How to Use strtok Function in C
- Understanding strtok Function
- Basic Example of Using strtok
- Handling Multiple Delimiters
- Important Considerations
- Conclusion
- FAQ
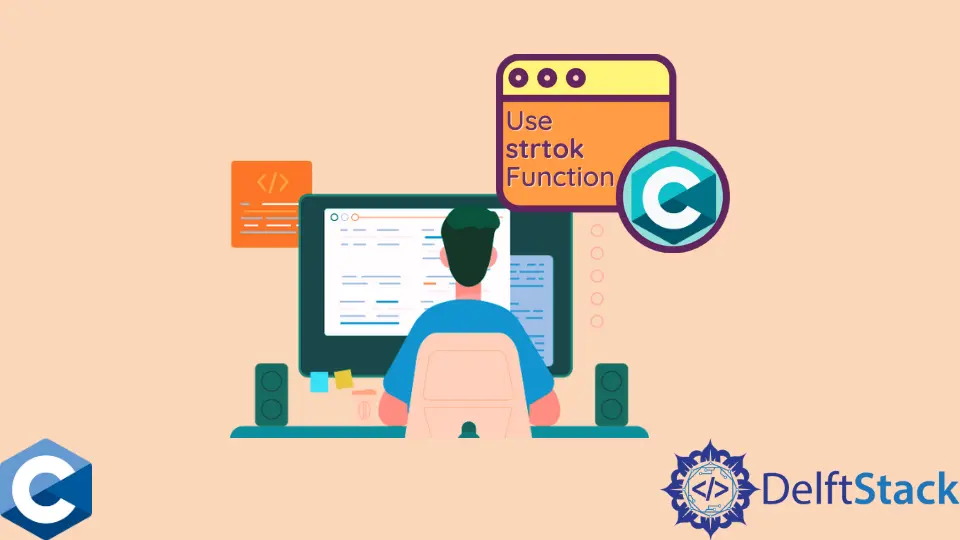
When it comes to string manipulation in C, one of the most essential functions you’ll encounter is strtok
. This function is particularly useful for splitting strings into tokens based on specified delimiters. Whether you’re parsing user input, reading data from files, or processing strings in general, mastering strtok
can significantly enhance your programming skills.
In this article, we will explore how to effectively use the strtok
function in C, providing you with practical examples and a clear understanding of its functionality. By the end, you’ll be equipped to handle string tokenization like a pro.
Understanding strtok Function
The strtok
function is part of the C standard library and is included in the <string.h>
header file. Its primary purpose is to tokenize a string, meaning it breaks down a string into smaller parts, or tokens, based on specified delimiters. The syntax for strtok
is as follows:
char *strtok(char *str, const char *delim);
- str: The string to be tokenized. On the first call, this should point to the string you want to tokenize. On subsequent calls, this should be
NULL
. - delim: A string containing all the delimiter characters.
When you call strtok
, it returns a pointer to the first token found in the string. Subsequent calls to strtok
with the same string will return the next token until there are no more tokens left, at which point it returns NULL
.
Basic Example of Using strtok
To demonstrate how to use strtok
, let’s look at a simple example where we split a comma-separated list of fruits.
#include <stdio.h>
#include <string.h>
int main() {
char fruits[] = "apple,banana,cherry,dates";
char *token;
token = strtok(fruits, ",");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
return 0;
}
Output:
apple
banana
cherry
dates
In this example, we start by defining a string fruits
containing several fruit names separated by commas. We call strtok
with fruits
and the delimiter ","
to extract the first token. We then enter a loop where we continue to call strtok
with NULL
to get subsequent tokens. Each token is printed until there are no more tokens left.
Handling Multiple Delimiters
One of the powerful features of strtok
is its ability to handle multiple delimiters. For example, if you want to tokenize a string that contains spaces, commas, and semicolons as delimiters, you can easily achieve this.
#include <stdio.h>
#include <string.h>
int main() {
char data[] = "apple;banana, cherry orange;grape";
char *token;
token = strtok(data, " ,;");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, " ,;");
}
return 0;
}
Output:
apple
banana
cherry
orange
grape
In this example, the string data
contains fruit names separated by spaces, commas, and semicolons. When we call strtok
, we specify a string of delimiters " ,;"
. Each token is extracted and printed in the loop, demonstrating how strtok
can effectively handle multiple delimiters.
Important Considerations
While strtok
is a powerful tool for tokenization, there are some important considerations to keep in mind when using it. First, strtok
modifies the original string by inserting null characters ('\0'
) at the delimiter positions. This means that if you need to preserve the original string, you should make a copy before tokenizing.
Additionally, strtok
is not thread-safe because it uses a static variable to keep track of the current position in the string. If you need to tokenize strings in a multi-threaded environment, consider using strtok_r
, which is a reentrant version of strtok
.
Here’s an example of preserving the original string:
#include <stdio.h>
#include <string.h>
int main() {
char original[] = "apple,banana,cherry";
char copy[50];
strcpy(copy, original);
char *token;
token = strtok(copy, ",");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
printf("Original string: %s\n", original);
return 0;
}
Output:
apple
banana
cherry
Original string: apple,banana,cherry
In this example, we create a copy of the original string before tokenizing it. This way, we can still access the original string after the tokenization process.
Conclusion
The strtok
function is a fundamental tool for string manipulation in C, allowing you to split strings into manageable tokens based on specified delimiters. By understanding its functionality and limitations, you can effectively use strtok
in your programs. Whether you’re parsing data, handling user input, or processing strings in general, mastering strtok
will enhance your coding skills and make your programs more efficient.
FAQ
-
What is the purpose of the strtok function?
Thestrtok
function is used to tokenize a string into smaller segments based on specified delimiters. -
Can strtok handle multiple delimiters?
Yes,strtok
can handle multiple delimiters by passing a string of delimiter characters. -
Does strtok modify the original string?
Yes,strtok
modifies the original string by replacing delimiters with null characters. -
Is strtok thread-safe?
No,strtok
is not thread-safe. For thread-safe tokenization, usestrtok_r
. -
How can I preserve the original string while using strtok?
You can create a copy of the original string before usingstrtok
to preserve it.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook