How to Create a File in C++
-
Use
std::fstream
,std::open
andstd::ios_base::out
to Create a File in C++ -
Use
std::fstream
,std::open
andstd::ios_base::app
to Create a File in C++ -
Use
std::fstream
andfopen
Function to Create a File in C++
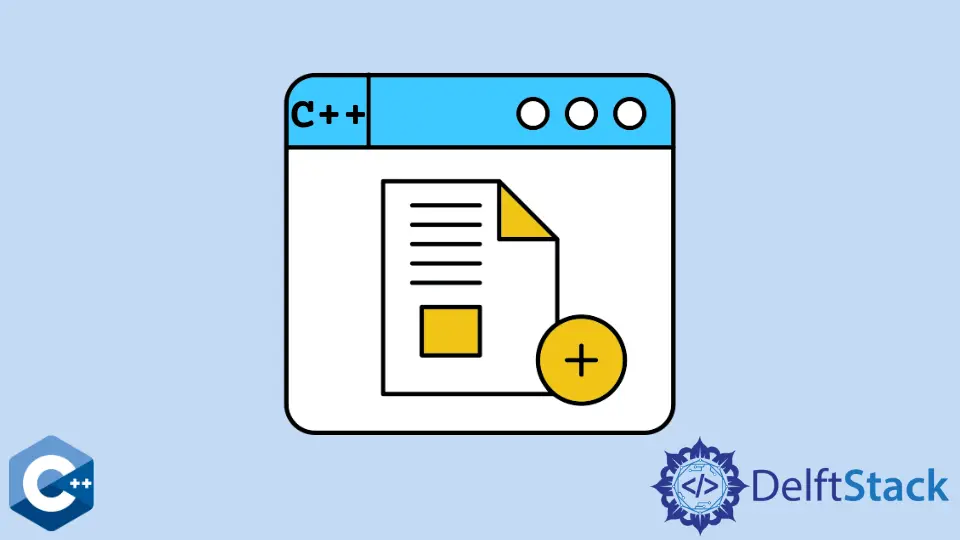
This article will explain several methods of how to create a file in C++.
Use std::fstream
, std::open
and std::ios_base::out
to Create a File in C++
Creating files is generally connected to the file opening operation, which in itself is the action of associating the file stream structure to the corresponding physical file on the disk. Typically, file creation operation depends on the mode in which the program opens the file. There are multiple modes to open a file, like write-only mode, read-only mode, append mode, etc. Usually, every mode that entails writing to the file is meant to create a new file if the given filename does not exist. Thus, we need to call the open
built-in function of std::fstream
to create a new file with the name provided as the first string
argument. The second argument to open
specifies the mode in which to open the file stream, and these modes are predefined by the language (see page).
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string filename("output.txt");
fstream output_fstream;
output_fstream.open(filename, std::ios_base::out);
if (!output_fstream.is_open()) {
cerr << "Failed to open " << filename << '\n';
} else {
output_fstream << "Maecenas accumsan purus id \norci gravida pellentesque."
<< endl;
cerr << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Use std::fstream
, std::open
and std::ios_base::app
to Create a File in C++
Alternatively, we can open the file in append mode denoted by std::ios_base::app
and force the stream to be positioned at the end of the file on each writing. This mode also assumes to create a new file if it does not exist in the given path. Note that, successfully opened file stream can be verified with the is_open
function that returns true
when the stream is associated.
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string text("Large text stored as a string\n");
string filename2("output2.txt");
fstream outfile;
outfile.open(filename2, std::ios_base::app);
if (!outfile.is_open()) {
cerr << "failed to open " << filename2 << '\n';
} else {
outfile.write(text.data(), text.size());
cerr << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Use std::fstream
and fopen
Function to Create a File in C++
Another option to create a file is to utilize the C standard library interface fopen
, which returns FILE*
structure. The function takes two arguments: a character string that specifies the file pathname to be opened and another string literal to indicate the mode in which to open. Six common modes are defined: r
- read-only when the stream is positioned at the beginning, w
- write only - at the beginning, a
- write-only (append) - positioned at the end of the file, and the plus versions of the three - r+
, w+
, a+
, which additionally include the opposite mode.
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string text("Large text stored as a string\n");
fstream outfile;
string filename3("output3.txt");
FILE *o_file = fopen(filename3.c_str(), "w+");
if (o_file) {
fwrite(text.c_str(), 1, text.size(), o_file);
cerr << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook