Inizializza un vettore in C++
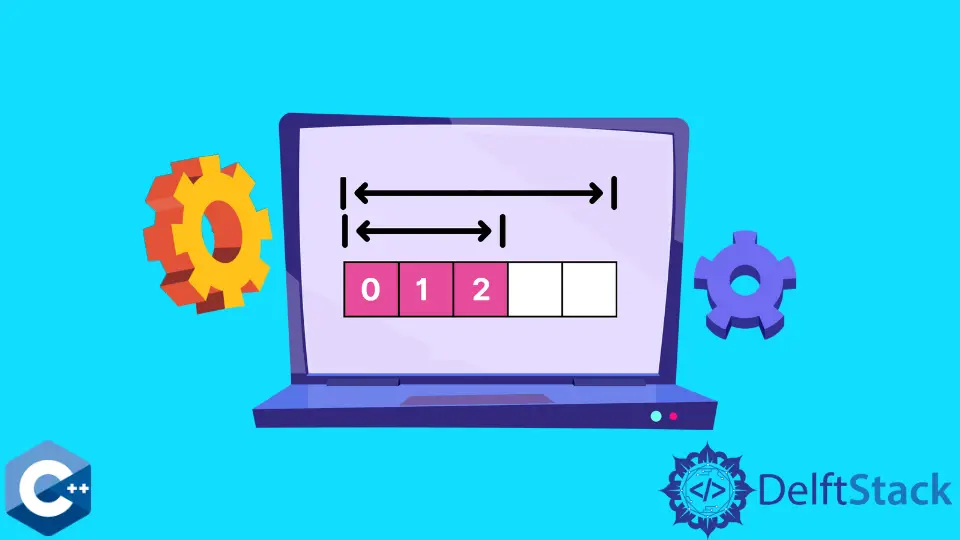
Questo articolo spiegherà come inizializzare un vettore con valori costanti in C++.
Usa la notazione dell’lista di inizializzatori per assegnare valori costanti agli elementi Vector
in C++
Questo metodo è stato supportato dallo stile C++ 11 ed è relativamente il modo più leggibile per inizializzare costantemente la variabile vector
. I valori sono specificati come una lista di inizializzazione tra parentesi
che è preceduta dall’operatore di assegnazione. Questa notazione è spesso chiamata inizializzazione dell’lista di copie.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> arr = {"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
Produzione:
one; two; three; four;
Il metodo alternativo consiste nell’usare la chiamata del costruttore dell’lista di inizializzatori quando si dichiara la variabile vector
come mostrato nel seguente blocco di codice:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> arr{"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
Una macro del preprocessore è un altro modo per implementare la notazione dell’lista di inizializzatori. In questo esempio, definiamo i valori degli elementi costanti come macro tipo oggetto INIT
e poi lo assegniamo alla variabile vector
come segue:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
#define INIT \
{ "one", "two", "three", "four" }
int main() {
vector<string> arr = INIT;
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
La sintassi dell’lista di inizializzatori C++ 11 è uno strumento abbastanza potente e può essere utilizzato per istanziare un vector
di strutture complesse come dimostrato nel seguente esempio di codice:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct cpu {
string company;
string uarch;
int year;
} typedef cpu;
int main() {
vector<cpu> arr = {{"Intel", "Willow Cove", 2020},
{"Arm", "Poseidon", 2021},
{"Apple", "Vortex", 2018},
{"Marvell", "Triton", 2020}};
for (const auto &item : arr) {
cout << item.company << " - " << item.uarch << " - " << item.year << endl;
}
return EXIT_SUCCESS;
}
Produzione:
Intel - Willow Cove - 2020
Arm - Poseidon - 2021
Apple - Vortex - 2018
Marvell - Triton - 2020
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook