How to Return 2D Array From Function in C++
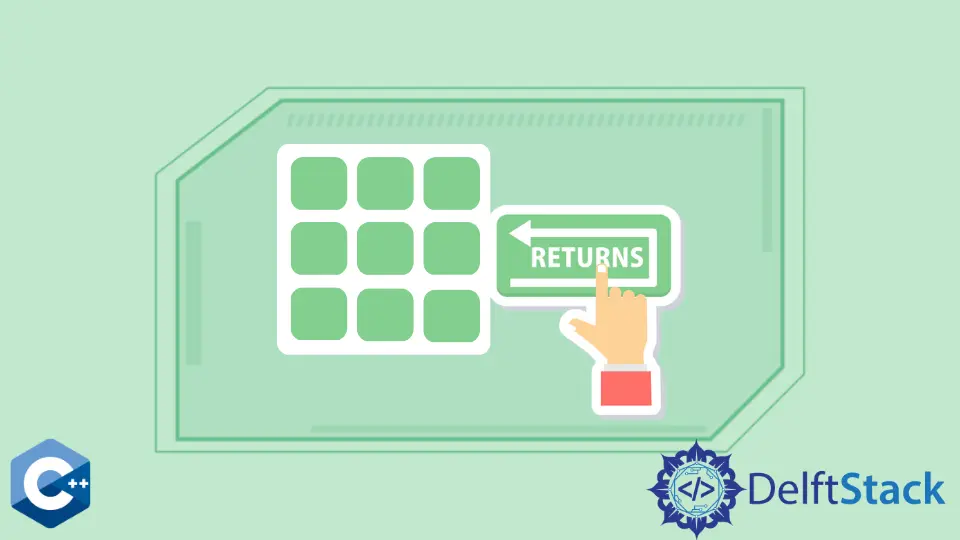
Returning a 2D array from a function in C++ can be a bit tricky, especially for beginners. Unlike languages like Python, C++ does not allow you to return arrays directly. However, understanding the various methods to achieve this will not only enhance your programming skills but also equip you with the knowledge to tackle more complex problems.
In this article, we will discuss several effective methods for returning a 2D array from a function in C++. By the end, you’ll be able to implement these techniques confidently in your projects. So, let’s dive into the world of C++ arrays!
Method 1: Using Pointers
One of the most common methods to return a 2D array from a function in C++ is by using pointers. This method involves dynamically allocating memory for your array and returning a pointer to that memory. Here’s how to do it:
#include <iostream>
int** create2DArray(int rows, int cols) {
int** array = new int*[rows];
for (int i = 0; i < rows; i++) {
array[i] = new int[cols];
}
return array;
}
void fillArray(int** array, int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
array[i][j] = i * j;
}
}
}
void deleteArray(int** array, int rows) {
for (int i = 0; i < rows; i++) {
delete[] array[i];
}
delete[] array;
}
int main() {
int rows = 3, cols = 4;
int** myArray = create2DArray(rows, cols);
fillArray(myArray, rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
std::cout << myArray[i][j] << " ";
}
std::cout << std::endl;
}
deleteArray(myArray, rows);
return 0;
}
Output:
0 0 0 0
0 1 2 3
0 2 4 6
In this code, we first create a 2D array using dynamic memory allocation with pointers. The create2DArray
function allocates memory for the array and returns a pointer to it. The fillArray
function populates the array with values, while the deleteArray
function is essential for deallocating the memory to prevent memory leaks. This method provides flexibility, especially when the size of the array is determined at runtime.
Method 2: Using std::vector
Another popular approach in modern C++ is to use the std::vector
class from the Standard Template Library (STL). Vectors are dynamic arrays that can resize themselves automatically. Here’s how to return a 2D vector from a function:
#include <iostream>
#include <vector>
std::vector<std::vector<int>> create2DVector(int rows, int cols) {
std::vector<std::vector<int>> vec(rows, std::vector<int>(cols));
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
vec[i][j] = i * j;
}
}
return vec;
}
int main() {
int rows = 3, cols = 4;
std::vector<std::vector<int>> myVector = create2DVector(rows, cols);
for (const auto& row : myVector) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
return 0;
}
Output:
0 0 0 0
0 1 2 3
0 2 4 6
In this example, we utilize std::vector
to create a 2D array. The create2DVector
function initializes a 2D vector with specified dimensions and fills it with values. The main advantage of using vectors is that they automatically manage memory, making your code cleaner and less prone to memory-related errors. Additionally, vectors offer various useful functions, enhancing their usability.
Method 3: Using a Struct
If you want to encapsulate a 2D array along with its dimensions, using a struct can be an effective solution. This method allows you to return both the array and its size from a function. Here’s how it works:
#include <iostream>
struct Array2D {
int** array;
int rows;
int cols;
};
Array2D createArray2D(int rows, int cols) {
Array2D arr;
arr.rows = rows;
arr.cols = cols;
arr.array = new int*[rows];
for (int i = 0; i < rows; i++) {
arr.array[i] = new int[cols];
}
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
arr.array[i][j] = i + j;
}
}
return arr;
}
void deleteArray2D(Array2D arr) {
for (int i = 0; i < arr.rows; i++) {
delete[] arr.array[i];
}
delete[] arr.array;
}
int main() {
Array2D myArray = createArray2D(3, 4);
for (int i = 0; i < myArray.rows; i++) {
for (int j = 0; j < myArray.cols; j++) {
std::cout << myArray.array[i][j] << " ";
}
std::cout << std::endl;
}
deleteArray2D(myArray);
return 0;
}
Output:
0 1 2 3
1 2 3 4
2 3 4 5
In this code, we define a struct called Array2D
that holds a pointer to a 2D array, along with its dimensions. The createArray2D
function initializes the struct, allocates memory for the array, and fills it with values. This method not only returns the array but also its dimensions, making it easier to handle in the main function. Remember to free the allocated memory afterward to avoid memory leaks.
Conclusion
Returning a 2D array from a function in C++ can be accomplished through various methods, each with its own advantages and use cases. Whether you choose to use pointers, std::vector
, or a struct, understanding these techniques will enhance your programming skills and allow you to write more efficient code. Always remember to manage memory properly to avoid leaks, especially when using dynamic arrays. With practice, you’ll master these methods and be able to tackle more complex C++ programming challenges.
FAQ
-
How can I return a 2D array without using dynamic memory?
You can usestd::vector
to create a 2D array that automatically manages memory for you, making it easier to return. -
What are the advantages of using
std::vector
over raw pointers?
std::vector
handles memory management automatically, prevents memory leaks, and offers various functionalities that simplify array manipulation. -
Can I return a 2D array of different sizes in C++?
Yes, you can usestd::vector
since it allows dynamic resizing, making it suitable for 2D arrays of varying sizes. -
Is it necessary to delete dynamically allocated memory?
Yes, if you allocate memory usingnew
, you must usedelete
to free it; otherwise, you will encounter memory leaks. -
What is the best method for beginners to return a 2D array?
Usingstd::vector
is often the best choice for beginners, as it simplifies memory management and reduces the risk of errors.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook