C++에서 벡터를 배열로 변환하는 방법
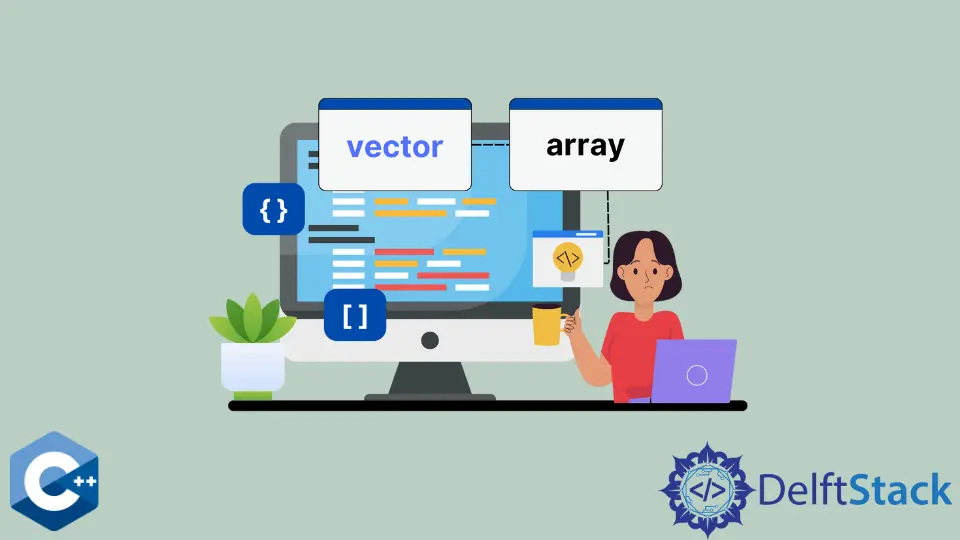
이 기사에서는 C++에서 벡터를 배열로 변환하는 방법을 소개합니다.
data()
메서드를 사용하여 벡터를 배열로 변환
C++ 표준은vector
컨테이너 요소가 메모리에 연속적으로 저장되도록 보장하므로 내장 벡터 메소드 인data
를 호출하고 다음과 같이 새로 선언 된double
포인터에 반환 된 주소를 할당 할 수 있습니다. 다음 코드 샘플.
d_arr
포인터를 사용하여 요소를 수정하면 원래 벡터의 데이터 요소가 변경됩니다. 왜냐하면 arr
요소 만 가리키고 새 위치에 복사하지 않기 때문입니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double *d_arr = arr.data();
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
-3.5; -21.1; -1.99; 0.129; 2.5; 3.111;
-3.5; -21.1; -1.99; 0.129; 2.5; 3.111;
연산자의&
주소를 사용하여 벡터를 배열로 변환
또는 앰퍼샌드 연산자를 사용할 수 있습니다. 앰퍼샌드 연산자는 메모리에있는 객체의 주소를 가져 와서 새로 선언 된 double
포인터에 할당합니다.
이 예에서는 vector
의 첫 번째 요소 주소를 가져 오지만 다른 요소에 대한 포인터를 추출하고 필요에 따라 작업을 수행 할 수 있습니다.
요소는d_arr[index]
배열 표기법을 사용하여 액세스 할 수 있으며 이전 방법과 유사하게d_arr
에 대한 모든 수정은 arr
데이터에 영향을줍니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double *d_arr = &arr[0];
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
copy()
함수를 사용하여 벡터를 배열로 변환
copy()
메소드는 벡터를double
배열로 변환하여 데이터 요소가 다른 메모리 위치로 복사되도록 할 수 있습니다. 나중에 원래 ‘벡터’데이터를 변경하는 것에 대해 걱정하지 않고 수정할 수 있습니다.
스택 메모리로 할당 된 6 개의 고정 요소가있는d_arr
를 선언하고 있습니다. 대부분의 시나리오에서 배열의 크기는 미리 알 수 없으므로new
또는malloc
과 같은 메소드와 함께 동적 메모리 할당을 사용해야합니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double d_arr[6];
copy(arr.begin(), arr.end(), d_arr);
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
new_vec - | 97 | 98 | 99 | 100 | 101 |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook