Bit Array in C++
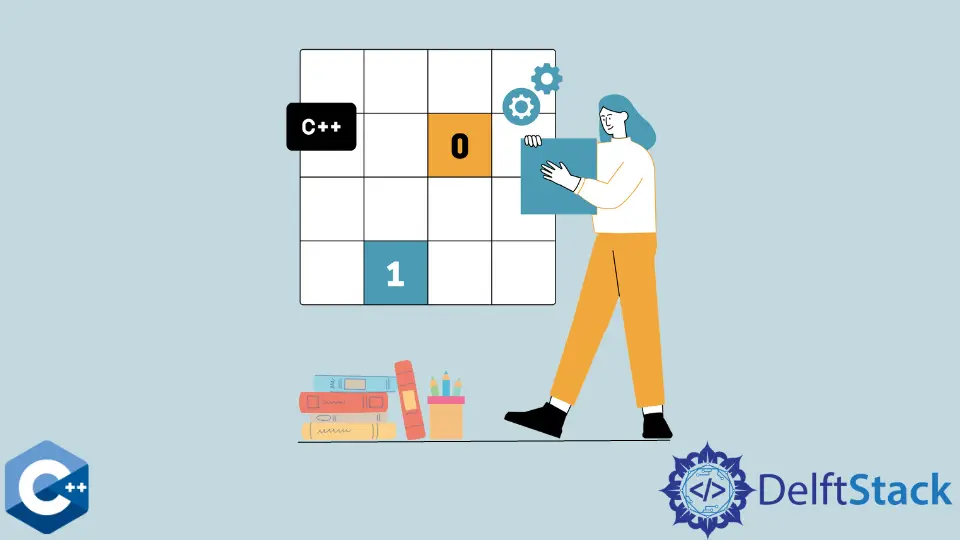
A bit array is an efficient data structure that can store and manipulate a sequence of bits. This post will show how to create, initialize, access, and print the content of a bit array in C++.
Bit arrays are usually initialized with a set of bits in the range 0-1. Bit arrays are similar to other data structures like strings, vectors, and lists because they store sequential data in memory.
They also have different data types, such as unsigned integers or characters, affecting how they are stored in memory and what operations can be done.
Bit arrays are sometimes implemented as an array of pointers to arrays of char
or unsigned char
, where each pointer points to the first element (i.e., bit) of an array, and the size is given by the number of elements in that array.
Uses of Bit Array in C++
Bit arrays are an efficient data structure in C++ which allow programmers to store large amounts of data in a fixed-size array.
The primary use of a bit array is to store Boolean values. Bit arrays can also be used for other purposes, including storing integers, strings, or pointers.
Bit arrays are often used as a compact representation of sets or flags. Since it can hold only one bit per memory location, it is not very efficient for storing large data arrays, as it would take up too much memory.
Steps to Use Bit Array in C++
The steps to use a bit array in C++ are as follows.
-
Define the size of the array in bits.
-
Define the number of elements in the array.
-
Initialize the value of all bits to 0.
-
Set a specific bit to 1 by specifying its index in the array, and then set all other bits to zero by shifting them off by one position.
-
Add a new element at any position by setting that bit and shifting off all other bits.
Example:
#include <bits/stdc++.h>
#include <stdio.h>
using namespace std;
int main() {
bitset<4> bset1(14);
bitset<4> bset2(7);
cout << (bset1 &= bset2) << endl;
cout << (bset1 ^= bset2) << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook