How to Check if String Contains Substring in C
-
Method 1: Using the
strstr
Function - Method 2: Manual Search Using Loops
-
Method 3: Using the
strpbrk
Function - Conclusion
- FAQ
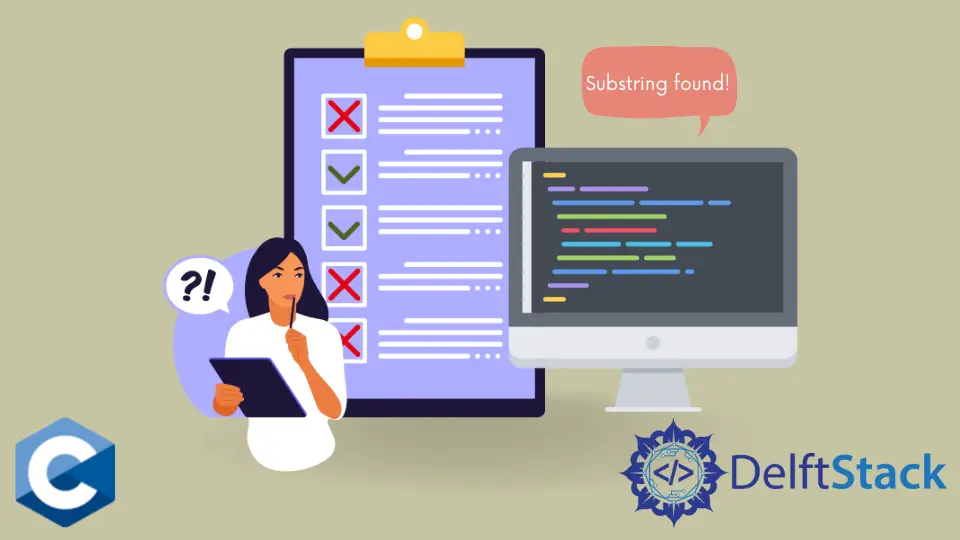
In C programming, working with strings is a fundamental skill that every developer must master. One common task is checking if a string contains a specific substring. This operation is crucial in various applications, such as text processing, data validation, and user input handling.
In this article, we will explore different methods to achieve this goal in C. Whether you’re a beginner or a seasoned programmer, understanding how to manipulate strings effectively can enhance your coding capabilities. So, let’s dive into the methods that will help you determine if a string contains a substring in C.
Method 1: Using the strstr
Function
One of the most straightforward ways to check if a string contains a substring in C is by using the strstr
function. This function is part of the C standard library and is included in the <string.h>
header file. The strstr
function searches for the first occurrence of a substring within a string and returns a pointer to the beginning of the substring if found. If not, it returns NULL
.
Here’s a simple example:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, welcome to the world of C programming.";
char substr[] = "welcome";
if (strstr(str, substr) != NULL) {
printf("Substring found!\n");
} else {
printf("Substring not found.\n");
}
return 0;
}
Output:
Substring found!
In this example, we define a main function that initializes a string and a substring. The strstr
function is called to check if substr
exists within str
. If the function returns a non-NULL pointer, it indicates that the substring is present, and we print a corresponding message. If it returns NULL, we notify the user that the substring is absent. This method is efficient and easy to implement, making it a popular choice among C programmers.
Method 2: Manual Search Using Loops
While the strstr
function is convenient, sometimes you may want to implement your own logic for educational purposes or specific requirements. A manual search using loops can achieve this. In this method, we iterate through the main string and check for matches character by character.
Here’s how you can do it:
#include <stdio.h>
int containsSubstring(char *str, char *substr) {
int strLen = 0, substrLen = 0;
while (str[strLen] != '\0') strLen++;
while (substr[substrLen] != '\0') substrLen++;
for (int i = 0; i <= strLen - substrLen; i++) {
int j;
for (j = 0; j < substrLen; j++) {
if (str[i + j] != substr[j]) break;
}
if (j == substrLen) return 1; // Found
}
return 0; // Not found
}
int main() {
char str[] = "Hello, welcome to the world of C programming.";
char substr[] = "world";
if (containsSubstring(str, substr)) {
printf("Substring found!\n");
} else {
printf("Substring not found.\n");
}
return 0;
}
Output:
Substring found!
In this example, we define a function containsSubstring
that takes two strings as parameters. It first calculates the lengths of both strings. The outer loop iterates through the main string, while the inner loop checks for matching characters. If all characters match, we return 1, indicating that the substring was found. If no match is found after all iterations, we return 0. This method gives you a deeper understanding of string manipulation in C and can be customized for various needs.
Method 3: Using the strpbrk
Function
Another useful function from the C standard library is strpbrk
. While it does not directly check for a substring, it can be employed creatively to determine if any character from the substring exists in the main string. This can be useful in scenarios where you want to check for the presence of any character from a set.
Here’s an implementation:
#include <stdio.h>
#include <string.h>
int containsAnyChar(char *str, char *substr) {
return strpbrk(str, substr) != NULL;
}
int main() {
char str[] = "Hello, welcome to the world of C programming.";
char substr[] = "xyz";
if (containsAnyChar(str, substr)) {
printf("At least one character from the substring found!\n");
} else {
printf("No characters from the substring found.\n");
}
return 0;
}
Output:
No characters from the substring found.
In this code, the containsAnyChar
function uses strpbrk
to check if any character from substr
is found in str
. If it finds a match, it returns a non-NULL pointer; otherwise, it returns NULL. The main function tests this with a string and a substring. This method is particularly useful when you want to check for multiple characters rather than an exact substring match.
Conclusion
Checking if a string contains a substring in C can be accomplished through various methods, each with its advantages. Whether you choose to use built-in functions like strstr
and strpbrk
or implement a manual search using loops, understanding these techniques will enhance your programming skills. As you continue to work with strings, remember that practice is key. Experiment with these methods, and soon you’ll feel confident in handling string manipulations in C.
FAQ
-
Can I check for case-insensitive substring matches in C?
Yes, you can convert both strings to lowercase or uppercase before performing the substring check. -
Is there a built-in function in C for case-insensitive substring search?
The C standard library does not provide a direct function for case-insensitive searches, but you can implement one usingstricmp
orstrcasecmp
. -
What if the substring is longer than the main string?
If the substring is longer than the main string, it cannot be found, and the search should return false. -
How can I find all occurrences of a substring in a string?
You can modify the loop logic to continue searching after finding a match, storing the positions of all occurrences. -
Are there any performance considerations when checking for substrings?
Yes, the complexity of the search method can affect performance, especially with larger strings. Using efficient algorithms can help improve speed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook