在 C++ 中获取当前目录
Jinku Hu
2023年10月12日
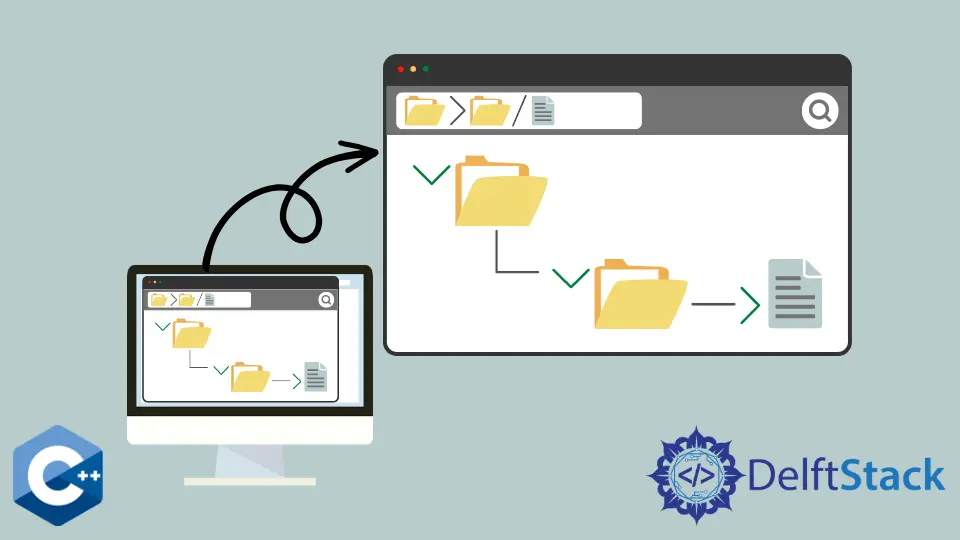
本文将介绍几种在 C++ 中获取当前目录的方法。
使用 getcwd
函数获取当前目录的方法
getcwd
是一个兼容 POSIX 的函数,在许多基于 Unix 的系统上都可以使用,它可以检索当前的工作目录。该函数需要两个参数-第一个 char*
缓冲区,其中存放目录路径名和缓冲区的大小。请注意,我们声明 char
为固定长度的数组,并分配 256 元素的缓冲区,作为一个随机的例子。如果调用成功,可以通过访问 char
缓冲区打印当前目录的存储名称。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char tmp[256];
getcwd(tmp, 256);
cout << "Current working directory: " << tmp << endl;
return EXIT_SUCCESS;
}
输出:
Current working directory: /tmp/projects
使用 std::filesystem::current_path
函数获取当前目录
std::filesystem::current_path
方法是 C++ 文件系统库的一部分,是现代编码准则推荐的检索当前目录的方式。需要注意的是,filesystem
库是在 C++17 版本之后加入的,要想使用下面的代码,需要相应版本的编译器。
std::filesystem::current_path
在没有任何参数的情况下,返回当前的工作目录,可以直接输出到控制台。如果将目录路径传递给函数,则将当前目录改为给定路径。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
cout << "Current working directory: " << current_path() << endl;
return EXIT_SUCCESS;
}
输出:
Current working directory: /tmp/projects
使用 get_current_dir_name
函数获取当前目录
get_current_dir_name
是 C 库函数,与前面的方法类似,只是在调用成功后返回 char*
,存储当前工作目录的路径。get_current_dir_name
会自动分配足够的动态内存来存储路径名,但调用者有责任在程序退出前释放内存。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char *cwd = get_current_dir_name();
cout << "Current working directory: " << cwd << endl;
free(cwd);
return EXIT_SUCCESS;
}
输出:
Current working directory: /tmp/projects
C++ 文件系统库的另一个有用的功能是 std::filesystem::directory_iterator
对象,它可以用来遍历给定目录路径中的元素。注意,元素可以是文件、符号链接、目录或套接字,它们的迭代顺序是不指定的。在下面的例子中,我们输出当前工作目录中每个元素的路径名。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
using std::filesystem::directory_iterator;
int main() {
for (auto& p : directory_iterator("./")) cout << p.path() << '\n';
return EXIT_SUCCESS;
}
输出:
"./main.cpp"
"./a.out"
作者: Jinku Hu