How to Initialize Array of Objects in C++
-
Use the
new
Operator to Initialize Array of Objects With Parameterized Constructors in C++ -
Use the
std::vector::push_back
Function to Initialize Array of Objects With Parameterized Constructors -
Use the
std::vector::emplace_back
Function to Initialize Array of Objects With Parameterized Constructors
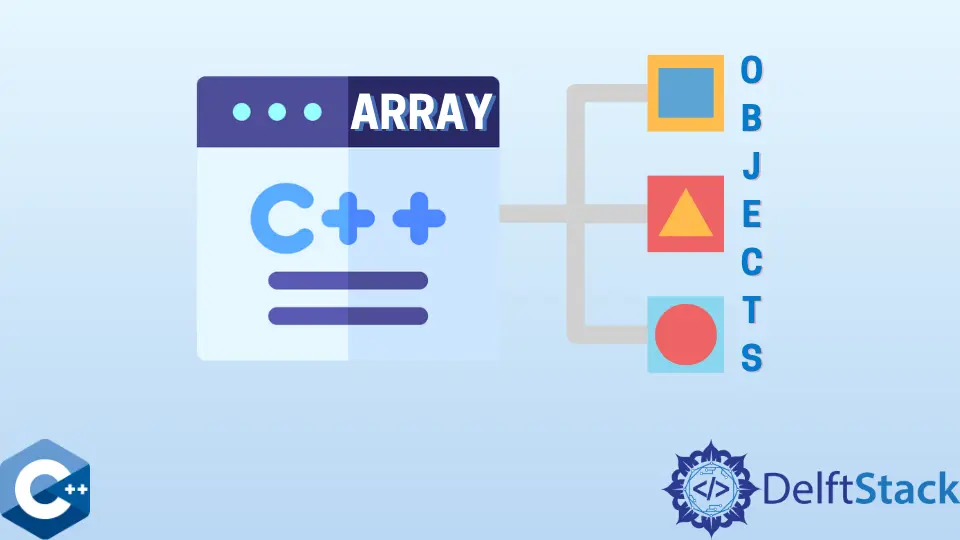
This article will demonstrate multiple methods about initializing an array of objects with parameterized constructors in C++.
Use the new
Operator to Initialize Array of Objects With Parameterized Constructors in C++
The new
operator is part of the C++ dynamic memory management interface, and it is equivalent to the malloc
function from the C language. Note that dynamic memory management entails the flow in which the user allocates the memory of a specified fixed size and returns it to the system when it is not needed anymore.
new
is used to request the fixed number of bytes from the system, which returns the pointer to the memory region if the call is successful. In the following example, we defined a class named Pair
with two constructors and printPair
to output the data members to the console. At first, we need to allocate a four-member array of Pairs
using the new
operator. Next, we can traverse the array with the for
loop, and each iteration initialize the element with the parameterized constructor. Mind though, that the allocated array should be freed before the program exit using the delete
operator. Notice also the delete []
notation is necessary to deallocate each element of the array.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
Pair *arr = new Pair[4];
for (int i = 0; i < 4; ++i) {
arr[i] = Pair(i * 2, i * i);
}
for (int i = 0; i < 4; ++i) {
arr[i].printPair();
}
delete[] arr;
return EXIT_SUCCESS;
}
Output:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
Use the std::vector::push_back
Function to Initialize Array of Objects With Parameterized Constructors
Alternatively, a more headless approach would be to store the objects in the std::vector
container, which would provide the built-in function to initialize new elements dynamically. Namely, the Pair
definition stays the same, but each iteration, we will call the push_back
function and pass it the Pair
value from the second constructor. On the plus side, we don’t need to worry about memory resources’ deallocation; they get cleaned up automatically.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
vector<Pair> array;
for (int i = 0; i < 4; ++i) {
array.push_back(Pair(i * 2, i * i));
}
for (const auto &item : array) {
item.printPair();
}
return EXIT_SUCCESS;
}
Output:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
Use the std::vector::emplace_back
Function to Initialize Array of Objects With Parameterized Constructors
Another method to initialize an array of objects with parameterized constructors is to utilize the emplace_back
function of the std::vector
class. In this way, we only need to pass the Pair
constructor’s values, and emplace_back
would automatically construct a new element for us. Like the previous method, this one also does not need the user to worry about memory management.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
class Pair {
int x, y;
public:
Pair() = default;
Pair(int a, int b) : x(a), y(b) {}
void printPair() const { cout << "x: " << x << endl << "y: " << y << endl; }
};
int main() {
vector<Pair> array;
for (int i = 0; i < 4; ++i) {
array.emplace_back(i * 2, i * i);
}
for (const auto &item : array) {
item.printPair();
}
return EXIT_SUCCESS;
}
Output:
x: 0
y: 0
x: 2
y: 1
x: 4
y: 4
x: 6
y: 9
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook