How to Declare an Array of Vectors in C++ STL
- Use C-style Array Notation to Declare an Array of Vectors in C++
-
Use
std::vector
to Declare an Array of Vectors in C++
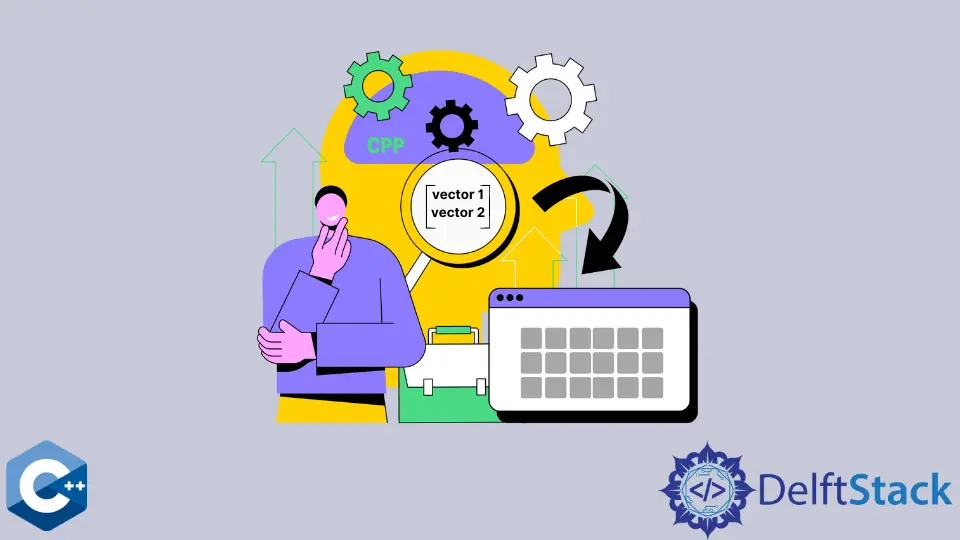
This article will demonstrate multiple methods about how to declare an array of vectors in C++.
Use C-style Array Notation to Declare an Array of Vectors in C++
A fixed array of vectors can be declared by the C-style array brackets notation - []
. This method essentially defines a two-dimensional array with a fixed number of rows and a variable number of columns. The columns can be added with the push_back
function call and the elements accessed by the arr[x][y]
notation if needed. In the following example, we push ten random integer values to each column of the array, which results in a ten by ten matrix.
#include <iomanip>
#include <iostream>
#include <vector>
using std::array;
using std::cout;
using std::endl;
using std::setw;
using std::vector;
int main() {
vector<int> arr_vectors[10];
for (auto &vec : arr_vectors) {
for (int i = 0; i < 10; ++i) {
vec.push_back(rand() % 100);
}
}
for (auto &item : arr_vectors) {
for (auto &i : item) {
cout << setw(3) << i << "; ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Output:
83; 86; 77; 15; 93; 35; 86; 92; 49; 21;
62; 27; 90; 59; 63; 26; 40; 26; 72; 36;
11; 68; 67; 29; 82; 30; 62; 23; 67; 35;
29; 2; 22; 58; 69; 67; 93; 56; 11; 42;
29; 73; 21; 19; 84; 37; 98; 24; 15; 70;
13; 26; 91; 80; 56; 73; 62; 70; 96; 81;
5; 25; 84; 27; 36; 5; 46; 29; 13; 57;
24; 95; 82; 45; 14; 67; 34; 64; 43; 50;
87; 8; 76; 78; 88; 84; 3; 51; 54; 99;
32; 60; 76; 68; 39; 12; 26; 86; 94; 39;
Use std::vector
to Declare an Array of Vectors in C++
Alternatively, one can use the std::vector
container to declare a variable array of vectors. The following code snippet demonstrates the declaration and initialization of the four by four matrix of integers. Note that the constructor’s second argument is another vector
constructor that initializes its elements to zeros. The object’s elements can be accessed using the same arr[x][y]
notation. On the plus side, the row and columns can be extended dynamically using the built-in functions of the std::vector
container.
#include <iomanip>
#include <iostream>
#include <vector>
using std::array;
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int LENGTH = 4;
constexpr int WIDTH = 4;
int main() {
vector<vector<int>> vector_2d(LENGTH, vector<int>(WIDTH, 0));
vector_2d[2][2] = 12;
cout << vector_2d[2][2] << endl;
vector_2d.at(3).at(3) = 99;
cout << vector_2d[3][3] << endl;
cout << endl;
return EXIT_SUCCESS;
}
Output:
12
99
Iteration through the vector of vectors can be done using the two-level nested range
based loops. Notice that the access element alias name is the vector element in the inner for
loop.
#include <iomanip>
#include <iostream>
#include <vector>
using std::array;
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int LENGTH = 4;
constexpr int WIDTH = 4;
int main() {
vector<vector<int>> vector_2d(LENGTH, vector<int>(WIDTH, 0));
std::srand(std::time(nullptr));
for (auto &item : vector_2d) {
for (auto &i : item) {
i = rand() % 100;
cout << setw(2) << i << "; ";
}
cout << endl;
}
cout << endl;
for (auto &item : vector_2d) {
for (auto &i : item) {
i *= 3;
}
}
for (auto &item : vector_2d) {
for (auto &i : item) {
cout << setw(3) << i << "; ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Output:
62; 85; 69; 73;
22; 55; 79; 89;
26; 89; 44; 66;
32; 40; 64; 32;
186; 255; 207; 219;
66; 165; 237; 267;
78; 267; 132; 198;
96; 120; 192; 96;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook