How to Dynamically Allocate an Array in C++
-
Use the
new()
Operator to Dynamically Allocate Array in C++ -
Use the
std::unique_ptr
Method to Dynamically Allocate Array in C++ -
Use
std::make_unique()
Method to Dynamically Allocate Array in C++ - Conclusion
- FAQ
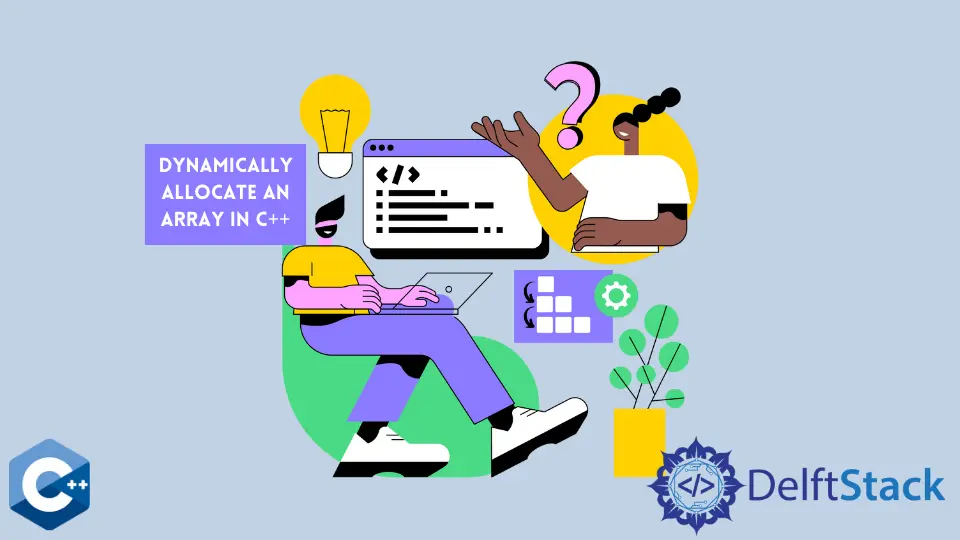
Dynamically allocating an array in C++ is an essential skill for any programmer looking to manage memory efficiently. Unlike static arrays, which have a fixed size determined at compile time, dynamic arrays offer flexibility, allowing you to allocate memory during runtime. This capability is particularly useful when the size of the array is not known beforehand or when working with large datasets.
In this article, we will explore the methods to dynamically allocate arrays in C++ and provide clear examples to illustrate each method. Whether you’re a beginner or an experienced developer, understanding dynamic memory allocation will enhance your programming toolkit and improve your software’s performance.
Use the new()
Operator to Dynamically Allocate Array in C++
The new
operator allocates the object on the heap
memory dynamically and returns a pointer to the location. In this example program, we declare the constant character array and size as an int
variable. Then, we dynamically allocate the char
array and assign the corresponding values to its elements in the for
loop body.
Note that the delete
operator must be explicitly called once the memory associated with the arr
pointer is no longer needed. The specified brackets after the delete
operator tell the compiler that the pointer refers to the first element of an array. If the brackets are omitted when deleting a pointer to an array, the behavior is undefined.
#include <iostream>
using std::cout;
using std::endl;
constexpr int SIZE = 10;
static const char chars[] = {'B', 'a', 'd', 'C', 'T', 'L', 'A', 'R', 'e', 'I'};
int main() {
char *arr = new char[SIZE];
for (int i = 0; i < SIZE; ++i) {
arr[i] = chars[i];
cout << arr[i] << "; ";
}
cout << endl;
delete[] arr;
return EXIT_SUCCESS;
}
Output:
B; a; d; C; T; L; A; R; e; I;
Use the std::unique_ptr
Method to Dynamically Allocate Array in C++
Another way to allocate a dynamic array is to use the std::unique_ptr
smart pointer, which provides a safer memory management interface. The unique_ptr
function is said to own the object it points; in return, the object gets destroyed once the pointer goes out of the scope. Contrary to the regular pointers, the smart pointer doesn’t need the delete
operator to be called by a programmer; instead, it’s called implicitly when the object is destroyed.
#include <iostream>
#include <memory>
using std::cout;
using std::endl;
constexpr int SIZE = 10;
static const char chars[] = {'B', 'a', 'd', 'C', 'T', 'L', 'A', 'R', 'e', 'I'};
int main() {
std::unique_ptr<char[]> arr(new char[SIZE]);
for (int i = 0; i < SIZE; ++i) {
arr[i] = chars[i];
cout << arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
B; a; d; C; T; L; A; R; e; I;
Use std::make_unique()
Method to Dynamically Allocate Array in C++
The make_unique
function is a more contemporary alternative to handle dynamic memory management. This method dynamically allocates an object of a given type and returns the std::unique_ptr
smart pointer. The type of the object is specified like the template parameter. On the other hand, the char
array of fixed size is allocated and assigned to the auto
type variable in the following example:
#include <iostream>
#include <memory>
using std::cout;
using std::endl;
constexpr int SIZE = 10;
static const char chars[] = {'B', 'a', 'd', 'C', 'T', 'L', 'A', 'R', 'e', 'I'};
int main() {
auto arr = std::make_unique<char[]>(SIZE);
for (int i = 0; i < SIZE; ++i) {
arr[i] = chars[i];
cout << arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
B; a; d; C; T; L; A; R; e; I;
Conclusion
Dynamically allocating arrays in C++ is a fundamental concept that allows for more flexible and efficient memory management. Whether you choose to use the new
operator for manual allocation or std::vector
for automatic handling, understanding these methods will empower you to write better and more robust C++ programs. As you continue to explore the language, remember that choosing the right method for dynamic memory allocation can significantly impact your program’s performance and reliability.
FAQ
-
What is dynamic memory allocation?
Dynamic memory allocation refers to the process of allocating memory at runtime, allowing programs to request and release memory as needed. -
Why should I use
std::vector
instead of raw arrays?
std::vector
automatically manages memory, reducing the risk of memory leaks and pointer errors, making it a safer and more efficient choice. -
How do I free memory allocated with
new
?
You can free memory allocated withnew
using thedelete
operator for single objects ordelete[]
for arrays. -
Can I resize a
std::vector
after it has been created?
Yes, you can resize astd::vector
using theresize()
method, which allows you to change its size dynamically. -
What happens if I forget to free dynamically allocated memory?
Failing to free dynamically allocated memory can lead to memory leaks, which can consume system resources and potentially crash your program.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook