How to Remove Spaces From String in C++
- Method 1: Using the erase and remove Functions
- Method 2: Using a Loop to Construct a New String
- Method 3: Using String Streams
- Conclusion
- FAQ
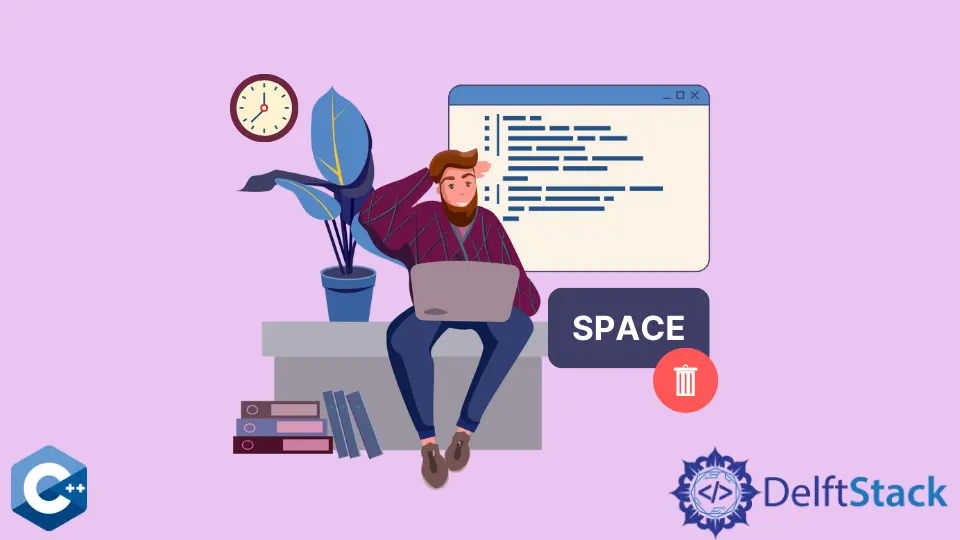
When working with strings in C++, you may often encounter the need to remove unnecessary spaces. Whether you’re cleaning up user input or processing data for display, eliminating spaces can enhance the readability and utility of your strings.
In this article, we will explore various methods to remove spaces from strings in C++. We will cover straightforward approaches, including using standard library functions and simple loops. By the end of this guide, you’ll be equipped with the knowledge to handle strings effectively, making your C++ programming experience smoother and more efficient.
Method 1: Using the erase and remove Functions
One of the most efficient ways to remove spaces from a string in C++ is by utilizing the erase
and remove
functions from the Standard Template Library (STL). This method involves removing all occurrences of a specific character—in this case, the space character—from the string.
Here’s a simple example:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello World! Welcome to C++.";
str.erase(remove(str.begin(), str.end(), ' '), str.end());
std::cout << str << std::endl;
}
Output:
HelloWorld!WelcometoC++.
In this code, we first include the necessary headers, iostream
, string
, and algorithm
. We define a string that contains spaces. The remove
function shifts all non-space characters to the front of the string and returns an iterator pointing to the new end of the string. The erase
function then removes the unwanted spaces from the string based on this iterator. The result is a string devoid of spaces, which we print to the console.
This method is concise and leverages the power of STL algorithms, making it a favorite among C++ developers.
Method 2: Using a Loop to Construct a New String
Another straightforward approach to removing spaces is to create a new string by iterating through the original string and appending only the non-space characters. This method is simple and intuitive.
Here’s how you can do it:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World! Welcome to C++.";
std::string result;
for (char c : str) {
if (c != ' ') {
result += c;
}
}
std::cout << result << std::endl;
}
Output:
HelloWorld!WelcometoC++.
In this example, we initialize an empty string called result
. We then use a range-based for loop to traverse each character in the original string. If the character is not a space, we append it to result
. Finally, we print the new string, which contains all the characters from the original string except for spaces.
This method is particularly useful for beginners as it provides a clear and straightforward way to manipulate strings in C++. It also allows for easy modifications if you want to remove other characters in the future.
Method 3: Using String Streams
For those who prefer a more sophisticated approach, using string streams can be an elegant solution. This method involves reading the string into a stream and then extracting words, which effectively removes spaces.
Here’s an example of how to implement this:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "Hello World! Welcome to C++.";
std::istringstream iss(str);
std::string word, result;
while (iss >> word) {
result += word;
}
std::cout << result << std::endl;
}
Output:
HelloWorld!WelcometoC++.
In this code, we utilize istringstream
from the <sstream>
library to create a stream from the original string. By using the extraction operator (>>
), we read each word from the stream, effectively skipping spaces. We then concatenate these words into the result
string. The final output is printed, showing the string without spaces.
This method is particularly powerful when you need to process strings with multiple spaces or other delimiters, as it can be easily adapted to handle various cases.
Conclusion
Removing spaces from strings in C++ can be accomplished in several ways, each with its own advantages. Whether you choose to use the erase
and remove
functions, construct a new string with a loop, or utilize string streams, the key is to select the method that best fits your specific needs. With the techniques outlined in this article, you’ll be well-equipped to handle strings effectively in your C++ projects, enhancing both performance and readability. Happy coding!
FAQ
-
What is the fastest way to remove spaces from a string in C++?
The fastest way is to use theerase
andremove
functions together, as it operates directly on the original string and avoids additional memory allocation. -
Can I remove other characters using the same methods?
Yes, you can modify the conditions in the loop or the character specified in theremove
function to eliminate any character, not just spaces. -
Are there any built-in C++ functions specifically for string manipulation?
C++ provides various functions in the standard library, such asfind
,substr
, andreplace
, which can be very useful for string manipulation tasks. -
Is it possible to remove spaces from a string in-place?
Yes, using theerase
andremove
approach modifies the original string directly, making it an in-place operation. -
How can I remove leading and trailing spaces from a string in C++?
You can use thefind_first_not_of
andfind_last_not_of
functions to locate the first and last non-space characters and then usesubstr
to create a new string without the leading and trailing spaces.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++