How to Copy an Array in C++
-
Use the
for
Loop to Implement Manual Deep Copying in C++ - Use the Copy Constructor to Copy a C++ Array
-
Use the
copy()
Function to Copy an Array in C++ -
Use the
copy_backward()
Function to Copy an Array -
Use the
assign()
Method to Copy an Array -
Use the
std::memcpy
Function to Copy a C++ Array - Conclusion
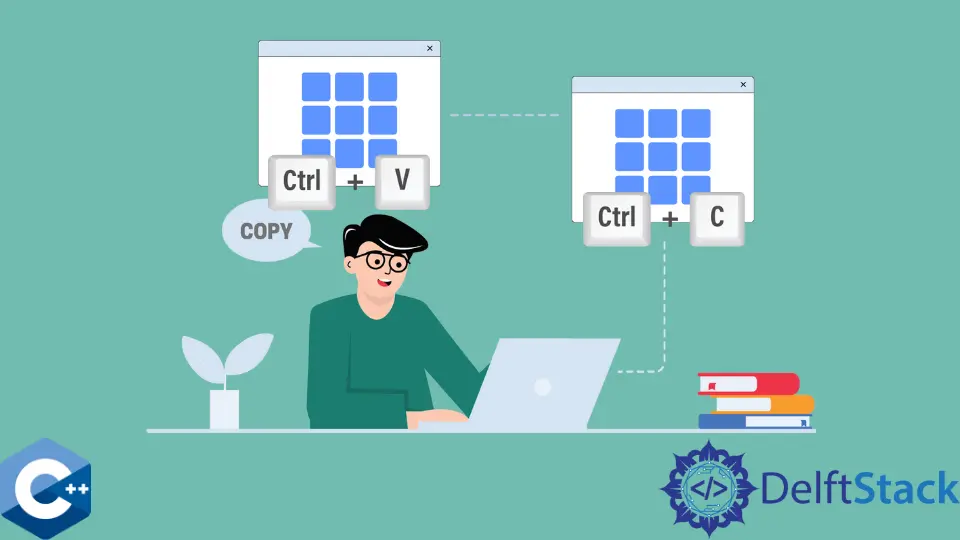
In C++, working with arrays is a core aspect of programming and can be said to be essential when it comes to understanding programming. Whether you’re dealing with small arrays or complex multidimensional structures, the need to copy arrays arises frequently.
Copying an array allows you to manipulate data without needing to alter the original, making it a crucial skill for every developer.
In this article, we will delve into various methods for copying arrays in C++. We will explore making use of standard library functions, user-defined functions, and modern techniques like std::copy
.
By the end of this article, you will have a thorough understanding of the available methods and be able to choose the most appropriate one for your programming needs.
Use the for
Loop to Implement Manual Deep Copying in C++
Two types of copying exist in programming, with one being shallow copying and the other being deep copying.
Shallow copying is in the cases where two different arrays (the original and the new) refer to the same content. Deep copying is when two different arrays refer to two independent addresses in memory while carrying the same content.
A for
loop is an entry-controlled loop that is able to, in one line of code, perform an n
number of steps.
Below is the syntax of the for
loop,
for (initialization; condition; update) {
// body of-loop
}
Here, initialization
is a statement that initializes the given variable and is executed just once.
The condition
is used to determine if the for
loop will get terminated or not in each iteration. If the condition
comes on to be true
, then the body-of-loop
gets automatically executed, and if not true
, then the loop automatically gets terminated.
The update
changes the value of the initialized variable in the next run of the loop.
We can make use of a simple for
loop to conduct this task given to us. This is further explained by taking an example code given below.
#include <iostream>
int main()
{
const int x = 5;
int orig[] = {10,20,30,40,50};
int new1[x];
for (int i = 0; i < x; i++) {
new1[i] = orig[i];
}
for (int &i: new1) {
std::cout << i << ' ';
}
return 0;
}
The above code specifies an original array and makes use of the for
loop to copy it into another brand-new array.
Output:
As seen in the output, the new array is displayed that has its contents copied from the original array.
Use the Copy Constructor to Copy a C++ Array
A copy constructor
can be defined as a function that is able to initialize an object with the help of another object that exists in the same class as this function. The members of an already existing object can be copied to initialize a new object.
The syntax of the copy constructor is,
Class_name(const class_name &old_object);
This method becomes a viable option when working post any versions after C++ 11. The copy constructor is capable of directly copying a std::array
.
However, we should note that this method is not recommended and might not work with C-style arrays.
Consider the following code.
#include <iostream>
#include <array>
int main()
{
const int x = 5;
std::array<int, x> orig = {10, 20, 30, 40, 50};
std::array<int, x> new1 = orig; // or, std::array<int, x> new1(orig);
for (int &i: new1) {
std::cout << i << ' ';
}
return 0;
}
The above code makes use of the copy constructor
to initialize a new array by copying all the details of an already existing object.
Output:
Similar to the ways above, we can see that the newly created array is able to successfully copy the elements from the original array with the help of the copy constructor
.
Use the copy()
Function to Copy an Array in C++
The copy()
method is the recommended way of copying range-based structures with a single function call. copy()
takes the first and the last elements of the range and the beginning of the destination array.
The syntax for the copy()
function is as follows,
copy(first, last, result)
The copy()
function accepts the following parameters,
first
: A simple iterator that specifies the first index of the array from which we need to copy the elements.last
: A simple iterator that specifies the last index of the array to which we need to copy the elements.result
: An iterator that points to the newly created array to which we need to copy the elements. There is a condition involved here that the size of thisresult
should be appropriate enough to efficiently accommodate all the elements in between the specified range[first, last)
.
Notice that you might get undefined behavior if the third parameter is within the source range.
Consider the following code.
#include <iostream>
#include <vector>
#include <iterator>
#include <string>
using std::cout; using std::endl;
using std::vector; using std::copy;
using std::string;
int main() {
vector<string> str_vec = { "Drake", "Tory",
"Breezy", "Russ",
"Abel", "Future" };
vector<string> new_vec(str_vec.size());
copy(str_vec.begin(), str_vec.end(), new_vec.begin());
cout << "new_vec - | ";
copy(new_vec.begin(), new_vec.end(),
std::ostream_iterator<string>(cout," | "));
cout << endl;
return EXIT_SUCCESS;
}
The above code implements the copy()
function to copy the elements of the str_vec
vector and puts it into the new_vec
vector.
Output:
As seen in the output, the contents of the str_vec
vector are copied into the new_vec
vector.
Use the copy_backward()
Function to Copy an Array
The copy_backward()
method can copy an array in reverse order with the original element, but the order is preserved. Its working is almost entirely similar to the copy()
function, except for the fact that the process of copying starts backward and continues till all the elements are copied.
It is important to note, however, that the order of elements will not be a reversed one; only the process remains to be backward.
When copying the overlapping ranges, there is a rule of thumb one should mind when using the methods std::copy
and std::copy_backward
: copy
is appropriate when copying to the left (the beginning of the destination range is outside the source range). In contrast, copy_backward
is appropriate when copying to the right (the end of the destination range is outside the source range).
Let us consider an example code.
#include <iostream>
#include <vector>
#include <iterator>
#include <string>
using std::cout;
using std::endl;
using std::vector;
using std::copy_backward;
using std::string;
int main() {
vector<string> str_vec = { "Drake", "Tory",
"Breezy", "Russ",
"Abel", "Future" };
vector<string> new_vec(str_vec.size());
copy_backward(str_vec.begin(), str_vec.end(), new_vec.end());
cout << "new_vec - | ";
copy(new_vec.begin(), new_vec.end(),
std::ostream_iterator<string>(cout," | "));
cout << endl;
return EXIT_SUCCESS;
}
The above method makes use of the copy_backward()
function to copy the contents of one array to another. The process is carried out in almost a similar way to the above-mentioned copy()
function and provides the same output.
Output:
As we can see, the output is the same as the function above, and it is only the unseen process that has a little bit of a difference.
Use the assign()
Method to Copy an Array
A method that mainly deals with vectors, it can be improvised in cases of normal arrays as well. The assign()
function is originally utilized to assign fresh values to a vector.
Let us consider a vector x
that needs to be assigned a value. The syntax for that is shown below.
x.assign(first,last);
x.assign(n,val);
The parameters of the assign()
function are:
(first,last)
: This defines a range.first
points to the first element, andlast
refers to the last element.n
: This defines the number of times the value has to occur.val
: This specifies the value that needs to be assigned to the vector.
assign()
is a built-in method of a vector
container, which replaces the contents of the calling vector
object with passed elements of the range. The assign()
method could be handy when copying the vectors of the types, which can be converted to each other easily.
In the following code example, we demonstrate a way to copy a char
vector into the int
vector with a single assign
call. Let us consider the following example code:
#include <iostream>
#include <vector>
#include <iterator>
#include <string>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> char_vec { 'd', 'e', 'l', 'f', 't'};
vector<int> new_vec(char_vec.size());
new_vec.assign(char_vec.begin(), char_vec.end());
cout << "new_vec - | ";
copy(new_vec.begin(), new_vec.end(),
std::ostream_iterator<int>(cout," | "));
cout << endl;
return EXIT_SUCCESS;
}
The above code makes use of the assign()
function and copies the contents of one array to another while simultaneously converting the char
values in the original array to int
values in the newly created array.
Output:
Here, the output of the code is in an int
format as the function simultaneously converts char
to int
while copying from the old array to the new array.
Use the std::memcpy
Function to Copy a C++ Array
The memcpy()
function is utilized in cases where a block of memory needs to be copied from one given location to another. This function is contained within the <string.h>
header file.
Syntax:
void *memcpy(void *to, const void *from, size_t numBytes);
The parameters that this function has are,
to
: A pointer that points to the memory location where the data needs to be copied.from
: A pointer that points to the memory location from where the data needs to be copied.num
: Specifies the total number of bytes that need to be copied.
When dealing with POD (Plain Old Data) types like int
, char
, etc., implementing the std::memcpy
function becomes available to use. What this function does is create a binary copy of the given array.
Here’s an example code that implements the task using the std::memcpy
function.
#include <iostream>
#include <cstring>
int main()
{
const int x = 5;
int orig[] = {10, 20, 30, 40, 50};
int new1[x];
std::memcpy(&new1, &orig, sizeof(orig));
for (int &i: new1) {
std::cout << i << ' ';
}
return 0;
}
The above code makes use of the std::memcpy()
function to copy the block of memory held in the original array to another newly created array.
Output:
As we see, a basic copy operation is carried out, and the output above displays the newly created array with the copied values.
Conclusion
Copying arrays in C++ can be achieved through several methods, each with its own advantages and use cases. The best method for you depends on your specific requirements:
- Using either a
for
Loop or a copy constructor: This method is simple and suitable for small arrays with a known size. However, it may be less efficient for large arrays. - Using
std::copy
(C++ Standard Library):std::copy
is a versatile and efficient choice that works well for both small and large arrays. It is recommended for most scenarios, especially when working with C++ Standard Library containers. - Using
std::vector
(Dynamic Arrays): As showcased in thecopy()
,copy_backward()
, and theassign()
function, if you require dynamic sizing and flexibility, thestd::vector
is the preferred option. It’s an excellent choice for cases where you don’t know the array size at compile time.
Ultimately, the best method depends on the specific needs of your project.
For most cases, using std::copy
or the generic copy()
function with std::vector
is recommended due to their efficiency, safety, and compatibility with modern C++. However, consider the context and requirements of your program when choosing the appropriate method for copying arrays in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook