C++ で複数行の文字列を宣言
胡金庫
2023年10月12日
C++
C++ String
-
C++ で複数行の文字列を宣言するには
std::string
クラスを使用する -
複数行の文字列リテラルを宣言するために
const char *
記法を使用する -
バックラッシュ文字を用いた
const char *
記法を用いる複数行の文字列リテラルを宣言する
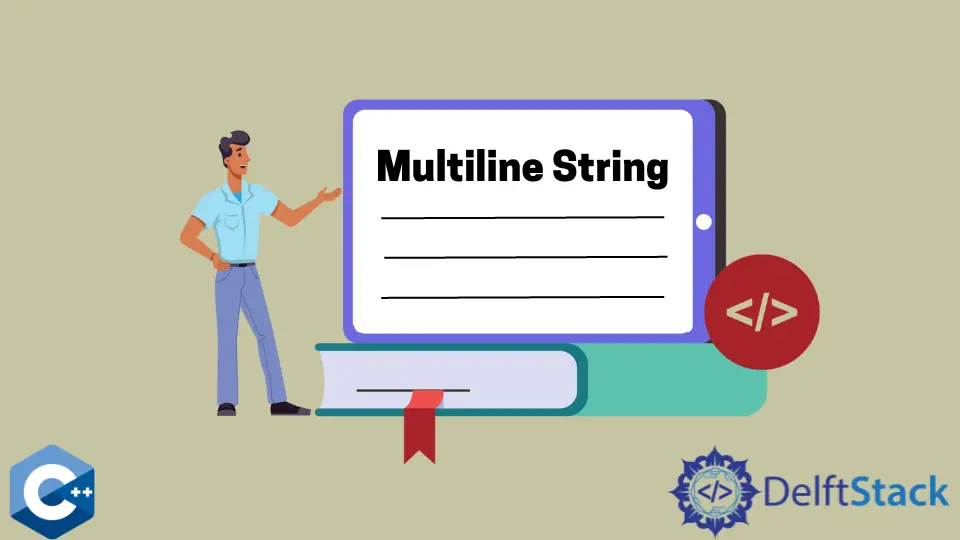
この記事では、C++ で複数行の文字列を宣言する方法をいくつか説明します。
C++ で複数行の文字列を宣言するには std::string
クラスを使用する
オブジェクト std::string
は文字列の値で初期化することができます。この場合、文字列変数 s1
を main
関数のローカル変数として宣言します。C++ では、複数の二重引用符で囲まれた文字列リテラルを文の中で自動的に連結することができます。その結果、string
変数を初期化する間に任意の行数を含めることができ、コードをより一貫して読みやすくすることができます。
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string s1 =
"This string will be printed as the"
" one. You can include as many lines"
"as you wish. They will be concatenated";
copy(s1.begin(), s1.end(), std::ostream_iterator<char>(cout, ""));
cout << endl;
return EXIT_SUCCESS;
}
出力:
This string will be printed as the one. You can include as many linesas you wish. They will be concatenated
複数行の文字列リテラルを宣言するために const char *
記法を使用する
しかし、ほとんどの状況では、const
修飾子で読み取り専用の文字列リテラルを宣言する方がより実用的かもしれません。これは比較的長いテキストがコンソールに出力され、これらのテキストはほとんどが静的で時間の経過とともにほとんど変化がない場合に最も実用的です。なお、const
修飾子の文字列は copy
アルゴリズムの引数として渡す前に std::string
オブジェクトに変換する必要があります。
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
const char *s2 =
"This string will be printed as the"
" one. You can include as many lines"
"as you wish. They will be concatenated";
string s1(s2);
copy(s1.begin(), s1.end(), std::ostream_iterator<char>(cout, ""));
cout << endl;
return EXIT_SUCCESS;
}
出力:
This string will be printed as the one. You can include as many linesas you wish. They will be concatenated
バックラッシュ文字を用いた const char *
記法を用いる複数行の文字列リテラルを宣言する
あるいは、複数行の文字列リテラルを構築し、const
修飾された char
ポインタにそれを代入するために、バックスラッシュ文字\
を利用することもできます。手短に言えば、バックスラッシュ文字は各改行の最後に含める必要があります。つまり、文字列は次の行に続きます。
しかし、タブやスペースのような見えない文字が出力に含まれてしまうので、スペーシングの処理はよりエラーになりやすいことに注意してください。一方で、いくつかのパターンをより簡単にコンソールに表示するためにこの機能を利用することもできます。
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
const char *s3 =
" This string will\n\
printed as the pyramid\n\
as one single string literal form\n";
cout << s1 << endl;
printf("%s\n", s3);
return EXIT_SUCCESS;
}
出力:
This string will
printed as the pyramid
as one single string literal form
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫