How to Convert Vector to Array in C++
-
Use the
data()
Method to Convert a Vector to an Array -
Use the
&
Address-Of Operator to Convert a Vector to an Array -
Use the
copy()
Function to Convert a Vector to an Array -
Use the
transform()
Function to Convert a Vector to an Array -
Use the
for
Loop to Convert a Vector to an Array - Conclusion
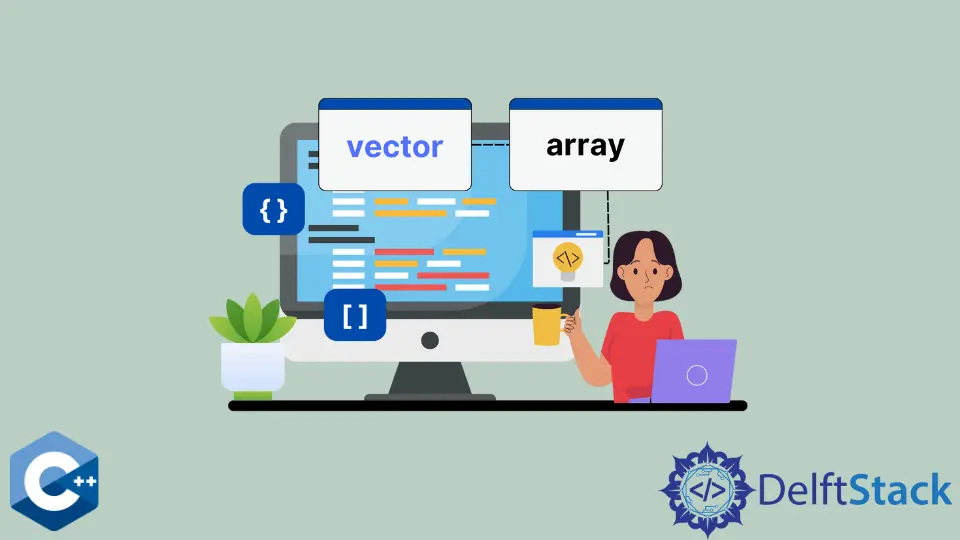
C++ programmers often encounter scenarios where the transition from a std::vector
to a traditional array is necessary. This can be prompted by the need to interface with functions or APIs that expect raw arrays or to leverage specific array-related functionalities.
In this article, we’ll explore various methods to convert a std::vector
to an array in C++, providing insights into their usage, benefits, and considerations.
Use the data()
Method to Convert a Vector to an Array
One of the convenient ways to convert a std::vector
to a conventional array is by utilizing the data()
method. This method is a member function of the std::vector
class, and it returns a pointer to the underlying array used by the std::vector
.
Essentially, it provides direct access to the memory location where the vector’s data is stored. This is beneficial when you need to interact with functions or APIs that expect a raw array.
Let’s explore how to convert a std::vector
to an array using the data()
method with a complete working example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
int* myArray = myVector.data();
std::cout << "Converted Array: ";
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myArray[i] << " ";
}
return 0;
}
Output:
Converted Array: 1 2 3 4 5
In the provided example, we start by creating a std::vector<int>
named myVector
and initialize it with some values.
The key step is the use of the data()
method on the vector, assigning its result to a pointer named myArray
. This pointer now holds the memory address of the first element in the underlying array of the vector.
After obtaining the array pointer, we can access the vector’s elements just like any other array. In the code, a simple loop is used to print the converted array’s elements to the console.
It’s important to note that when using the data()
method, you do not need to worry about memory management or deallocation. The std::vector
class takes care of this internally.
The array pointer obtained through data()
is only valid as long as the vector is not modified or reallocated. If the vector is modified, the pointer may become dangling, pointing to invalid memory.
Use the &
Address-Of Operator to Convert a Vector to an Array
While the data()
method is a convenient way to access the underlying array of a std::vector
, an alternative approach involves using the address-of operator (&
).
When applied to a variable, the &
operator returns the memory address where that variable is stored. By using this operator with the first element of a vector, we can obtain a pointer to the underlying array, similar to the data()
method.
Let’s delve into how to convert a std::vector
to an array using the &
address-of operator with a distinct example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {6, 7, 8, 9, 10};
int* myArray = &myVector[0];
std::cout << "Converted Array: ";
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myArray[i] << " ";
}
return 0;
}
Output:
Converted Array: 6 7 8 9 10
In this example, we begin by creating a std::vector<int>
called myVector
and initialize it with some values.
The crucial step here is obtaining the array pointer using the &
operator applied to the first element of the vector, assigned to the pointer myArray
. This pointer now holds the memory address of the vector’s underlying array.
Similar to the previous example, once we have the array pointer, we can access the vector’s elements as if they were in a traditional array. The provided loop iterates through the elements, printing them to the console.
It’s important to note that while using the &
operator to obtain the array pointer, you need to ensure that the vector is not empty. Attempting to get the address of the first element of an empty vector could result in undefined behavior.
Use the copy()
Function to Convert a Vector to an Array
In C++, the Standard Template Library (STL) provides the copy()
function, a powerful algorithm for copying elements from one range to another. Leveraging this function allows for an efficient and concise method to convert a std::vector
to a traditional array.
The copy()
function is part of the <algorithm>
header in C++. Its syntax is as follows:
template <class InputIt, class OutputIt>
OutputIt copy(InputIt first, InputIt last, OutputIt d_first);
Where:
first
,last
: Input iterators defining the range of elements to copy.d_first
: Output iterator pointing to the beginning of the destination range.
Let’s explore how to use the copy()
function to convert a std::vector
to an array with a distinct example:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {11, 12, 13, 14, 15};
int size = myVector.size();
int myArray[size];
std::copy(myVector.begin(), myVector.end(), myArray);
std::cout << "Converted Array: ";
for (int i = 0; i < size; ++i) {
std::cout << myArray[i] << " ";
}
return 0;
}
Output:
Converted Array: 11 12 13 14 15
In this example, we begin by creating a std::vector<int>
called myVector
and initialize it with some values. To convert this vector to an array, we first determine the size of the vector and create an array of the same size named myArray
.
The key step here is using the copy()
function, where we specify the range of elements in the vector (myVector.begin()
to myVector.end()
) and the starting point of the destination array (myArray
).
The copy()
function efficiently transfers the elements from the vector to the array, eliminating the need for explicit loops. After the copy operation, we can access the elements of the array just like a regular array.
The provided loop prints the elements of the converted array to the console.
Use the transform()
Function to Convert a Vector to an Array
In C++, the <algorithm>
header provides the std::transform()
function, a powerful tool for transforming elements in a range.
When it comes to converting a std::vector
to an array, std::transform()
proves to be a flexible option. It allows us to apply a transformation and copy elements from one container to another.
The transform()
function takes two input ranges and an output iterator. It applies a specified operation to each element in the input ranges and stores the result in the corresponding position of the output range.
This operation is provided as a unary or binary function, offering flexibility in how the elements are transformed.
template <class InputIt, class OutputIt, class UnaryOperation>
OutputIt transform(InputIt first1, InputIt last1, OutputIt d_first,
UnaryOperation unary_op);
Where:
first1
,last1
: Input iterators defining the source range.d_first
: Output iterator pointing to the beginning of the destination range.unary_op
: Unary function that will be applied to each element in the source range.
Let’s explore how to use the std::transform()
function to convert a std::vector
to an array with a distinctive example:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {21, 22, 23, 24, 25};
int myArray[myVector.size()];
std::transform(myVector.begin(), myVector.end(), myArray,
[](int element) { return element; });
std::cout << "Converted Array: ";
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myArray[i] << " ";
}
return 0;
}
Output:
Converted Array: 21 22 23 24 25
Similar to the previous example, we first create a std::vector<int>
named myVector
and initialize it with some values. Then, we declare an array myArray
with the same size as the vector.
The std::transform()
function is then employed to copy elements from the vector to the array.
The arguments passed to std::transform()
include the beginning and end iterators of the source range (myVector.begin()
and myVector.end()
), the iterator pointing to the starting position in the target range (myArray
), and a lambda function that specifies the transformation.
In this case, the lambda simply returns the element itself.
The function applies this transformation to each element in the source range and stores the result in the destination range. Finally, a loop is used to display the elements of the converted array on the console.
Use the for
Loop to Convert a Vector to an Array
The for
loop provides a manual approach for converting a std::vector
to an array. While it is not as concise as some other methods, using a for
loop grants direct control over the conversion process.
The for
loop is a control flow statement in C++ that allows the repeated execution of a block of statements. In the context of converting a vector to an array, a for
loop can be utilized to iterate through the vector’s elements and copy them one by one into the array.
for (initialization; condition; update) {
// Code to be executed in each iteration
}
Where:
initialization
: Executed once at the beginning of the loop.condition
: Checked before each iteration. If false, the loop exits.update
: Executed at the end of each iteration.
Let’s explore how to use a for
loop to convert a std::vector
to an array with a unique example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {31, 32, 33, 34, 35};
int myArray[myVector.size()];
for (size_t i = 0; i < myVector.size(); ++i) {
myArray[i] = myVector[i];
}
std::cout << "Converted Array: ";
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myArray[i] << " ";
}
return 0;
}
Output:
Converted Array: 31 32 33 34 35
As you can see, the loop’s initialization sets the index i
to 0
, and the condition checks whether i
is less than the size of the vector. As long as this condition is true, the loop continues.
In each iteration, the myArray[i]
element is assigned the value of myVector[i]
.
Then, a second loop is used to display the elements of the converted array on the console. While this method involves more explicit steps, it offers direct control over the copying process and may be preferable in certain scenarios.
Conclusion
Choosing the appropriate method for converting a std::vector
to an array depends on factors such as efficiency, code readability, and the need for additional transformations during the process. Each method presented here offers a unique set of advantages, allowing C++ developers to select the approach that best suits their specific requirements.
Whether the simplicity of data()
or the flexibility of algorithms like transform()
, knowing these techniques enhances a programmer’s ability to manipulate data structures effectively in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook