How to Declare Multiline String in C++
-
Use the
std::string
Class to Create a Multiline String in C++ -
Use the
std::string
Class With Escape Sequences to Create a Multiline String in C++ -
Use the
const char *
Notation to Declare Multiline String Literal in C++ -
Use the
const char *
Notation With Backlash Characters to Declare Multiline String Literal in C++ - Use Raw String Literals to Declare Multiline Strings in C++
- Use Macros to Create a Multiline String in C++
- Conclusion
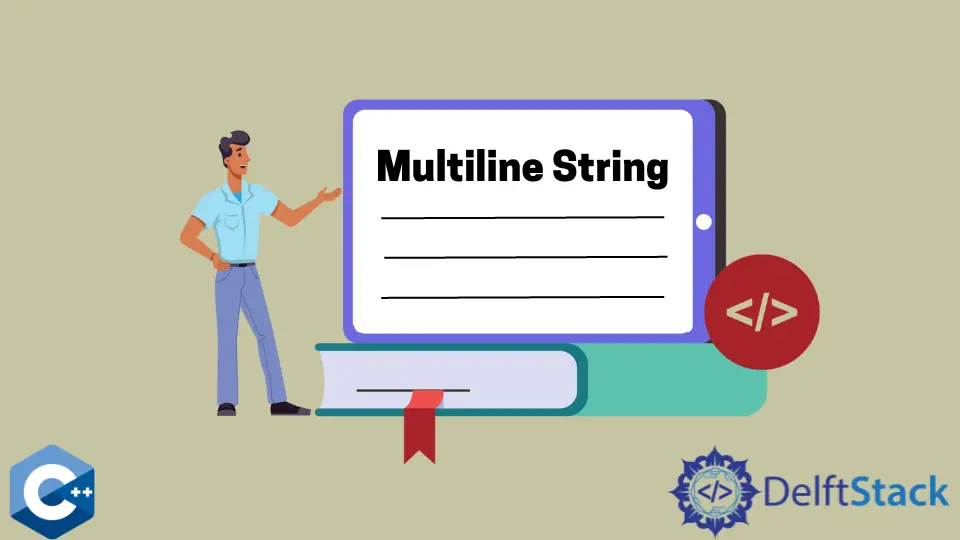
Multiline strings play a pivotal role in programming when dealing with lengthy text or preserving formatting. In C++, effectively handling multiline strings is essential for creating readable and maintainable code.
Fortunately, C++ provides several methods to work with multiline strings, each with advantages and use cases.
In this guide, we’ll explore all the available methods for working with multiline strings in C++, along with examples and comparisons, to help you choose the best approach for your programming needs.
Use the std::string
Class to Create a Multiline String in C++
The std::string
object can be initialized with a string value. In this case, we declare the s1
string variable to the main
function as a local variable.
C++ allows multiple double-quoted string literals to be concatenated automatically in a statement. As a result, one might include any number of lines while initializing the string
variable and keep the code more consistently readable.
Basic Syntax:
std::string multiline_str =
"Line 1 "
"Line 2 "
"Line 3";
Parameter:
multiline_str
: The parameter that assigns a value that includes the three lines of text.
Code Example:
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string s1 =
"I love"
" getting my doubts solved"
" by the help of Delft Stack Tutorials.";
copy(s1.begin(), s1.end(), std::ostream_iterator<char>(cout, ""));
cout << endl;
return EXIT_SUCCESS;
}
Output:
I love getting my doubts solved by the help of Delft Stack Tutorials.
In this code, we begin by including the necessary libraries. We then declare the usage of specific elements from the standard namespace, including copy
, cout
, endl
, string
, and vector
.
In the main
function, we initialize a string variable s1
using multiple string literals, creating a multiline string that concatenates the phrases "I love"
, "getting my doubts solved"
, and "by the help of Delft Stack Tutorials."
. Subsequently, we utilize the copy
function along with std::ostream_iterator<char>
to output each character of the string to the standard output (cout
) without any separation.
Finally, we append a newline character to enhance the readability of the output. Upon executing this code, we obtain a single-line output that combines the multiline string: "I love getting my doubts solved by the help of Delft Stack Tutorials."
.
Use the std::string
Class With Escape Sequences to Create a Multiline String in C++
If a backslash is placed at the end of each line, it gives the compiler the instructions to remove the new line and the preceding backslash character, which forms the multiline string. However, unlike the previous approach, the placement of indentation would matter here in this case.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string multiline_str =
"I love \
getting my doubts solved \
by the help of Delft Stack Tutorials.";
std::cout << multiline_str << std::endl;
return 0;
}
Output:
I love getting my doubts solved by the help of Delft Stack Tutorials.
In this code, we begin by including the necessary libraries. Inside the main
function, we initialize a std::string
variable named multiline_str
.
Using the backslash (\
) at the end of each line, we create a multiline string that concatenates the phrases "I love"
, "getting my doubts solved"
, and "by the help of Delft Stack Tutorials."
. Following this, we use std::cout
to print the contents of the multiline string to the console.
The std::endl
is then employed to add a newline character, ensuring proper formatting. Upon execution, the output will be a formatted multiline string that reads: "I love getting my doubts solved by the help of Delft Stack Tutorials."
.
Use the const char *
Notation to Declare Multiline String Literal in C++
In most situations, though, it may be more practical to declare a read-only string literal with a const
qualifier. This is most practical when the relatively long texts should be outputted to the console, and these texts are mostly static with little or no changes over time.
Note that the const
qualifier character string needs to be converted to the std::string
object before passing it as a copy
algorithm argument.
Basic Syntax:
const char *multiline_str =
"Line 1 "
"Line 2 "
"Line 3";
Parameter:
multiline_str
: This is the string literal parameter that is assigned to theconst char
.
Code Example:
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
const char *s2 =
"I love"
" getting my doubts solved"
" by the help of Delft Stack Tutorials";
string s1(s2);
copy(s1.begin(), s1.end(), std::ostream_iterator<char>(cout, ""));
cout << endl;
return EXIT_SUCCESS;
}
Output:
I love getting my doubts solved by the help of Delft Stack Tutorials
In this code, we include the necessary libraries. Inside the main
function, we declare the usage of specific elements from the standard namespace, including cin
, copy
, cout
, endl
, string
, and vector
.
We then initialize a const char *
variable named s2
with a multiline string created by concatenating the phrases "I love"
, "getting my doubts solved"
, and "by the help of Delft Stack Tutorials"
. Subsequently, we convert this const char *
to a std::string
variable named s1
.
Using the copy
function along with std::ostream_iterator<char>
, we output each character of the string to the standard output (cout
) without any separation. Finally, we append a newline character to enhance the readability of the output.
Upon executing this code, the output will be a single-line string: "I love getting my doubts solved by the help of Delft Stack Tutorials."
.
Use the const char *
Notation With Backlash Characters to Declare Multiline String Literal in C++
Alternatively, one can use the backslash character \
to construct a multiline string literal and assign it to the const
qualified char
pointer. Shortly, the backslash character needs to be included at the end of each line break, meaning that the string continues on the next line.
Be aware, though, that spacing becomes more error-prone to handle as any invisible characters like tabs or spaces will be included in the output. On the other hand, one might utilize this feature to display some patterns more easily on to console.
Code Example:
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
const char *s3 =
" This string will\n\
printed as the pyramid\n\
as one single string literal form\n";
printf("%s\n", s3);
return EXIT_SUCCESS;
}
Output:
This string will
printed as the pyramid
as one single string literal form
In this code, we include the necessary libraries. Inside the main
function, we declare the usage of specific elements from the standard namespace, including cin
, copy
, cout
, endl
, string
, and vector
.
We initialize a const char *
variable named s3
with a multiline string that includes line breaks and indentation, forming a pyramid-like structure. Using the printf
function, we print the contents of this multiline string to the console.
Upon executing this code, the output will be a formatted multiline string, displaying a pyramid shape like in the output above.
Use Raw String Literals to Declare Multiline Strings in C++
C++11 introduced raw string literals, which provide a concise and elegant way to define multiline strings without needing the help of escape sequences. Enclosing the text between R"()
lets you include newlines directly without hassle while improving code readability and maintainability.
Basic Syntax:
std::string multiline_str = R"(
Line 1
Line 2
Line 3
)";
There isn’t a traditional “parameter” being passed to a function or constructor. Instead, the right-hand side of the assignment (R"(...)"
) is a raw string literal, and it is directly used to initialize the std::string
variable multiline_str
.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string multiline_str = R"(I love
getting my doubts solved
by the help of Delft Stack Tutorials.)";
std::cout << multiline_str << std::endl;
return 0;
}
Output:
I love
getting my doubts solved
by the help of Delft Stack Tutorials.
In this code, we initialize a std::string
variable named multiline_str
using raw string literals. Within the raw string literal, we create a multiline string by directly including newlines without the need for escape sequences.
The content of the multiline string comprises the phrases "I love"
, "getting my doubts solved"
, and "by the help of Delft Stack Tutorials."
. We then use std::cout
to print the contents of this multiline string to the console, and std::endl
is added for proper formatting with a newline character.
Upon execution, the output will be a formatted multiline string like in the output above.
Use Macros to Create a Multiline String in C++
Finally, macros can be touched upon to create a multiline string literal in C++. The indentation would only matter a little here, as the solution would replace any consecutive whitespace characters with a single space.
Basix Syntax:
#define MULTILINE_STRING(...) #__VA_ARGS__
This macro, when used, will convert the provided multiline text into a string literal. The usage would look like this:
std::string multiline_str = MULTILINE_STRING(Line 1 Line 2 Line 3);
The parameter is represented by ...
within the macro definition. This is known as the “variadic macro” feature, allowing the macro to accept a variable number of arguments.
The multiline text within the parentheses is passed as arguments to the variadic macro. In this case, the content within the parentheses (multiline text) is the parameter.
Code Example:
#include <iostream>
#include <string>
#define MULTILINE_STRING(...) #__VA_ARGS__
int main() {
std::string multiline_str = MULTILINE_STRING(
I love getting my doubts solved by the help of Delft Stack Tutorials.);
std::cout << multiline_str << std::endl;
return 0;
}
Output:
I love getting my doubts solved by the help of Delft Stack Tutorials.
In this code, we utilize a macro defined with #define
to create a multiline string. The macro, named MULTILINE_STRING
, takes any content passed within the parentheses and converts it into a string literal.
Inside the main
function, we use this macro to create a std::string
variable named multiline_str
by passing the following phrase as an argument: "I love getting my doubts solved by the help of Delft Stack Tutorials."
. We then use std::cout
to print the contents of this multiline string to the console, and std::endl
is added for proper formatting with a newline character.
Upon execution, the output will be a single-line string.
Conclusion
Mastering multiline string handling in C++ is vital for code readability and maintainability, especially with extensive text. This guide comprehensively covers various methods, offering examples and comparisons to aid in selecting the best approach.
Whether utilizing std::string
, escape sequences, const char *
notations, raw string literals, or macros, each method has its advantages based on factors like readability and flexibility. Understanding and applying these techniques empower developers to efficiently handle multiline strings, fostering cleaner and more effective code in C++ projects.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++