How to Get Current Directory in C++
-
Use the
getcwd
Function to Get Current Directory -
Use the
std::filesystem::current_path
Function to Get Current Directory -
Use the
get_current_dir_name
Function to Get Current Directory
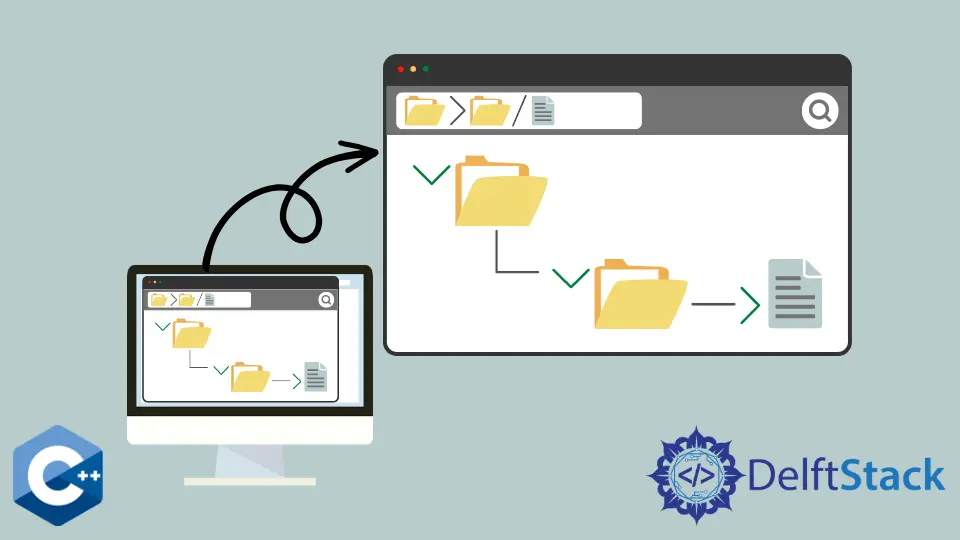
This article will explain several methods of how to get the current directory in C++.
Use the getcwd
Function to Get Current Directory
The getcwd
is a POSIX compliant function, available on many Unix based systems, and it can retrieve the current working directory. The function takes two parameters - the first char*
buffer where the directory pathname is stored and the buffer’s size. Note that we are declaring char
fixed-length array and allocate 256 element buffer, as an arbitrary example. If the call is successful, the stored name of the current directory can be printed by accessing the char
buffer.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char tmp[256];
getcwd(tmp, 256);
cout << "Current working directory: " << tmp << endl;
return EXIT_SUCCESS;
}
Output:
Current working directory: /tmp/projects
Use the std::filesystem::current_path
Function to Get Current Directory
The std::filesystem::current_path
method is part of the C++ filesystem library and is the recommended way of retrieving the current directory by the modern coding guidelines. Note that the filesystem
library was added after the C++17 version and the corresponding version of the compiler is required to work with the following code.
std::filesystem::current_path
without any arguments returns the current working directory and can be directly outputted to console. If the directory path is passed to the function, it changes the current directory to the given path.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
cout << "Current working directory: " << current_path() << endl;
return EXIT_SUCCESS;
}
Output:
Current working directory: /tmp/projects
Use the get_current_dir_name
Function to Get Current Directory
The get_current_dir_name
is the C library function that is similar to the previous method except that it returns char*
where the path of the current working directory is store after a successful call. get_current_dir_name
automatically allocates enough dynamic memory for the pathname to be stored, but the caller is responsible to free the memory before the program exit.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char *cwd = get_current_dir_name();
cout << "Current working directory: " << cwd << endl;
free(cwd);
return EXIT_SUCCESS;
}
Output:
Current working directory: /tmp/projects
Another useful feature from the C++ filesystem library is the std::filesystem::directory_iterator
object, which can be utilized to iterate through the elements in a given directory path. Note that elements can be files, symlinks, directories, or sockets, and their iteration order is unspecified. In the following example, we output the pathname of each element in the current working directory.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
using std::filesystem::directory_iterator;
int main() {
for (auto& p : directory_iterator("./")) cout << p.path() << '\n';
return EXIT_SUCCESS;
}
Output:
"./main.cpp"
"./a.out"
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook