C++에서 현재 디렉토리 가져 오기
-
getcwd
함수를 사용하여 현재 디렉토리 가져 오기 -
std::filesystem::current_path
함수를 사용하여 현재 디렉토리 가져 오기 -
get_current_dir_name
함수를 사용하여 현재 디렉토리 가져 오기
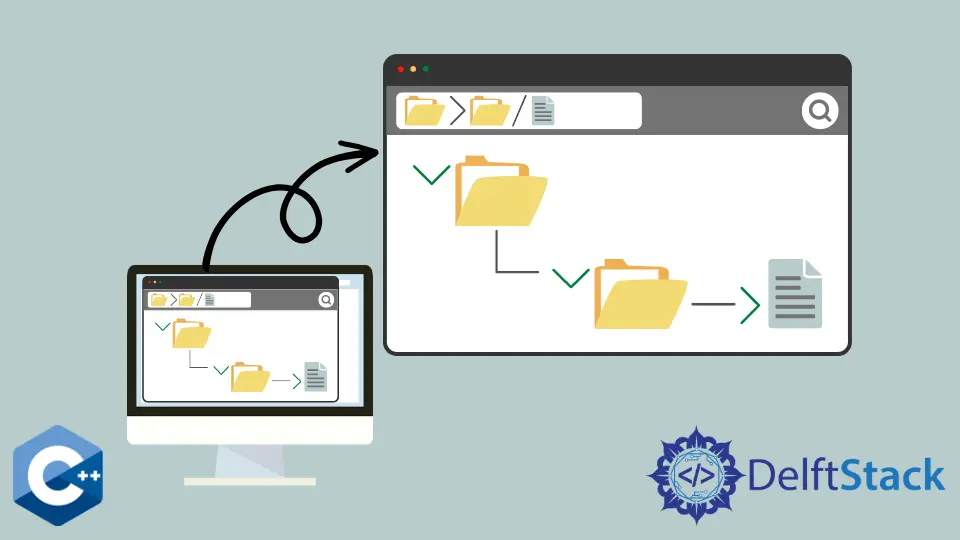
이 기사에서는 C++에서 현재 디렉토리를 얻는 방법에 대한 몇 가지 방법을 설명합니다.
getcwd
함수를 사용하여 현재 디렉토리 가져 오기
getcwd
는 많은 유닉스 기반 시스템에서 사용할 수있는 POSIX 호환 함수이며 현재 작업 디렉토리를 검색 할 수 있습니다. 이 함수는 두 개의 매개 변수, 즉 디렉토리 경로 이름이 저장되는 첫 번째 char*
버퍼와 버퍼의 크기를 사용합니다. 임의의 예로서char
고정 길이 배열을 선언하고 256 요소 버퍼를 할당합니다. 호출이 성공하면char
버퍼에 접근하여 현재 디렉토리의 저장된 이름을 인쇄 할 수 있습니다.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char tmp[256];
getcwd(tmp, 256);
cout << "Current working directory: " << tmp << endl;
return EXIT_SUCCESS;
}
출력:
Current working directory: /tmp/projects
std::filesystem::current_path
함수를 사용하여 현재 디렉토리 가져 오기
std::filesystem::current_path
메소드는 C++ 파일 시스템 라이브러리의 일부이며 최신 코딩 지침에 따라 현재 디렉토리를 검색하는 데 권장되는 방법입니다. C++ 17 버전 이후에filesystem
라이브러리가 추가되었으며 다음 코드를 사용하려면 해당 버전의 컴파일러가 필요합니다.
인수없이std::filesystem::current_path
는 현재 작업 디렉토리를 반환하며 콘솔에 직접 출력 할 수 있습니다. 디렉토리 경로가 함수에 전달되면 현재 디렉토리를 주어진 경로로 변경합니다.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
cout << "Current working directory: " << current_path() << endl;
return EXIT_SUCCESS;
}
출력:
Current working directory: /tmp/projects
get_current_dir_name
함수를 사용하여 현재 디렉토리 가져 오기
get_current_dir_name
은 호출 성공 후 현재 작업 디렉토리의 경로가 저장된char*
를 반환한다는 점을 제외하면 이전 메소드와 유사한 C 라이브러리 함수입니다. get_current_dir_name
은 경로 이름을 저장할 충분한 동적 메모리를 자동으로 할당하지만 호출자는 프로그램이 종료되기 전에 메모리를 해제해야합니다.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
int main() {
char *cwd = get_current_dir_name();
cout << "Current working directory: " << cwd << endl;
free(cwd);
return EXIT_SUCCESS;
}
출력:
Current working directory: /tmp/projects
C++ 파일 시스템 라이브러리의 또 다른 유용한 기능은std::filesystem::directory_iterator
객체로, 지정된 디렉토리 경로의 요소를 반복하는 데 사용할 수 있습니다. 요소는 파일, 심볼릭 링크, 디렉토리 또는 소켓 일 수 있으며 반복 순서는 지정되지 않습니다. 다음 예에서는 현재 작업 디렉토리에있는 각 요소의 경로 이름을 출력합니다.
#include <unistd.h>
#include <filesystem>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::current_path;
using std::filesystem::directory_iterator;
int main() {
for (auto& p : directory_iterator("./")) cout << p.path() << '\n';
return EXIT_SUCCESS;
}
출력:
"./main.cpp"
"./a.out"
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook