you have a known set of data that you want to work with immediately. By using an initializer list, you can easily create a fully populated vector in a single line of code, improving readability and maintainability.
Method 3: Initializing a Vector from Another Vector
You can also initialize a vector using another vector. This method is helpful when you want to create a copy of an existing vector or when you want to create a new vector that shares the same values as an existing one.
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {10, 20, 30}; // Original vector
std::vector<int> copy(original); // Initializes a new vector from the original
for (int i : copy) {
std::cout << i << " ";
}
return 0;
}
Output:
10 20 30
In this case, we first create an original
vector with specific values. Then, we initialize a new vector named copy
using the original
vector. This method is particularly useful for creating duplicates or when you want to manipulate a copy without affecting the original data. The copy constructor of the vector class makes this operation efficient and straightforward.
Method 4: Initializing a Vector with a Fill Value
If you want to initialize a vector with a specific size and fill it with a particular value, you can use the fill constructor. This method allows you to create a vector of a given size, where each element is initialized to the specified value.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec(5, 100); // Initializes a vector of size 5, each element set to 100
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
Output:
100 100 100 100 100
In this example, we create a vector of size 5, and every element is initialized to 100. This method is particularly useful when you need a vector of a specific size with a common initial value, such as when setting up a grid or a matrix in your application. It simplifies the initialization process and makes your code cleaner.
Conclusion
Understanding how to initialize a vector in C++ is vital for any programmer looking to leverage the power of dynamic arrays. From creating a vector of a specific size to filling it with values or copying from another vector, each method has its unique advantages. By mastering these techniques, you can enhance your coding efficiency and write cleaner, more maintainable code. Whether you’re working on a small project or a large software application, knowing how to initialize vectors effectively will serve you well in your C++ programming journey.
FAQ
-
What is a vector in C++?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. -
How do I initialize a vector with specific values?
You can initialize a vector with specific values using an initializer list, like this:std::vector<int> vec = {1, 2, 3};
. -
Can I initialize a vector from another vector?
Yes, you can create a new vector using another vector as a source, like this:std::vector<int> copy(original);
. -
What happens if I don’t specify a fill value when initializing a vector?
If you don’t specify a fill value, the vector will be initialized with default values (typically zeros for numeric types).
- Are vectors in C++ the same as arrays?
No, vectors are dynamic and can resize themselves, whereas arrays have a fixed size once defined.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook