C++의 문자열에서 공백 제거
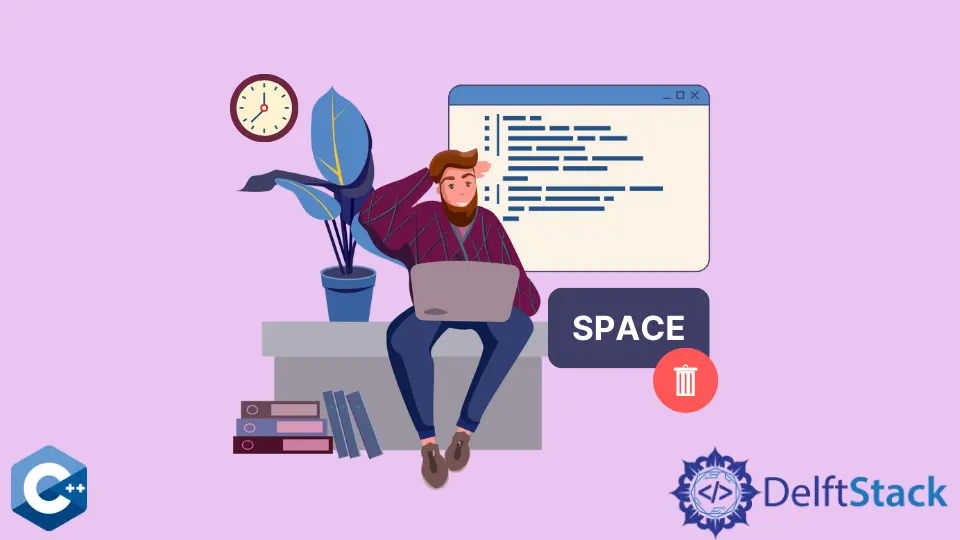
이 기사에서는 C++의 문자열에서 공백을 제거하는 방법에 대한 여러 방법을 보여줍니다.
erase-remove
Idiom을 사용하여 C++의 문자열에서 공백 제거
C++에서 범위 조작에 가장 유용한 방법 중 하나는std::erase
(대부분의 STL 컨테이너에 대한 내장 함수) 및std::remove
(부분 STL 알고리즘 라이브러리). 둘 다 주어진 개체에 대한 제거 작업을 수행하기 위해 연결되어 있습니다. std::remove
함수는 범위를 지정하기 위해 두 개의 반복자를 사용하고 제거 할 요소의 값을 표시하는 세 번째 인수를 사용합니다. 이 경우 공백 문자를 직접 지정하지만 문자열에서 모든 발생을 제거하기 위해 임의의 문자를 지정할 수 있습니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
str.erase(std::remove(str.begin(), str.end(), ' '), str.end());
cout << str << endl;
return EXIT_SUCCESS;
}
출력:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
반면에 사용자는std::remove
알고리즘의 세 번째 인수로 단항 술어를 전달할 수도 있습니다. 술어는 모든 요소에 대해bool
값으로 평가되어야하며 결과가true
이면 해당 값이 범위에서 제거됩니다. 따라서 공백-" "
, 줄 바꿈-\n
, 가로 탭-\t
및 기타 여러 공백 문자를 확인하는 사전 정의 된isspace
기능을 사용할 수 있습니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
str.erase(std::remove_if(str.begin(), str.end(), isspace), str.end());
cout << str << endl;
return EXIT_SUCCESS;
}
출력:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
사용자 지정 함수를 사용하여 C++의 문자열에서 공백 제거
이전의 모든 솔루션이 원래 문자열 개체를 수정했지만 때로는 모든 공백이 제거 된 새 문자열을 만들어야 할 수도 있습니다. 동일한erase-remove
관용구를 사용하여 사용자 정의 함수를 구현할 수 있습니다.이 관용구는 문자열 참조를 가져 와서 별도의 문자열 객체에 저장할 구문 분석 된 값을 반환합니다. 이 메서드는 제거해야하는 문자를 지정하는 다른 함수 매개 변수를 지원하도록 수정할 수도 있습니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string removeSpaces(const string& s) {
string tmp(s);
tmp.erase(std::remove(tmp.begin(), tmp.end(), ' '), tmp.end());
return tmp;
}
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
string newstr = removeSpaces(str);
cout << newstr << endl;
return EXIT_SUCCESS;
}
출력:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook