C++ Error ID Returned 1 Exit Status
-
Common Causes of
[Error]: Id returned 1 exit status
in C++ -
How to Fix the Error
[Error]: Id returned 1 exit status
in C++ -
FAQ: Troubleshooting
Id Returned 1 Exit Status
in C++ Projects - Conclusion
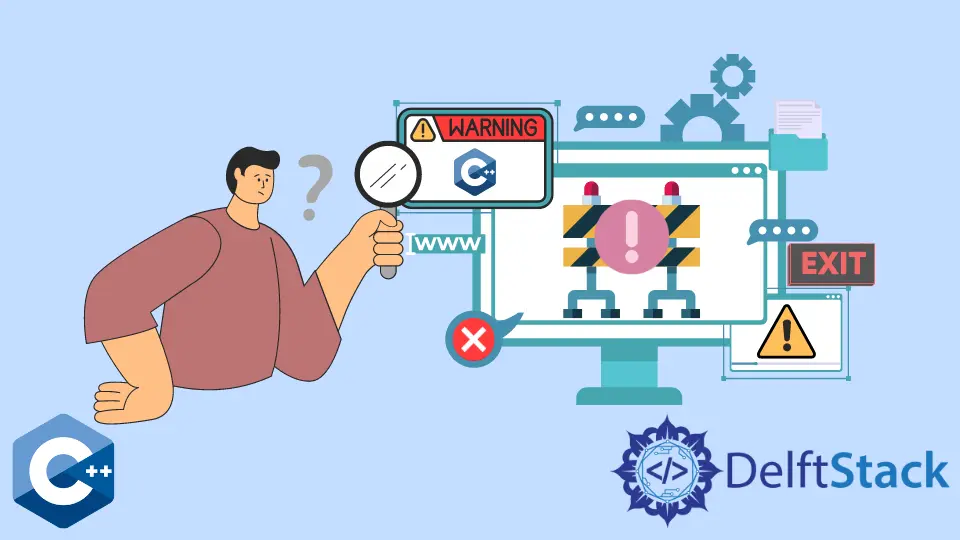
The error [Error]: Id returned 1 exit status
typically occurs during the compilation and linking process of a C++ program. This error is a linker error, and it indicates that there is an issue during the final step of creating the executable from the compiled source code.
A linker error typically arises when invalid object files are linked with the main object file. In such instances, the compiler faces challenges in loading the executable file due to incorrect prototyping and inappropriate header files.
Encountering the Error Id returned 1
in C++ signals a compiler alert about a code issue, often stemming from syntax errors or other coding problems hindering successful compilation and linking. The compiler not only identifies the error but also specifies its location, enabling a targeted debugging approach.
This allows developers to efficiently locate and rectify the problem at its source, facilitating a more effective resolution of the error message.
Common Causes of [Error]: Id returned 1 exit status
in C++
Below are some common causes of Error Id returned 1
in C++:
-
A syntax error in the C++ program.
-
A mismatch between the compiler and the library.
-
An incorrect link to a library.
-
An incorrect or missing header file.
-
Defining the same function or variable in multiple source files.
-
A missing main function.
How to Fix the Error [Error]: Id returned 1 exit status
in C++
Let’s discuss the solutions of these two errors:
-
A mismatch between the compiler version.
-
An incorrect or missing header file.
Example 1: Mismatch Between the Compiler Version
There are two major compiler versions: GCC and Microsoft Visual Studio. Below are four ways to fix this problem.
-
Download the latest version of the compiler from its website and install it on our computer.
-
Update our system with the latest updates from Windows Update, including installing any recommended updates for Visual Studio or GCC.
-
Use a different compiler, Clangor MinGW, to compile our code instead of GCC or Microsoft Visual Studio.
-
Examine how the
main()
function is written. The functionmain()
must be written in lowercase and with proper spelling.
Let’s discuss an example of this error.
Error - Example Code:
#include <stdio.h>
int Main() {
printf("My name is Muhammad Adil");
return 0;
}
We observe that the code contains a common mistake, as the main function is incorrectly declared with a capital M
. In C++, the main function serves as the entry point for the program and must be written in lowercase.
Output:
To address this issue, we will correct the function declaration by changing Main
to main
, ensuring proper adherence to C++ syntax standards. Let’s look at the following snippet.
Solution - Example Code:
#include <stdio.h>
int main() {
printf("My name is Muhammad Adil");
return 0;
}
Output:
In the above code, we have appropriately modified the declaration of the main
function, ensuring that it is written in lowercase. This adjustment resolves the error, allowing the program to execute successfully.
Example 2: Incorrect or Missing Header Files
Error - Example Code (main.cpp
):
#include "functions.h"
int main() {
definedFunction();
return 0;
}
This is the main file of the program, and it includes the functions.h
header file, where the declaration of definedFunction()
is present. The main
function calls definedFunction()
, which is declared but not defined in this file.
Error - Example Code (function.cpp
):
#include <iostream>
void undefinedFunction() {
std::cout << "This function is now defined." << std::endl;
}
In the code above, we create a function called undefinedFunction()
. However, notice that it doesn’t define the definedFunction()
, which is declared in the header file (functions.h
).
Error - Example Code (functions.h
):
#ifndef FUNCTIONS_H
#define FUNCTIONS_H
void definedFunction();
#endif
In the above header file, we create the definedFunction()
without providing its implementation. The purpose of this header file is to make the declaration visible to other files (like main.cpp
).
Output:
The error we encountered indicates that the compiler does not recognize the definedFunction()
because it hasn’t seen a declaration for that function before it’s used in main cpp file (main.cpp
).
Solution - Example Code:
function.cpp
:
#include <iostream>
#include "functions.h"
void definedFunction() {
std::cout << "This function is now defined." << std::endl;
}
functions.h
:
#ifndef FUNCTIONS_H
#define FUNCTIONS_H
void definedFunction();
#endif
main.cpp
:
#include "functions.h"
int main() {
definedFunction();
return 0;
}
In the above code, we have renamed the function from undefinedFunction()
to definedFunction()
. Now, this function can be called from main.cpp
.
These changes ensure that the function called in main.cpp
is both declared and defined, resolving the Id returned 1 exit status
error; thus, the following output indicates that the program executes successfully.
Output:
In these examples and solutions, accompanied by practical examples, emphasize addressing root causes. This approach leads to a smoother development process and helps minimize the occurrence of previous errors.
FAQ: Troubleshooting Id Returned 1 Exit Status
in C++ Projects
Q1: What Steps Can I Take When the Error Persists After Addressing Common Causes?
A1 - If the Id Returned 1 Exit Status
error persists, consider checking for typos, file naming conventions, or hidden characters in our code. Revisit function declarations, file paths, and the overall project structure.
Additionally, review any recent changes or updates that might have introduced new issues.
Q2: How Do I Interpret Specific Error Messages Related to Id Returned 1 Exit Status
?
A2 - Pay close attention to error details provided by the compiler and linker. These messages often indicate the specific source file or function causing the issue.
Analyze the error details to pinpoint the root cause and use them as a guide for debugging and resolving the problem.
Conclusion
In conclusion, the Id returned 1 exit status
error in C++ is a common linker error that occurs during the compilation and linking process, signaling a problem in the final step of creating an executable from compiled source code. This error is often associated with issues such as syntax errors, compiler-library mismatches, incorrect library links, missing header files, duplications of functions or variables across multiple source files, and the absence of a main function.
The article outlines common causes of the error and provides detailed solutions for two specific cases: a mismatch between compiler versions and incorrect or missing header files. Practical examples and code snippets are used to illustrate the solutions, emphasizing the importance of addressing root causes for a smoother development process and reducing the likelihood of recurring errors.
By following the recommended solutions, such as correcting syntax errors, ensuring proper compiler version compatibility, and managing header files appropriately, developers can successfully resolve the Id returned 1 exit status
error, leading to the execution of the program without issues. Overall, the article serves as a comprehensive guide for developers facing this particular error, offering insights and practical solutions to enhance the troubleshooting and debugging process in C++ projects.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook