C++ で現在のディレクトリを取得
-
カレントディレクトリを取得するには
getcwd
関数を使用する -
関数
std::filesystem::current_path
を用いてカレントディレクトリを取得する -
関数
get_current_dir_name
を用いてカレントディレクトリを取得する
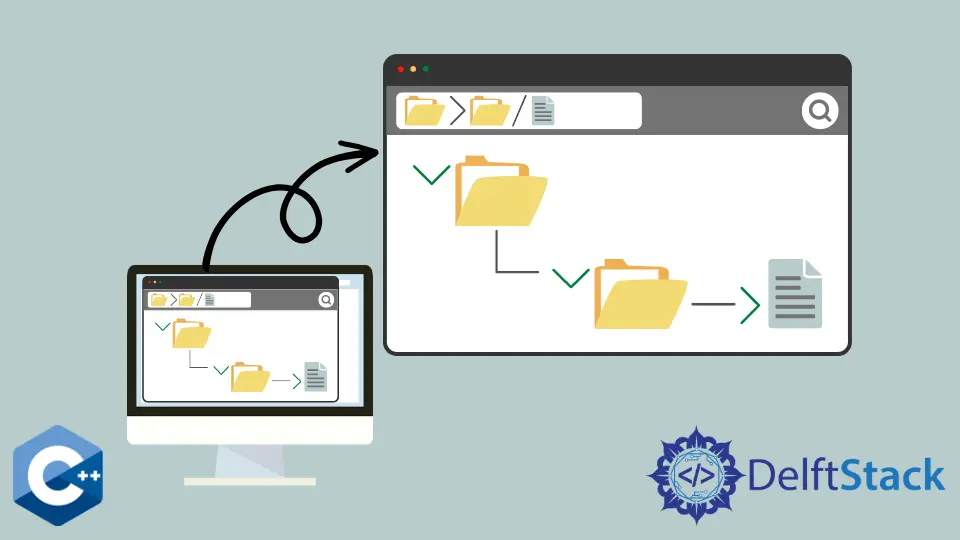
この記事では、C++ でカレントディレクトリを取得する方法をいくつか説明します。
カレントディレクトリを取得するには getcwd
関数を使用する
getcwd
は POSIX に準拠した関数であり、多くの Unix ベースのシステムで利用可能であり、現在の作業ディレクトリを取得することができます。この関数は 2つのパラメータを取ります。任意の例として、char
を固定長の配列として宣言し、256 要素のバッファを確保していることに注意してください。呼び出しが成功した場合、char
バッファにアクセスすることで、現在のディレクトリの保存されている名前を表示することができます。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char tmp[256];
getcwd(tmp, 256);
cout << "Current working directory: " << tmp << endl;
return EXIT_SUCCESS;
}
出力:
Current working directory: /tmp/projects
関数 std::filesystem::current_path
を用いてカレントディレクトリを取得する
std::filesystem::current_path
メソッドは C++ ファイルシステムライブラリの一部であり、最新のコーディングガイドラインで推奨されているカレントディレクトリの取得方法です。なお、filesystem
ライブラリは C++17 バージョン以降に追加されたものであり、以下のコードを扱うには対応するバージョンのコンパイラが必要です。
引数なしの std::filesystem::current_path
は現在の作業ディレクトリを返し、直接コンソールに出力することができます。ディレクトリパスが関数に渡された場合、カレントディレクトリを指定されたパスに変更します。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
cout << "Current working directory: " << current_path() << endl;
return EXIT_SUCCESS;
}
出力:
Current working directory: /tmp/projects
関数 get_current_dir_name
を用いてカレントディレクトリを取得する
get_current_dir_name
は C 言語のライブラリ関数であり、char*
を返す点を除いては前のメソッドと似ていますが、呼び出しに成功した後に現在の作業ディレクトリのパスが格納されます。get_current_dir_name
はパス名を格納するのに十分な動的メモリを自動的に確保しますが、プログラム終了前にメモリを解放するのは呼び出し元の責任です。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char *cwd = get_current_dir_name();
cout << "Current working directory: " << cwd << endl;
free(cwd);
return EXIT_SUCCESS;
}
出力:
Current working directory: /tmp/projects
C++ ファイルシステムライブラリのもう一つの便利な機能に std::filesystem::directory_iterator
オブジェクトがあります。要素にはファイル、シンボリックリンク、ディレクトリ、ソケットのいずれかを指定することができ、繰り返しの順序は不定であることに注意してください。次の例では、現在の作業ディレクトリ内の各要素のパス名を出力します。
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
using std::filesystem::directory_iterator;
int main() {
for (auto& p : directory_iterator("./")) cout << p.path() << '\n';
return EXIT_SUCCESS;
}
出力:
"./main.cpp"
"./a.out"