How to Declare 2D Array Using new in C++
- Understanding Dynamic Memory Allocation
-
Declare 2D Array to Access Elements With
arr[x][y]
Notation -
Declare 2D Array to Access Elements With
arr[]
Notation -
Use
vector
Container to Implicitly Allocate Dynamic 2D Array - Important Considerations
- Conclusion
- FAQ
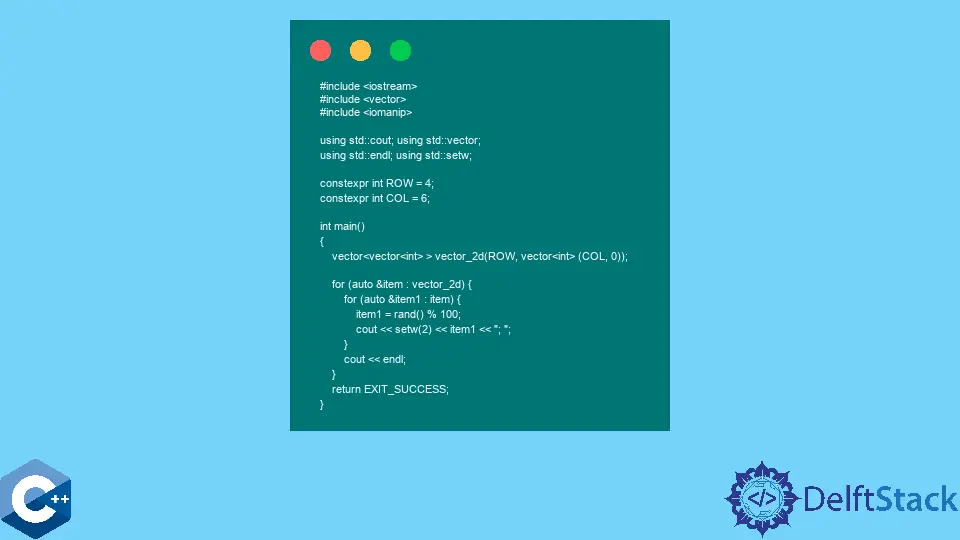
When it comes to programming in C++, managing data structures efficiently is crucial. One common requirement is declaring and using 2D arrays. Unlike static arrays, using dynamic memory allocation with the new
operator allows for greater flexibility and control over memory usage.
This article will guide you through the methods of declaring 2D arrays using new
in C++, providing clear examples and explanations to help you understand the process better. Whether you’re a beginner or looking to refine your skills, this comprehensive guide will equip you with the knowledge you need.
Understanding Dynamic Memory Allocation
Dynamic memory allocation in C++ is a powerful feature that allows you to allocate memory at runtime. This is particularly useful when you don’t know the size of your data structures in advance. The new
operator is used for this purpose, enabling you to create arrays whose size can be determined during program execution.
When declaring a 2D array using new
, you essentially create an array of pointers, where each pointer points to a dynamically allocated array. This method provides greater flexibility, especially when working with large datasets or when memory needs to be managed efficiently.
Declare 2D Array to Access Elements With arr[x][y]
Notation
This solution utilizes new
keyword so that the generated matrix structure can be accessed using array notation - [x][y]
. At first, we declare pointer to pointer to integer (int **
) variable and allocate int
pointer array of row size in the array. Next, we loop over this pointer array and allocate the int
array of column size each iteration. Lastly, when we finish the 2D array operation, we need to free up the allocated memory. Notice, that deallocation is done in the reverse order loop.
#include <iomanip>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
int **matrix = new int *[ROW];
for (int i = 0; i < ROW; ++i) {
matrix[i] = new int[COL];
}
for (int i = 0; i < ROW; ++i) {
for (int j = 0; j < COL; ++j) {
matrix[i][j] = rand() % 100;
cout << setw(2) << matrix[i][j] << "; ";
}
cout << endl;
}
for (int i = 0; i < ROW; ++i) {
delete matrix[i];
}
delete[] matrix;
return EXIT_SUCCESS;
}
Output:
83; 86; 77; 15; 93; 35;
86; 92; 49; 21; 62; 27;
90; 59; 63; 26; 40; 26;
72; 36; 11; 68; 67; 29;
Declare 2D Array to Access Elements With arr[]
Notation
This method uses a single array allocation, making it a more efficient method in terms of program execution speed. Consequently, we should use the []
notation and some arithmetic to access the elements. This method is the recommended way when the array size gets bigger, and calculations on its elements are quite intensive. Note also that deallocation becomes easier this way.
#include <iomanip>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
int *matrix = new int[ROW * COL];
for (int i = 0; i < ROW; ++i) {
for (int j = 0; j < COL; ++j) {
matrix[j * ROW + i] = rand() % 100;
cout << setw(2) << matrix[j * ROW + i] << "; ";
}
cout << endl;
}
delete[] matrix;
return EXIT_SUCCESS;
}
Use vector
Container to Implicitly Allocate Dynamic 2D Array
Alternatively, we can construct a dynamic 2D array using a vector
container and without manual memory allocation/deallocation. In this example, we initialize a 4x6 vector_2d
array with each element of the value - 0
. The best part of this method is the flexibility of the iteration with the range-based loop.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
vector<vector<int> > vector_2d(ROW, vector<int>(COL, 0));
for (auto &item : vector_2d) {
for (auto &item1 : item) {
item1 = rand() % 100;
cout << setw(2) << item1 << "; ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Important Considerations
When working with dynamic memory in C++, there are a few important considerations to keep in mind. First and foremost is memory management. Always ensure that you deallocate any memory that you allocate with new
using delete[]
. Failing to do so can lead to memory leaks, which can degrade performance over time.
Additionally, be cautious when accessing elements in your 2D array. Ensure that your indices are within bounds to avoid undefined behavior. Implementing checks or using assert statements can help catch potential errors early.
Lastly, consider the performance implications of using dynamic memory allocation. While it offers flexibility, it may introduce overhead compared to static arrays. Always weigh the benefits against the potential performance costs based on your application’s needs.
Conclusion
Declaring a 2D array using new
in C++ is a valuable skill that enhances your ability to manage data structures dynamically. By understanding how to allocate and deallocate memory properly, you can create flexible and efficient applications. Remember to always manage memory carefully and keep performance considerations in mind. With these techniques in your toolkit, you’ll be well-equipped to tackle a variety of programming challenges.
FAQ
-
How do I declare a 2D array without using
new
?
You can declare a 2D array statically using the syntaxint array[rows][cols];
. -
What happens if I forget to deallocate memory?
Failing to deallocate memory can lead to memory leaks, which may cause your program to consume more memory over time. -
Can I create a 2D array with varying row sizes?
Yes, you can create an array of pointers where each pointer points to a different-sized array, allowing for jagged arrays. -
Is it safe to access elements outside the bounds of the array?
No, accessing elements outside the bounds of the array can lead to undefined behavior and potential crashes. -
What is the difference between
new
andmalloc
?
new
initializes objects and calls constructors, whilemalloc
simply allocates raw memory without any initialization.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook